Set is an unordered collection of comma separated unique elements inside curly braces.
The order of element is undefined and it is possible to add and delete elements and, it is possible to perform standard operations on sets like union, intersection and difference.
In this post, we will see how to manage Set in Python.
CREATE
# create an empty Set
print(" CREATE")
varSet1 = set()
print(f"varSet1: {varSet1}")
# create set
varSet2 = {1,2,"three"}
varSet3 = {2,"four"}
print(f"varSet2: {varSet2}")
print(f"varSet3: {varSet3}")
# create set from an array
lst1 = [1,2,3,4,5]
print(f"lst1: {lst1}")
varSet4 = set(lst1)
print(f"varSet4: {varSet4}")
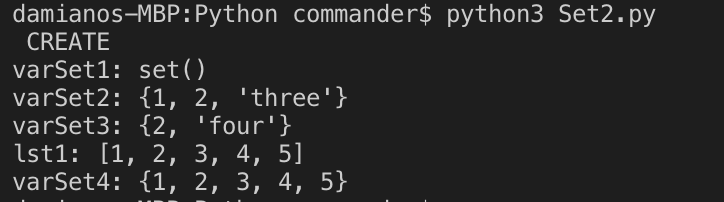
ADD
# add
print(" ADD ")
print(f"Initial value of varSet1: {varSet1}")
varSet1.add(1)
varSet1.add("two")
print(f"Final value of varSet1: {varSet1}")
# add multiple values
varSet1.update([5,6,"ten"])
print(f"New value of varSet1: {varSet1}")
print(f"Initial value of varSet2: {varSet2}")
# value will not be inserted because it's already in the Set
varSet2.add(1)
varSet2.add("two")
varSet2.add("five")
varSet2.add("9")
print(f"Final value of varSet2: {varSet2}")
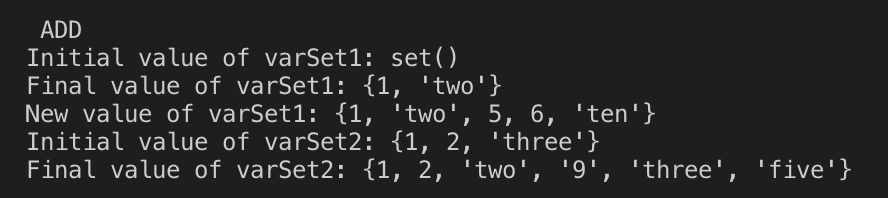
REMOVE
# remove
print(" REMOVE ")
print(f"Initial value of varSet2: {varSet2}")
varSet2.remove(2)
varSet2.remove('three')
print(f"Final value of varSet2: {varSet2}")
# delete all items in the Set
varSet2.clear()
print(f"Real final value of varSet2: {varSet2}")
# delete the set completely
del varSet2

READ
# read
print(f"varSet1: {varSet1}")
for i in varSet1:
print(i)
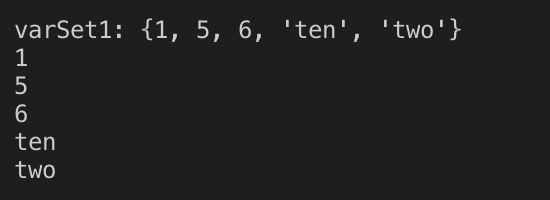
COPY A SET
set1 = {1,2,3,4}
set2 = set1
set3 = set1.copy()
print(f"Set1 -> {set1}")
print(f"Set2 -> {set2}")
print(f"Set3 -> {set3}")
set1.remove(3)
print("***************")
print(f"Set1 -> {set1}")
print(f"Set2 -> {set2}")
print(f"Set3 -> {set3}")
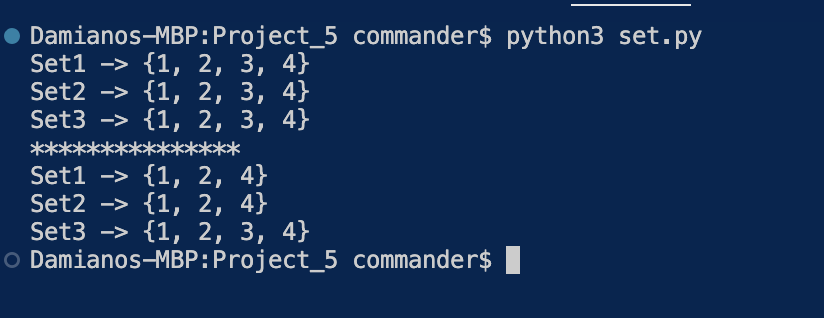
FROZEN A SET
# using frozenset, it will be impossible to change a Set
set1 = frozenset({1,2,3,4})
set1.add(7)
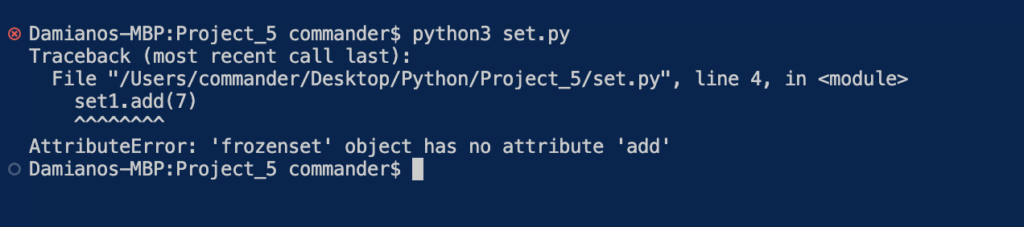
OPERATIONS
# some operations
A = {1,2,3,4}
B = {1,4,6,7}
print(f"A: {A}")
print(f"B: {B}")
# Difference: take items that are in A but not in B
print(f"A-B: {A-B}")
# Union: take items that are in A and in B
print(f"A|B: {A|B}")
# Simmetric Difference: take items that are in A but not in B and
# items that are in B and not in A
print(f"A^B: {A^B}")
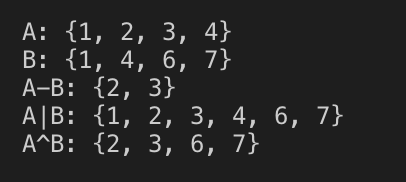