In this post, we will see how to create a RESTful service for handling data of some Dogs, with Python and Flask.
First of all, what Flask is?
From Wikipedia:
“Flask is a micro web framework written in Python. It is classified as a microframework because it does not require particular tools or libraries.
It has no database abstraction layer, form validation, or any other components where pre-existing third-party libraries provide common functions. However, Flask supports extensions that can add application features as if they were implemented in Flask itself. Extensions exist for object-relational mappers, form validation, upload handling, various open authentication technologies and several common framework related tools. Extensions are updated far more frequently than the core Flask program.“
We open Visual Studio Code and we create two files called EntityDog and Core.py:
[ENTITYDOG.PY]
class Dog:
def __init__(self, id, breed, color, age):
self.Id = id
self.Breed = breed
self.Color = color
self.Age = age
[CORE.PY]
# import the class Dog
from EntityDog import Dog
# This class will be our Business Layer
class Core:
def __init__(self):
# we define a public property called lstDogs
self.lstDogs = self.loadDog()
# we define a function in order to feed the lstDogs property
def loadDog(self):
lstDog = []
dog1 = Dog(1,'Alano', 'Black and White', 2)
dog2 = Dog(2,'Doberman', 'Black', 1)
dog3 = Dog(3,'Chihuahua', 'Black', 3)
dog4 = Dog(4,'Maltese', 'White', 4)
dog5 = Dog(5,'Rottweiler', 'Black', 7)
lstDog.append(dog1)
lstDog.append(dog2)
lstDog.append(dog3)
lstDog.append(dog4)
lstDog.append(dog5)
return lstDog;
# we define a function to get a dog by the ID
def GetDogById(self, id):
for item in self.lstDogs:
if item.Id == id:
return item
# we define a function to add a new dog
def AddNewDog(self,id, breed,color, age):
objDog = Dog(id,breed, color, age)
self.lstDogs.append(objDog)
Now, we create a folder called template, and inside we will add a file html called index:
[INDEX.HTML]
<!DOCTYPE html>
<html lang='en'>
<head>
<meta charset="utf-8" />
<title>Python</title>
</head>
<body>
<h1>This is a RestFul Service</h1>
</body>
</html>
Then, we open a new Terminal for installing Flask and jsonpickle (this is a Python library for serialization and deserialization of complex Python objects to and from JSON), using the commands:
pip3 install Flask
pip3 install jsonpickle
Finally, we create a file called ServiceRestFul.py:
[SERVICERESTFUL.PY]
from EntityDog import Dog
from Core import Core
import flask
import jsonpickle
# creation of a web server instance
service = flask.Flask(__name__)
# we define our Business layer
objCore = Core()
# we define the method ListDogs, linked with the route '/dogs'
@service.route("/dogs", methods=['GET'])
def ListDogs():
# we use jsonpickle in order to serialize our dogs' list in json
return (jsonpickle.encode(objCore.lstDogs))
# we define the method GetDog, linked with the route '/dog/ (id dog)'
@service.route("/dog/<id>", methods=['GET'])
def GetDog(id):
objDog = objCore.GetDogById(int(id))
if(objDog is None):
# in case of no data, we will show an error
return flask.make_response(flask.jsonify({'error': 'Not found'}), 404)
else:
return jsonpickle.encode(objDog)
# we define the method AddDog, linked with the route '/dog'
@service.route("/dog",methods=['POST'])
def AddDog():
objCore.AddNewDog(flask.request.json['id'], flask.request.json['breed'],flask.request.json['color'], flask.request.json['age'])
return flask.make_response(flask.jsonify({'Response': 'A new dog has been added successfully'}), 201)
# we define the method Home, linked with the route '/'
@service.route("/")
def Home():
# we show a file html
return flask.render_template("index.html")
# start the web server
if __name__ == "__main__":
# run the service
service.run()
Now, if we run the service with the command python3 ServiceRestFul.py, we will have this output:

To verify that everything works fine, we open a browser and go to http://127.0.0.1:5000
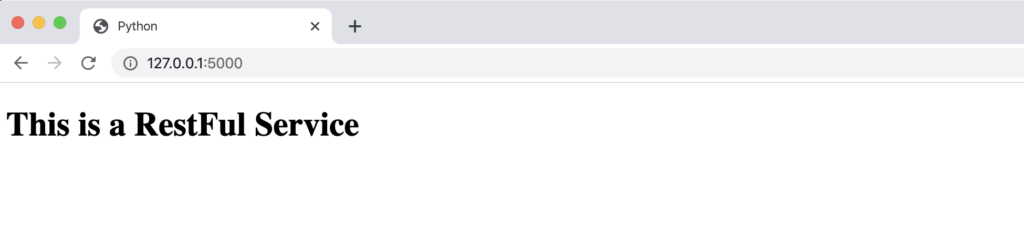
LIST OF DOGS:

GET DOG:
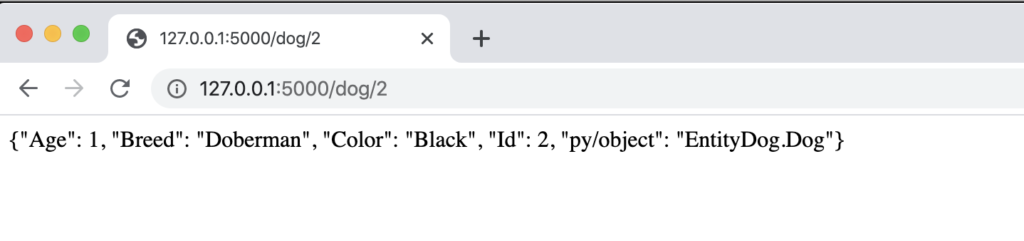
DOG NOT FOUND:

ADD A DOG (using Postman):

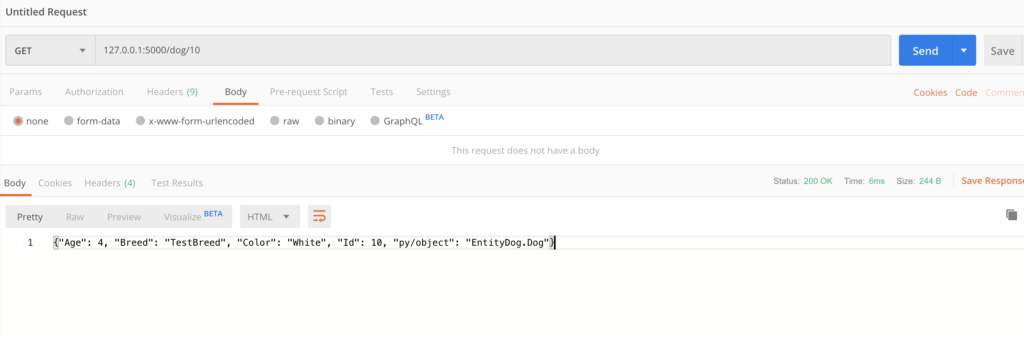