In this post, we will see how to read values from appsettings file in a Web API project.
We start creating a new Web API project where, we will add a section called AppInfo in the appsettings.json file:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AppInfo": {
"ApplicationName": "ReadConfigFile",
"Version": "1.0.0",
"Info": {
"CreatedBy": "Damiano"
}
},
"AllowedHosts": "*"
}
Then, we will create a new controller called ReadFileController where we will add three methods for reading the section AppInfo of appsettings.js file.
METHOD 1
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Options;
namespace ReadConfigFile.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ReadFileController : ControllerBase
{
private readonly IConfiguration _config;
public ReadFileController(IConfiguration config)
{
_config = config;
}
[HttpGet("v1/appinfo")]
public IActionResult ReadFile()
{
string appName = _config["AppInfo:ApplicationName"];
string appVersion = _config["AppInfo:Version"];
string createdBy = _config["AppInfo:Info:CreatedBy"];
return Ok($"{appName} - version: {appVersion} / createdby: {createdBy}");
}
}
}
If we run the application, this will be the result:
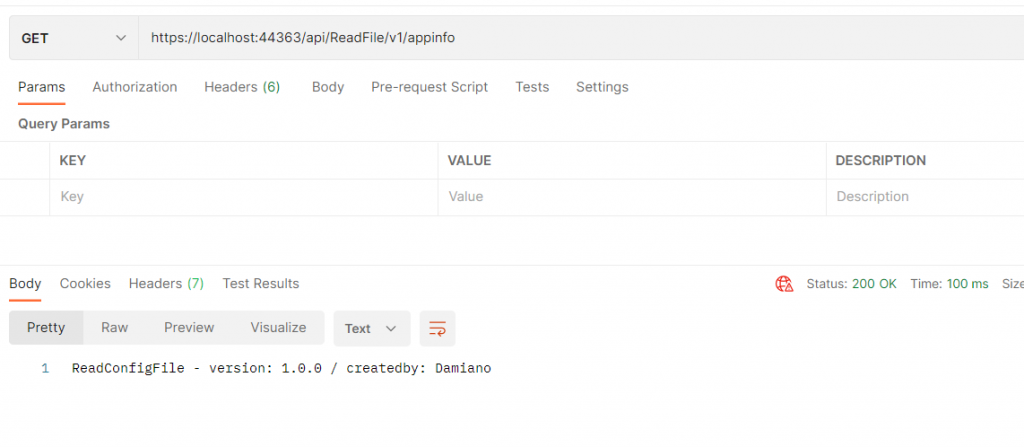
METHOD 2
First of all, we define two classes called Info and AppInfo with the same properties as we have defined in the section AppInfo of the appsettings.json file:
[INFO.CS]
namespace ReadConfigFile
{
public class Info
{
public string CreatedBy { get; set; }
}
}
[APPINFO.CS]
namespace ReadConfigFile
{
public class AppInfo
{
public string ApplicationName { get; set; }
public string Version { get; set; }
public Info Info { get; set; }
}
}
Then, we add the ReadFile2 method in ReadFileController:
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Options;
namespace ReadConfigFile.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ReadFileController : ControllerBase
{
private readonly IConfiguration _config;
private readonly AppInfo _appInfo;
public ReadFileController(IConfiguration config)
{
_config = config;
_appInfo = _config.GetSection(nameof(AppInfo)).Get<AppInfo>();
}
[HttpGet("v2/appinfo")]
public IActionResult ReadFile2()
{
return Ok($"{_appInfo.ApplicationName} - version: {_appInfo.Version} / createdby: {_appInfo.Info.CreatedBy}");
}
}
}
If we run the application, this will be the result:
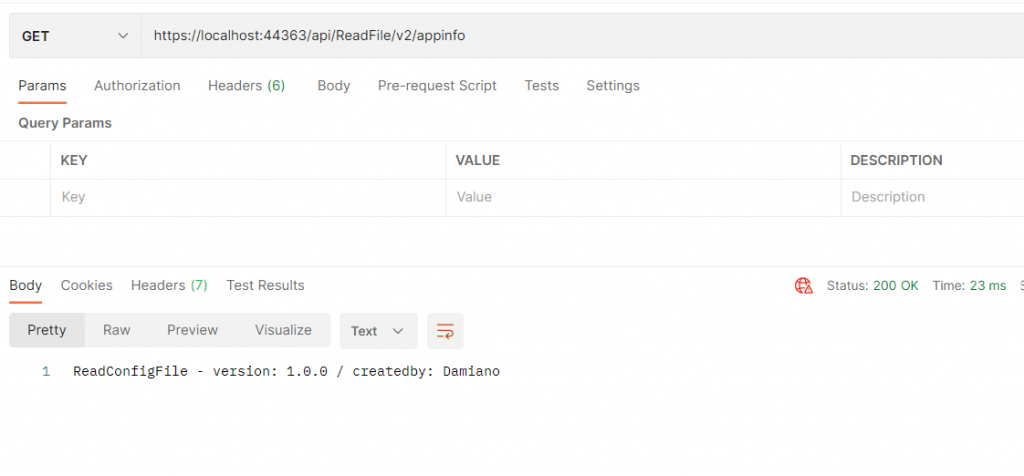
METHOD 3
This method is similar at the second one with only the difference that we will bind our classes with appsettings in the method ConfigureServices of Startup.cs file:
public void ConfigureServices(IServiceCollection services)
{
services.Configure<AppInfo>(Configuration.GetSection(nameof(AppInfo)));
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "ReadConfigFile", Version = "v1" });
});
}
Then, we will add the ReadFile3 method in ReadFileController:
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.Options;
namespace ReadConfigFile.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ReadFileController : ControllerBase
{
private readonly AppInfo _appInfo;
public ReadFileController(IOptions<AppInfo> appInfo)
{
_appInfo = appInfo.Value;
}
[HttpGet("v3/appinfo")]
public IActionResult ReadFile3()
{
return Ok($"{_appInfo.ApplicationName} - version: {_appInfo.Version} / createdby: {_appInfo.Info.CreatedBy}");
}
}
}
If we run the application, this will be the result:
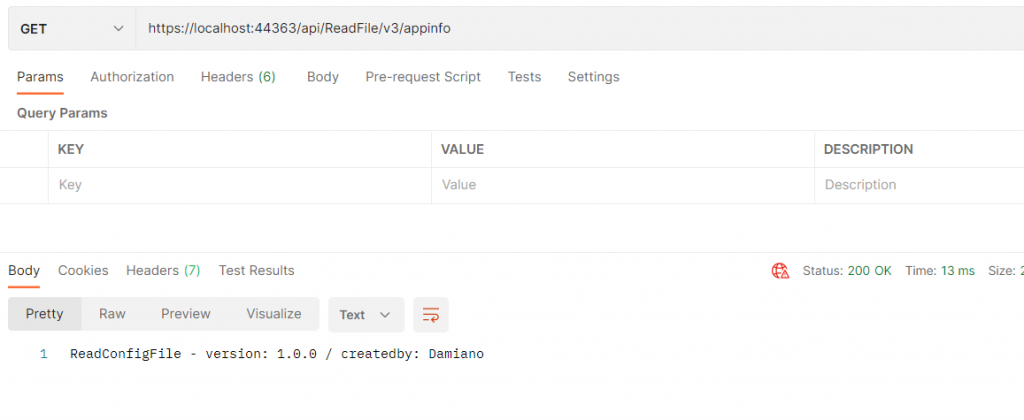