In this post, we will see how to add and use Bootstrap in a React project.
We open the project used in the Post React – How to create a menu and we run the command npm install –save bootstrap, in order to add the Bootstrap framework.
Now, we open the file index.js and we add the file bootstrap.min.css:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
ReactDOM.render(<App />, document.getElementById('root'));
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: https://bit.ly/CRA-PWA
serviceWorker.unregister();
Finally, we will modify the component Menu, in order to add bootstrap:
[MENU.JS]
import React from 'react'
// import from react-router-dom these three object
// used to manage the route
import {
Route,
NavLink,
HashRouter
} from "react-router-dom";
// import the page
import Home from "./Home";
import ListUsers from "./ListUsers";
import NewUser from "./NewUser";
// With <HashRouter> we define the Routing area
// With <NavLink> we define the navigation links
// In the Div "content", we will show all the pages
// The attributes "to" and "path" are used to create a link between NavLink and Route
class Menu extends React.Component {
render() {
return (
<HashRouter>
<nav class="navbar navbar-dark bg-dark mb-5">
<span class="navbar-brand">[Manage Users]</span>
<div class="navbar-expand mr-auto">
<ul class="nav navbar-nav" routerLinkActive="active">
<li class="nav-link"><NavLink to="/">Home</NavLink></li>
<li class="nav-link"><NavLink to="/ListUsers">List Users</NavLink></li>
<li class="nav-link"><NavLink to="/NewUser">New User</NavLink></li>
</ul>
</div>
</nav>
<div>
<h1>Manage Users</h1>
<div className="content">
<Route exact path="/" component={Home}/>
<Route path="/ListUsers" component={ListUsers}/>
<Route path="/NewUser" component={NewUser}/>
</div>
</div>
</HashRouter>
);
}
}
export default Menu;
Now, if we run the application, this will be the result:
HOME:
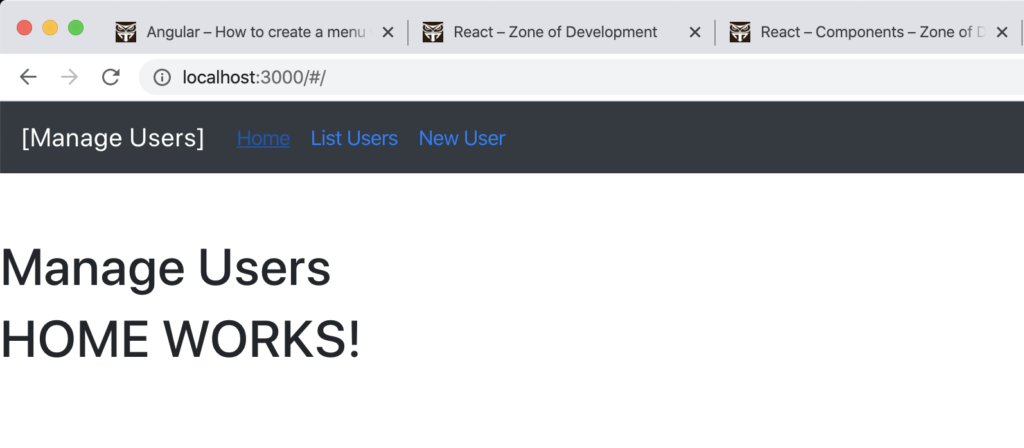
LIST USERS:
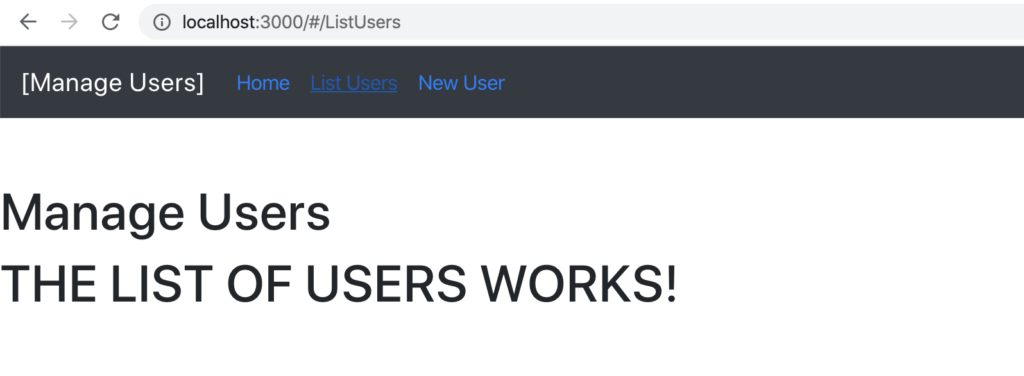
CREATE USER:
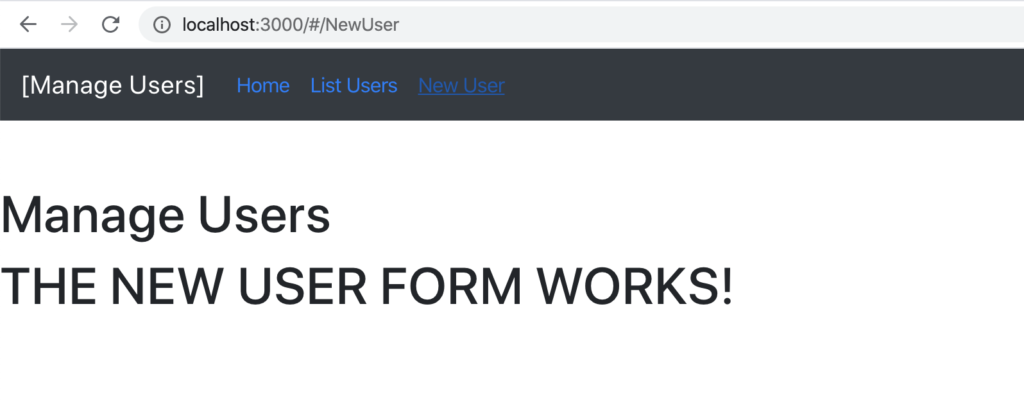