In this post, we will see what an Event is and how we can use it.
First of all, what is an Event?
From Microsoft web site:
“An event is a message sent by an object to signal the occurrence of an action. The action can be caused by user interaction, such as a button click, or it can result from some other program logic, such as changing a property’s value. The object that raises the event is called the event sender. The event sender doesn’t know which object or method will receive (handle) the events it raises. The event is typically a member of the event sender; for example, the Click event is a member of the Buttonclass, and the PropertyChanged event is a member of the class that implements the INotifyPropertyChanged interface.
To define an event, you use the C# event or the Visual Basic Event keyword in the signature of your event class, and specify the type of delegate for the event.”
In order to understand the Events, we will use an event and a delegate to run either a sum or a subtraction of two number:
[CALCULATOR.CS]
namespace PostDelegate
{
public class Calculator
{
// definition of a delegate
public delegate int Operation(int val1, int val2);
// definition of an event
public event Operation Calculate;
// definition of Sum
private int Sum (int val1, int val2)
{
return val1 + val2;
}
// definition of Subtraction
private int Subtraction(int val1, int val2)
{
return val1 - val2;
}
public Calculator(bool isSum)
{
// registration of the event
if (isSum)
{
this.Calculate += new Operation(this.Sum);
}
else
{
this.Calculate += new Operation(this.Subtraction);
}
}
public int RunOperation(int val1, int val2)
{
// fires the event
return Calculate(val1, val2);
}
}
}
Now, we will define the Main method in order to use the Calculator class:
[PROGRAM.CS]
using System;
namespace PostDelegate
{
class Program
{
static void Main(string[] args)
{
// creation an instance of Calculator
Calculator objCalculator = new Calculator(true);
Console.WriteLine($"The result of 5 + 5 is: {objCalculator.RunOperation(5,5)}");
// creation an instance of Calculator
Calculator objCalculator2 = new Calculator(false);
Console.WriteLine($"The result of 15 - 5 is: {objCalculator2.RunOperation(15, 5)}");
}
}
}
If we run the application, this will be the result:
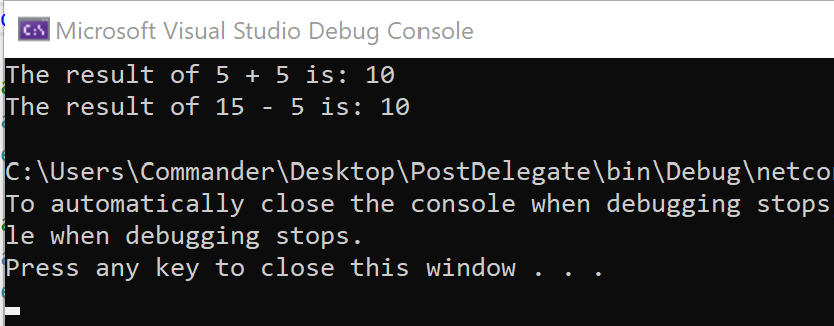