In this post, we will see what Eval() is and how we can use it.
We start creating a function that returns true if a given inequality expression is correct and false otherwise.
Examples:
test_signs(“3 < 7 <11”) return True
test_signs(“13 > 44 > 33 > 1”) return False
test_signs(“1 < 2 < 6 < 9 > 3”) return True
We open Visual Studio code, create a file called CorrectInequalitySigns and we write this code:
[CORRECTINEQUALITYSIGNS.PY]
def test_signs(txt):
arrayText = txt.split(' ')
for i in range(0,len(arrayText)-2, 2):
if(arrayText[i+1]=='>'):
if(int(arrayText[i]) <= int(arrayText[i+2])):
return False
else:
if(int(arrayText[i]) >= int(arrayText[i+2])):
return False
return True
valInput = input("Insert the sequence to check: ")
print(test_signs(valInput))
Now, if we run the code, this will be the result:
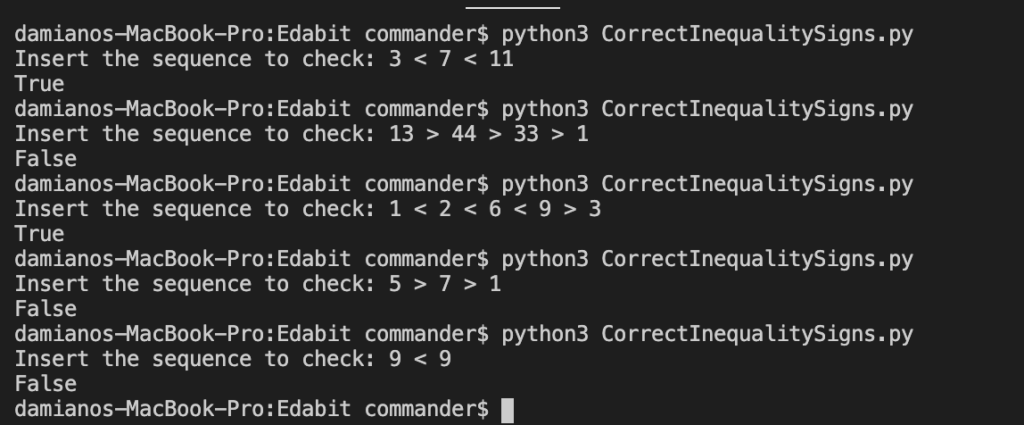
It works fine but, using the function Eval, we will able to write the test_signs method with only one row.
But, what is Eval()?
Eval function parses the expression argument and evaluates it as a python expression. In a nutshell, the eval function evaluates the “String” like a python expression and returns the result as an integer.
In simple words, we can write the code above in this way:
def test_signs(txt):
return eval(txt)
valInput = input("Insert the sequence to check: ")
print(test_signs(valInput))
If we run the code, this will be the result:
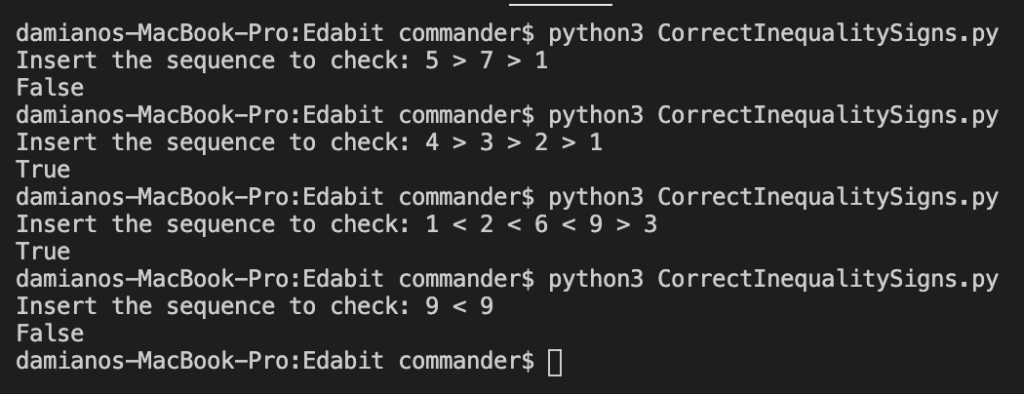