In this post, we will see three tips that can help us in develop of React applications.
CODESANDBOX
Codesandbox is an instant IDE and prototyping tool for rapid web development.
We can use it to create prototype for example in React, Angular or Vue, from anywhere, on any device without setup and using only a browser.
We open a browser, go to http://codesandbox.io, select “Create a sandbox” and finally we choose “React project”:


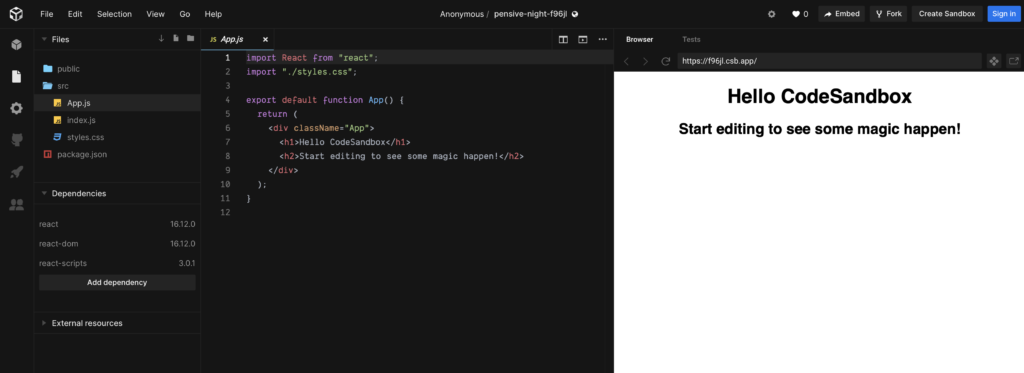
This is our Sandbox where we can develop our projects and in real time we can see the result:

BABELJS
Babeljs is a toolchain that is mainly used to convert ECMAScript 2015+ code into a backwards compatible version of JavaScript in current and older browsers or environments.
We open a browser, go to https://babeljs.io, and we select “Try it out”:
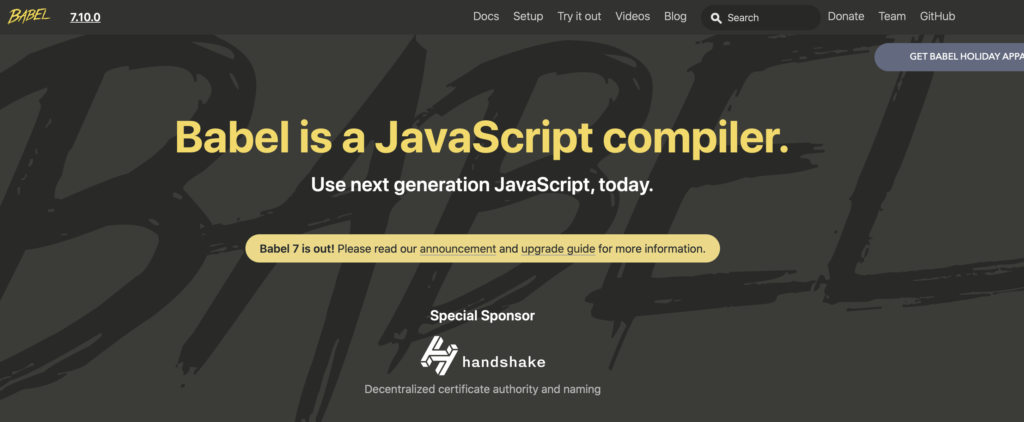
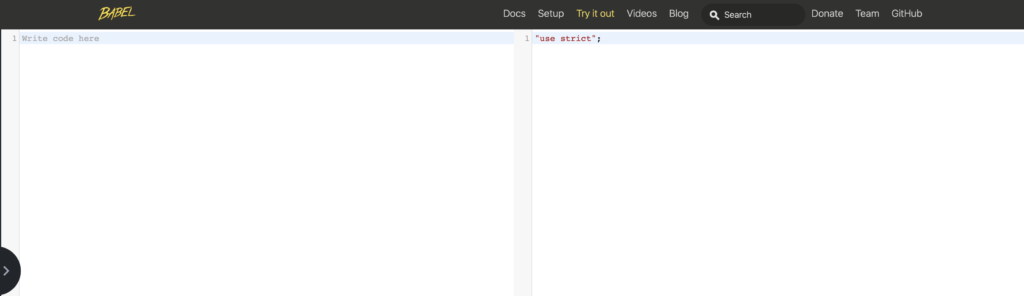
Now, if we take the function ShowName, created above, this will be the result:
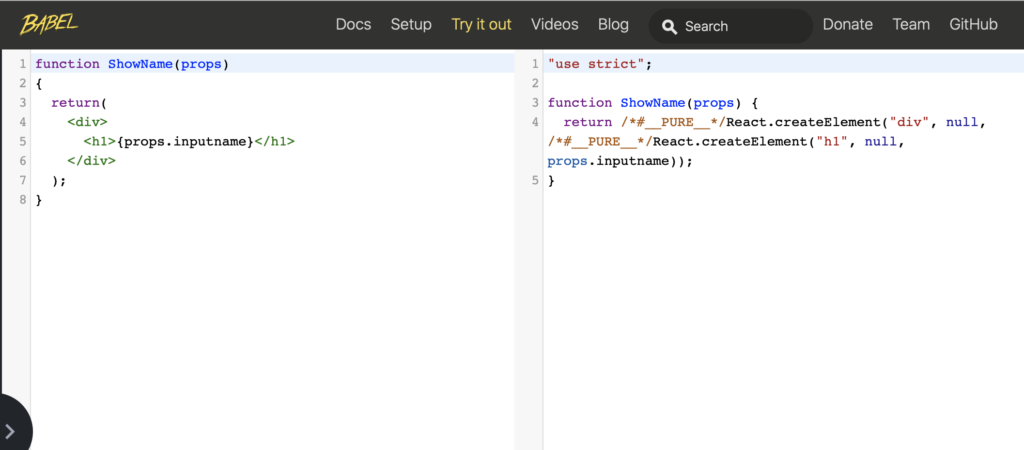
PROPS
We know that PROPS allow us to create a dynamic contents in React components.
For example, we define component called TestProps, where we pass in input two properties called Title1 and Title2:
[TESTPROPS.JS]
import React from 'react'
const TestProps = (props) => {
return(
<div>
<h1>{props.title1}</h1>
<br/>
<h1>{props.title2}</h1>
</div>
)
}
export default TestProps;
Then, we add TestProps in App.js:
[APPS.JS]
import React from 'react';
import './App.css';
import TestProps from './components/TestProps';
function App() {
return (
<div className="App">
<header className="App-header">
<TestProps title1="Title number 1" title2="Title number 2"/>
</header>
</div>
);
}
export default App;
Now, if we run the application, this will be the result:
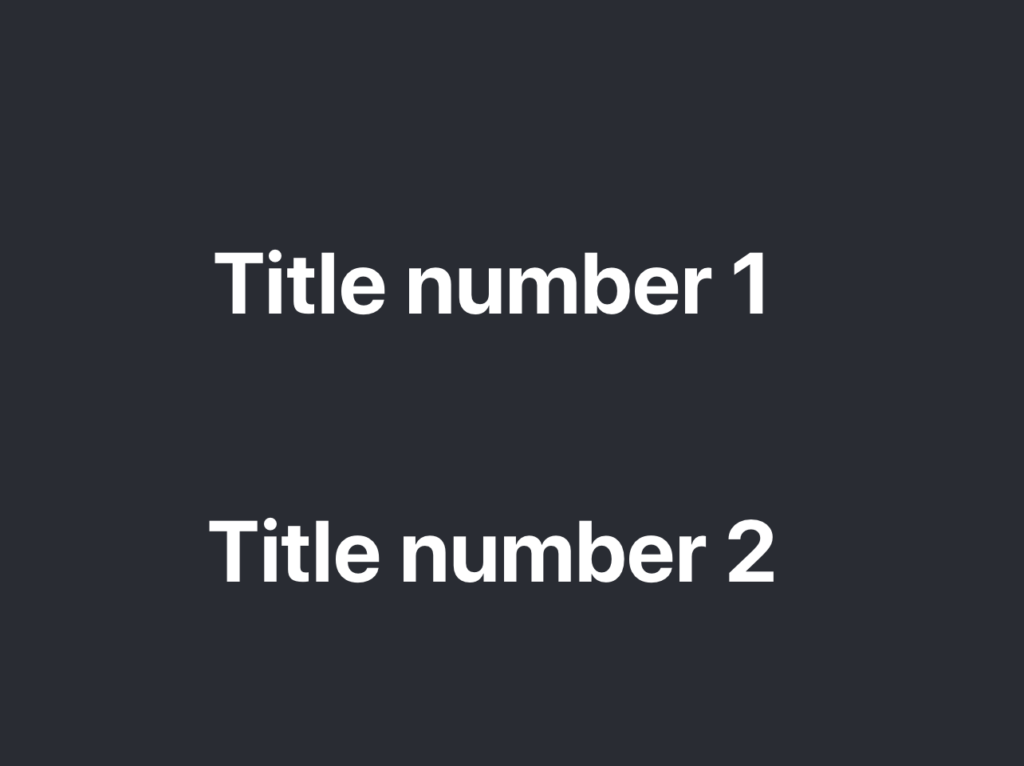
The tip is that there is an easier way to define and use Props in a React component: instead to pass a Props object, we can pass directly two parameters.
import React from 'react'
const TestProps = ({title1, title2}) => {
return(
<div>
<h1>{title1}</h1>
<br/>
<h1>{title2}</h1>
</div>
)
}
export default TestProps;