In this post, we will create an Angular application that it will show a list of users taken from the Web API created in the post: Web API with ASP.NET Core.
We open Terminal and create a new project called “manageusers”:
ng new manageusers
Now, we will create a component called listeners for showing the list of users.
We go into project directory and we run the command:
ng g c listusers

Now, we will create the class User:
ng g class user
Finally, we create the “service” called webapiuser to manage the calls from the Web API service:
ng g service webapiuser
Now, we will define all components created until now:
[USER.JS]
export class User
{
id: number;
username: string;
typeUserName: string;
createDate: string;
}
[WEBAPIUSER.JS]
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { Observable } from 'rxjs';
import { User } from './user';
@Injectable({
providedIn: 'root'
})
export class webapiuser {
// Web Api service url
webapiUserUrl = 'https://firstwebapi001.azurewebsites.net/api';
constructor(private http: HttpClient) { }
// define a method getListUsers for calling the user method of Web Api service
getListUsers(): Observable<Array<User>> {
return this.http.get<Array<User>>(this.webapiUserUrl + '/user');
}
}
[LISTUSERS.JS]
import { Component, OnInit } from '@angular/core';
import { User } from '../user';
import { webapiuser } from '../webapiuser.service';
@Component({
selector: 'app-listusers',
templateUrl: './listusers.component.html',
styleUrls: ['./listusers.component.css']
})
export class ListusersComponent implements OnInit {
// define an Array of User
lstUsers: Array<User>;
// Use the dependency injection to use the service in this component
constructor(private serviceuser: webapiuser) { }
ngOnInit() {
// call the service to take the list of users
this.serviceuser.getListUsers().subscribe(data => {
this.lstUsers = data;
});
}
}
[LISTUSERS.COMPONENT.HTML]
<p>List of Users</p>
<table>
<tr>
<td>ID</td>
<td>Username</td>
<td>TypeUser</td>
<td>Create Data</td>
</tr>
<tbody *ngFor="let item of lstUsers">
<tr>
<td>{{item.id}}</td>
<td>{{item.username}}</td>
<td>{{item.typeUserName}}</td>
<td>{{item.createDate}}</td>
</tr>
</tbody>
</table>
In app.component.html, we delete everything and leave only:
<router-outlet>
</router-outlet>
Now, we modify the file app-routing.module.ts:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { ListusersComponent } from './listusers/listusers.component';
const routes: Routes = [
{ path: '', pathMatch: 'full', redirectTo: 'listusers'},
{ path: 'listusers', component: ListusersComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Finally, we verify that the file app.module.ts is like that:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { ListusersComponent } from './listusers/listusers.component';
@NgModule({
declarations: [
AppComponent,
ListusersComponent
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Now, we run the application using the command ng serve, open a browser and we go to: http://localhost:4200

We can see that the application doesn’t work.
This because, I forgot to set up the CORS in my Web Api.
Now, we can fix it either via code or using the configuration in Azure and, for this post, we will use the second choice.
We go on Azure portal, select the web app, we click CORS in API and we set up the Allowed Origins.
In this case, I will insert localhost:4200.
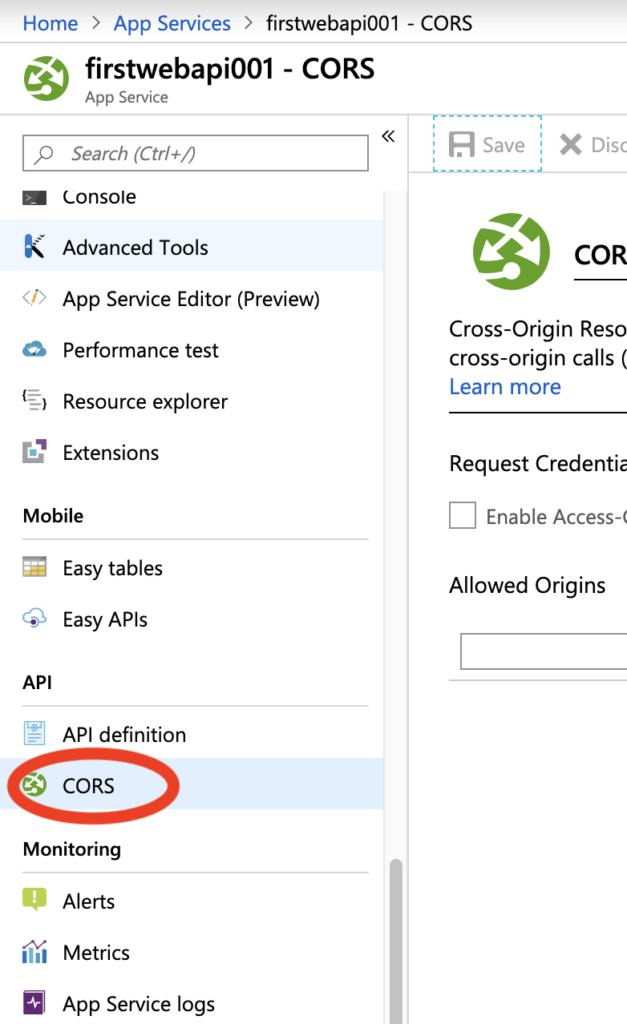
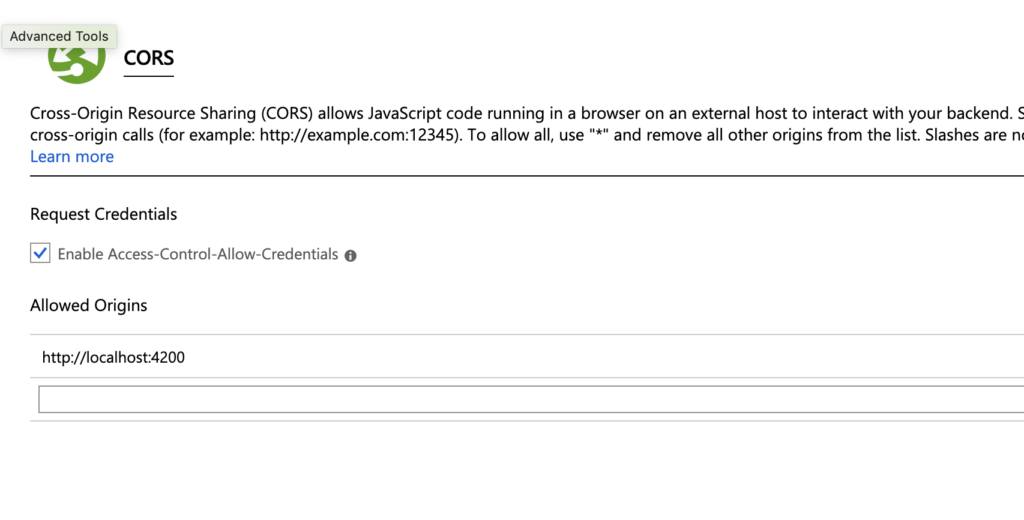
Now, if we try to run the application again, this will be the result:
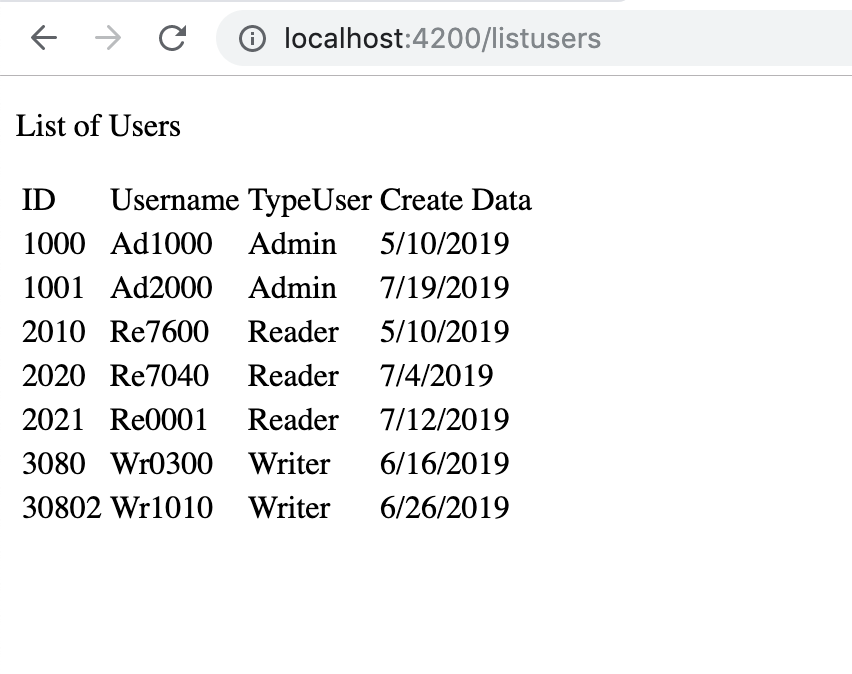