In this post, we will see what Swagger is and how to install it in the Web API project created in the post: ASP.NET Core – Web API.
First of all, what is Swagger?
From the official web site:
Swagger allows you to describe the structure of your APIs so that machines can read them. The ability of APIs to describe their own structure is the root of all awesomeness in Swagger.
For every information, go to the Swagger official web site: https://swagger.io
Before installing Swagger, we will add all CRUD operations in our Web API:
GET
[HttpGet("{id}")]
public async Task<ActionResult<UserVM>> GetUserItem(Int64 Id)
{
UserVM objUser = null;
objUser = await _contextProject.Users.Include(ll => ll.UserType).Where(ll=>ll.Id == Id).Select(ll =>
new UserVM()
{
Id = ll.Id,
Username = ll.Username,
CreateDate = ll.CreateDate.ToShortDateString(),
TypeUserName = ll.UserType.Name
}).FirstOrDefaultAsync();
if(objUser == null)
{
// Return code 404
return NotFound();
}
return objUser;
}
DELETE
[HttpDelete("{id}")]
public async Task<ActionResult> DeleteUserItem(Int64 Id)
{
var objUser = await _contextProject.Users.FindAsync(Id);
if (objUser == null)
{
// Return code 404
return NotFound();
}
_contextProject.Users.Remove(objUser);
await _contextProject.SaveChangesAsync();
// Return code 204
return NoContent();
}
CREATE
[HttpPost]
public async Task<ActionResult> CreateUserItem([FromBody] UserVM inputUser)
{
var IdTypeUser = await _contextProject.UserTypes.Where(ll=>ll.Name == inputUser.TypeUserName).FirstOrDefaultAsync();
User objUser = new User { CreateDate = DateTime.Now, TypeUserId = IdTypeUser.TypeUserId, Username = inputUser.Username };
_contextProject.Users.Add(objUser);
await _contextProject.SaveChangesAsync();
// Return code
return Ok();
}
UPDATE
[HttpPut]
public async Task<ActionResult> UpdateUserItem([FromBody] UserVM inputUser)
{
var objUserDb = await _contextProject.Users.Where(ll => ll.Id == inputUser.Id).FirstOrDefaultAsync();
if(objUserDb==null)
{
return NotFound();
}
var IdTypeUser = await _contextProject.UserTypes.Where(ll => ll.Name == inputUser.TypeUserName).FirstOrDefaultAsync();
objUserDb.Username = inputUser.Username;
objUserDb.TypeUserId = IdTypeUser.TypeUserId;
_contextProject.Entry(objUserDb).State = EntityState.Modified;
await _contextProject.SaveChangesAsync();
// Return code 204
return NoContent();
}
Now, we will install the Swashbuckle.AspNetCore package, using the command
Install-Package Swashbuckle.AspNetCore -Version 4.0.1 in the Package Manager Console.
After the installation, we have to configure the Swagger middleware into Startup.cs file.
Let’s start to register Swagger in the ConfigureServices method:
public void ConfigureServices(IServiceCollection services)
{
services.AddDbContext<ProjectContext>(opt => opt.UseInMemoryDatabase("ProjectDB"));
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_2);
services.AddSwaggerGen(c => {
c.SwaggerDoc("v1", new Info { Title = "User API", Version = "v1" });
});
}
Then, we have to enable the middleware for serving the json document and the Swagger UI:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseHsts();
}
app.UseSwagger();
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "User API");
});
app.UseHttpsRedirection();
app.UseMvc();
}
Finally, we have to add swagger as launchUrl in IIS Express, modifying the file launchSettings.json:
"profiles": {
"IIS Express": {
"commandName": "IISExpress",
"launchBrowser": true,
"launchUrl": "swagger",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
},
The installation is done and now, if we run the Web API, this will be the result:

DESCRIPTION OF THE OUTPUT

TESTING THE METHOD FROM SWAGGER UI
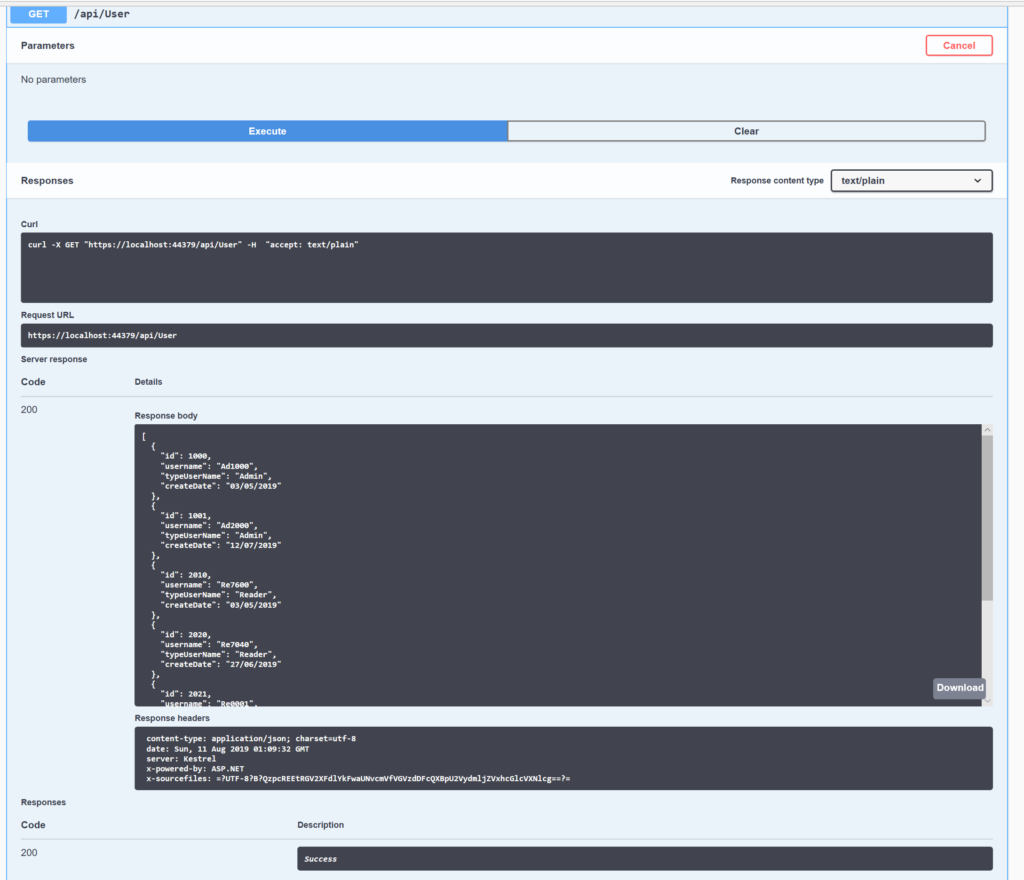