In this post, we will see how to use the Yield keyword in a C# application.
But first of all, what is Yield?
From Microsoft web site:
“When you use the yield contextual keyword in a statement, you indicate that the method, operator, or get accessor in which it appears is an iterator. Using yield to define an iterator removes the need for an explicit extra class (the class that holds the state for an enumeration, see IEnumerator for an example) when you implement the IEnumerable and IEnumerator pattern for a custom collection type.”
In a nutshell, with Yield return we can return each element one at a time and it allow us to do a full iteration without the need of creating a temporary collection.
We start creating a console application where we define a list of numbers and then, we will be read this list for running an operation for each item:
using System;
using System.Collections.Generic;
namespace Yield
{
class Program
{
static void Main(string[] args)
{
var list = CreateList(1000);
foreach (var item in list )
{
Console.WriteLine(((Int64)(item * item)).ToString());
}
}
private static List<Int64> CreateList(int maxValue)
{
List<Int64> lstResult = new List<Int64>();
for (int i = 1; i <= maxValue; i++)
{
lstResult.Add(i * i);
}
return lstResult;
}
}
}
If we run the application, this will be the result:
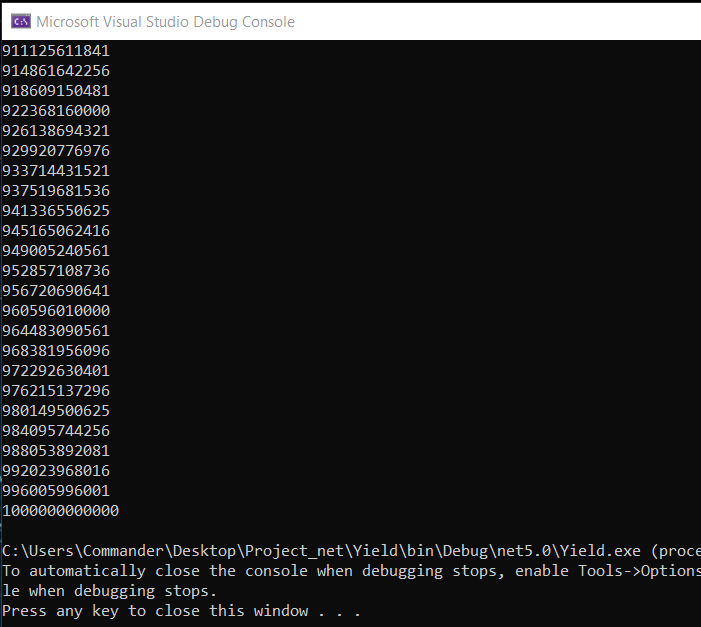
We can see that it works fine but we need to create a temporary list of number.
Instead, using Yield, we could modify the code avoiding to create a temporary list:
using System;
using System.Collections.Generic;
namespace Yield
{
class Program
{
static void Main(string[] args)
{
foreach (var item in GetValue(1000))
{
Console.WriteLine(((Int64)(item * item)).ToString());
}
}
private static IEnumerable<Int64> GetValue(int maxValue)
{
for (int i = 1; i <= maxValue; i++)
{
yield return i * i;
}
}
}
}
Obviously, if we run the application we will have the same result and maybe faster.
It is important to understand that with Yield we don’t exit from a method but, the system will continue to work.
For example, if we want to create a method in order to have the sum of a list of integer and in the same time we want to read the number that system is using, we can obtain it using the Yield:
using System;
using System.Collections.Generic;
namespace Yield
{
class Program
{
static int total = 0;
static void Main(string[] args)
{
foreach (var item in GetValue(20))
{
Console.WriteLine(item);
}
Console.WriteLine($"The result is: {total}");
}
private static IEnumerable<int> GetValue(int maxValue)
{
for (int i = 1; i <= maxValue; i++)
{
yield return i;
total += i;
}
}
}
}
If we run the application, this will be the result:
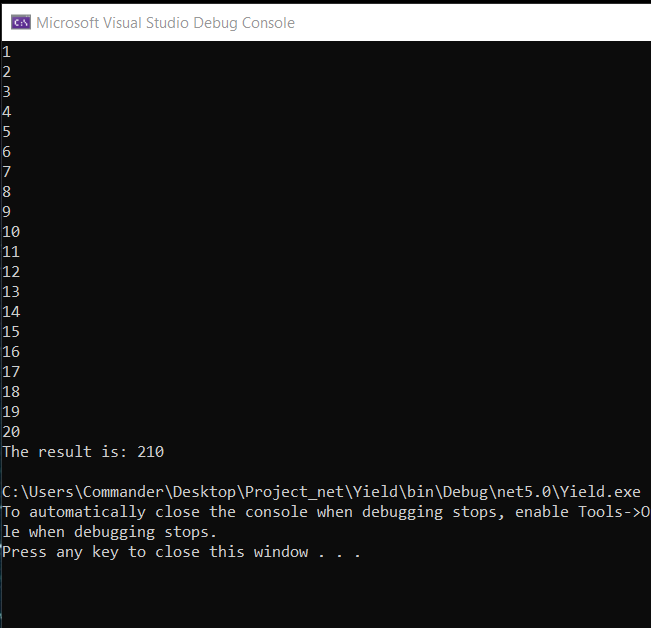
Finally, if we want to stop an iteration where we are using Yield, we have to use the command Yield break:
using System;
using System.Collections.Generic;
namespace Yield
{
class Program
{
static int total = 0;
static void Main(string[] args)
{
foreach (var item in GetValue(20))
{
Console.WriteLine(item);
}
Console.WriteLine($"The result is: {total}");
}
private static IEnumerable<int> GetValue(int maxValue)
{
for (int i = 1; i <= maxValue; i++)
{
yield return i;
total += i;
if (i == 6)
{
yield break;
}
}
}
}
}
If we run the application, this will be the result:
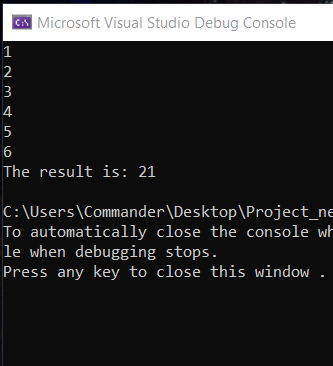