In this post, we will see how to use Struct in Swift.
But first of all, what is a Struct?:
From Apple web site:
“Use structures to represent common kinds of data. Structures in Swift include many features that are limited to classes in other languages: They can include stored properties, computed properties, and methods. Moreover, Swift structures can adopt protocols to gain behavior through default implementations. The Swift standard library and Foundation use structures for types you use frequently, such as numbers, strings, arrays, and dictionaries.“
STRUCT DEFINITION:
import UIKit
struct User {
let Username: String
let Password: String
var Password2: String = ""
func Greeting() {
print("Hello \(Username)")
print("Password \(Password)")
print("Password2 \(Password2)")
}
}
// we don't need to define Pasword2, because we defined a default value
var Admin = User(Username: "Admin", Password: "Pass1")
// We cannot change Password value, because we defined it as let
//Admin.Password = "Pass2"
// We can change Password2 value, because we defined it as var
Admin.Password2 = "Pass345"
Admin.Greeting()
print()
var Admin2 = User(Username: "Reader", Password: "Pass2", Password2: "Pass789")
Admin2.Greeting()
If we run the code, this will be the result:
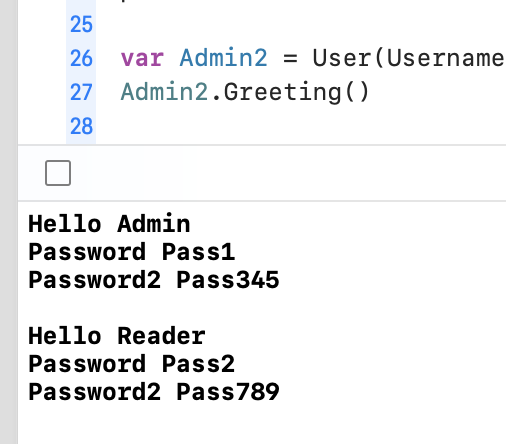
COMPUTE PROPERTY
struct Person {
var Name: String
// Compute property
var Age: Int{
return Int.random(in: 18...50)
}
}
var John = Person(Name: "John")
print("Hello, I am \(John.Name) and I am \(John.Age) years old")
If we run the code, this will be the result:
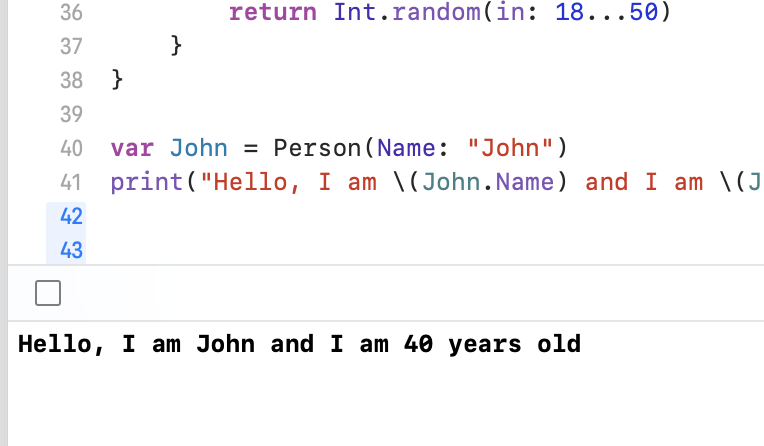
struct Person {
var Name: String
var StartAge: Int = 18
// Compute property with Get and Set
var Age: Int{
get{
StartAge
}
set{
StartAge += newValue
}
}
}
var Carol = Person(Name: "Carol")
// Age -> get
print("The start value of age is \(Carol.Age)")
// Age -> set
Carol.Age = 10
// Age -> get
print("The final value of age is \(Carol.Age)")
If we run the code, this will be the result:
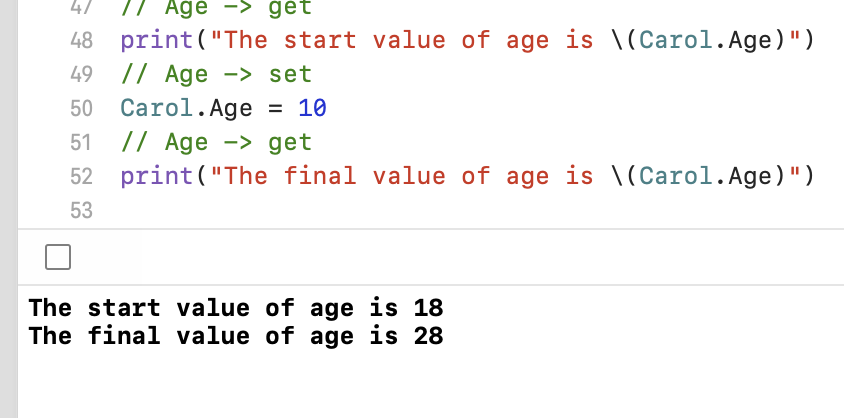
MUTATING FUNCTION
In a Struct, if we want to change a value of a variable in a function, we have to define the method as Mutating otherwise, we will receive an error:
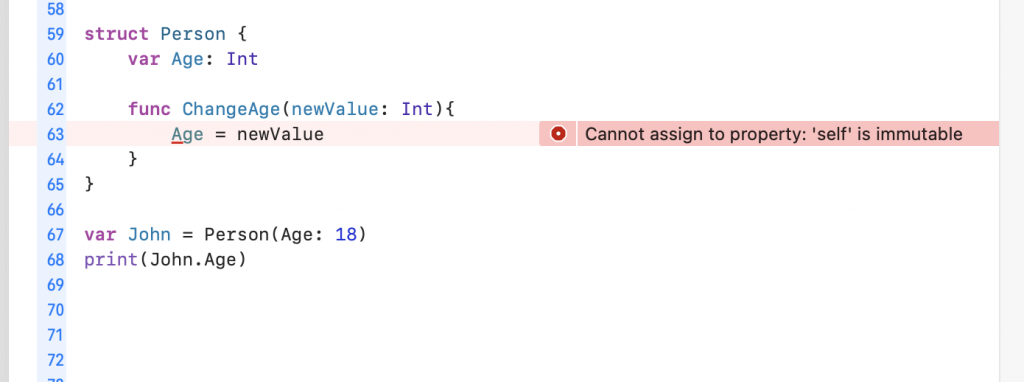
struct Person {
var Age: Int
mutating func ChangeAge(newValue: Int){
Age = newValue
}
}
var John = Person(Age: 18)
print(John.Age)
John.ChangeAge(newValue: 30)
print(John.Age)
If we run the code, this will be the result:
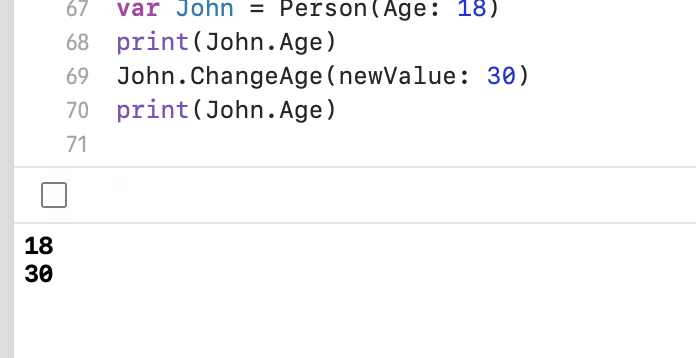
PROPERTY OBSERVERS
struct Person {
var Age: Int{
didSet{
print("The new value of Age is \(Age). The old value was \(oldValue)")
}
willSet{
print("The new value of Age is \(newValue). The old value was \(Age)")
}
}
}
var John = Person(Age: 18)
John.Age = 30
If we run the code, this will be the result:
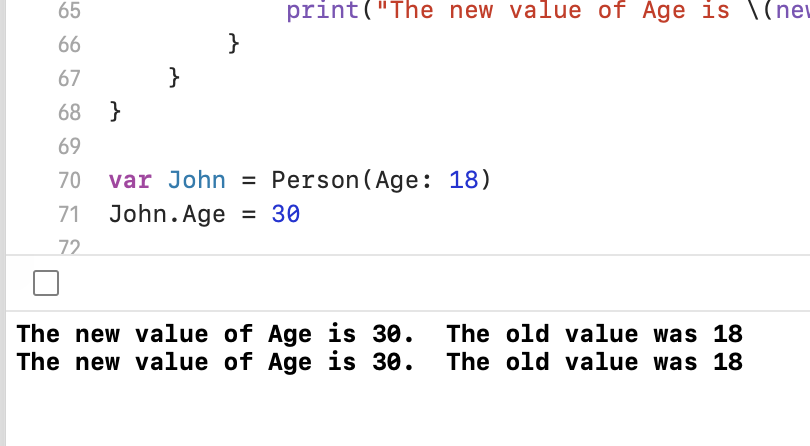
CUSTOM INITIALIZERS
struct Person {
var Name: String
var SurnName: String
var Age: Int = 18
func PrintInfo(){
print("\(SurnName) \(Name) - Age: \(Age)")
}
init(name: String, surname: String, age:Int)
{
self.Age = age
self.Name = name
self.SurnName = surname
}
init(name: String, surname:String)
{
self.Name = name
self.SurnName = surname
}
init(name: String)
{
self.Name = name
self.SurnName = "No Surname"
}
init(name: String, age: Int)
{
self.Name = name
self.SurnName = "No Surname"
self.Age = age
}
}
var person1 = Person(name: "Person1", surname: "Surname1")
person1.PrintInfo()
var person2 = Person(name: "Person2", surname: "Surname2", age: 30)
person2.PrintInfo()
var person3 = Person(name: "Person3")
person3.PrintInfo()
var person4 = Person(name: "Person4", age: 23)
person4.PrintInfo()
If we run the code, this will be the result:
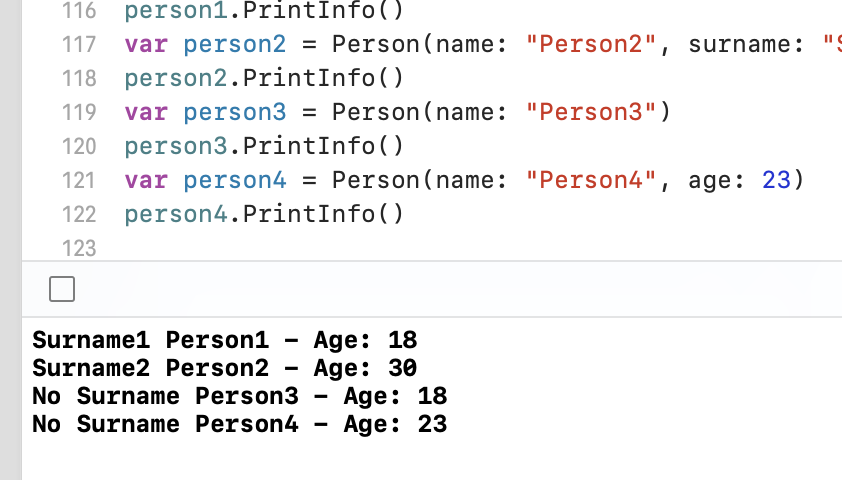