In this post, we will see how to declare and use variables, constants and functions in Go.
VARIABLES
We open Visual Studio Code, go the directory users\go and into the folder src we create a directory called Test1.
Here, we create a file called main.go:
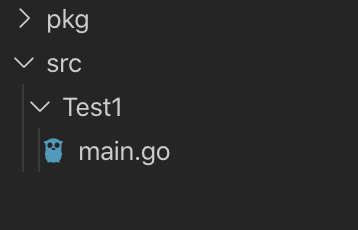
package main
import (
"fmt"
)
func main() {
// define the variable val1
var val1 int = 4
// define a list of varibles val2 val3
var (
val2 string = "One"
val3 bool = false
)
// define variable val4
val4 := 5
// define variable val5
var val5 float32 = 15.78
// print the values of variables
fmt.Printf("The value of val1 is: %d \n", val1)
fmt.Printf("The value of the variable val2 is: %s, instead the value of variable val3 is: %t \n", val2, val3)
fmt.Printf("The value of val4 is: %d \n", val4)
fmt.Printf("The value of val4 is: %f \n", val5)
}
For running the application, we could use the command go run main.go but, with the command go build, we will create an executable program:
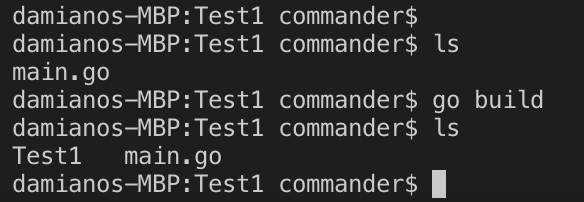
In macOS, we can run the executable file using the command ./Test1:

CONSTANTS
In order to see how to work with constants, we take the main.go file and we do these changes:
package main
import (
"fmt"
)
func main() {
printConst()
}
func printConst() {
const const1 int = 4
const (
const2 string = "Hello World"
const3 bool = true
)
fmt.Printf("The value of const1 is: %d \n", const1)
fmt.Printf("The value of const2 is: %s \n", const2)
fmt.Printf("The value of const3 is: %t \n", const3)
}
If we run the program, this will be the result:
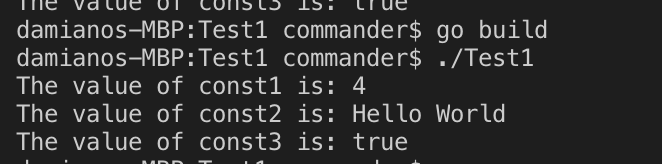
FUNCTIONS
In order to see how to create different types of function, we take the main.go file and we do these changes:
package main
import (
"fmt"
"time"
)
func main() {
test1()
test2("Hello world")
fmt.Printf("The current year is : %d \n", test3())
fmt.Printf("Ten squared is : %d \n", test4(10))
}
// function without parameters in input and output
func test1() {
fmt.Printf("Function without parameters \n")
}
// function with parameters in input and without parameters in output
func test2(val1 string) {
fmt.Printf("Function with parameter in input: %s \n", val1)
}
// function without parameters in input and with parameters in output
func test3() int {
return time.Now().Year()
}
// function with parameters in input and with parameters in output
func test4(val1 int) int {
return val1 * val1
}
If we run the program, this will be the result:
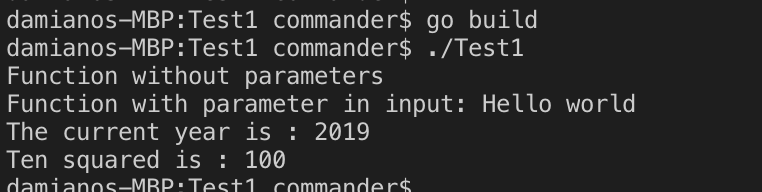