MULTIPLE INITIALIZERS
import UIKit
class User {
var _userName: String
var _password: String
var _email: String
init(UserName: String, Email: String, Password: String) {
self._userName = UserName
self._email = Email
self._password = Password
}
init(UserName: String, Email: String) {
self._userName = UserName
self._email = Email
self._password = "No Password"
}
init(UserName: String, Password: String) {
self._userName = UserName
self._password = Password
self._email = "No Email"
}
init(UserName: String) {
self._userName = UserName
self._password = "No Password"
self._email = "No Email"
}
func PrintInfo(){
print("Username: \(_userName) - Password: \(_password) - Email: \(_email)")
}
}
var UserComplete = User(UserName: "UserComplete", Email: "user@test.com", Password: "pass123")
var UserNoPassword = User(UserName: "UserNoPassword", Email: "user@test.com")
var UserNoEmail = User(UserName: "UserNoEmail", Password: "pass123")
var UserNoEmailNoPassword = User(UserName: "UserNoEmailNoPassword")
UserComplete.PrintInfo()
UserNoPassword.PrintInfo()
UserNoEmail.PrintInfo()
UserNoEmailNoPassword.PrintInfo()
If we run this code, these will be the results:
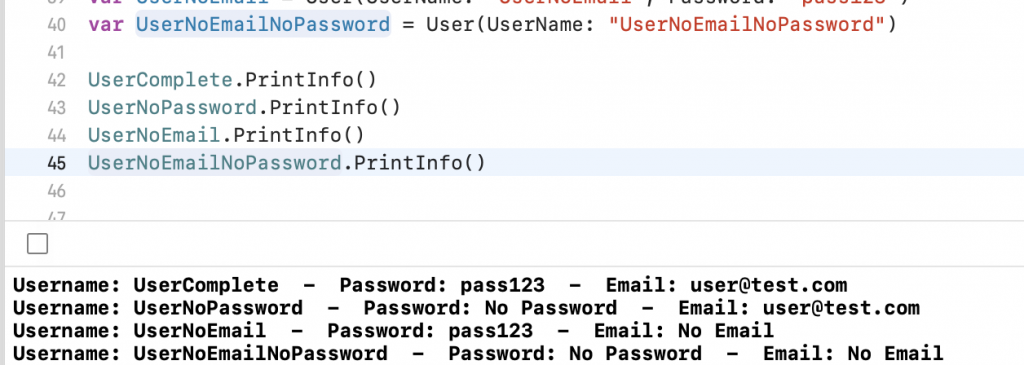
DEINITIALIZER
Deinitialization is a process used to deallocate the memory space before a class instance deallocated. The ‘deinit’ keyword is used to deallocate the memory spaces occupied by the system resources.
import UIKit
class User {
var _userName: String
var _password: String
var _email: String
init(UserName: String, Email: String, Password: String) {
self._userName = UserName
self._email = Email
self._password = Password
}
init(UserName: String, Email: String) {
self._userName = UserName
self._email = Email
self._password = "No Password"
}
init(UserName: String, Password: String) {
self._userName = UserName
self._password = Password
self._email = "No Email"
}
init(UserName: String) {
self._userName = UserName
self._password = "No Password"
self._email = "No Email"
}
func PrintInfo(){
print("Username: \(_userName) - Password: \(_password) - Email: \(_email)")
}
deinit {
print("The user \(_userName) has been destroyed")
}
}
var UserComplete = User(UserName: "UserComplete", Email: "user@test.com", Password: "pass123")
var UserNoPassword = User(UserName: "UserNoPassword", Email: "user@test.com")
var UserNoEmail = User(UserName: "UserNoEmail", Password: "pass123")
var UserNoEmailNoPassword = User(UserName: "UserNoEmailNoPassword")
UserComplete = User(UserName: "UserComplete2", Email: "user@test.com", Password: "pass123")
UserNoPassword = User(UserName: "UserNoPassword2", Email: "user@test.com")
UserNoEmail = User(UserName: "UserNoEmail2", Password: "pass123")
UserNoEmailNoPassword = User(UserName: "UserNoEmailNoPassword2")
If we run this code, these will be the results:
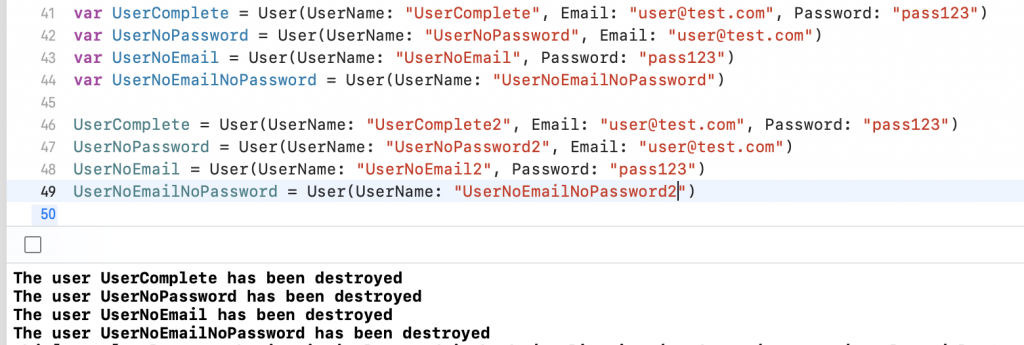
OVERRIDING METHOD
In swift, Overriding is the process in which subclass is fully responsible to change or re-implement the
instance method, instance property and type property which is defined in parent class or superclass.
import UIKit
class User {
var _name: String
init(Name: String) {
self._name = Name
}
func Print(){
print("Print from print() method \(_name)")
}
}
class Admin: User{
override func Print() {
print("Print from overridden print() method \(_name)")
}
func PrintNotOverridden(){
super.Print()
}
}
var objectUser = User(Name: "User")
var objectAdmin = Admin(Name: "Admin")
objectUser.Print()
objectAdmin.Print()
objectAdmin.PrintNotOverridden()
If we run this code, these will be the results:
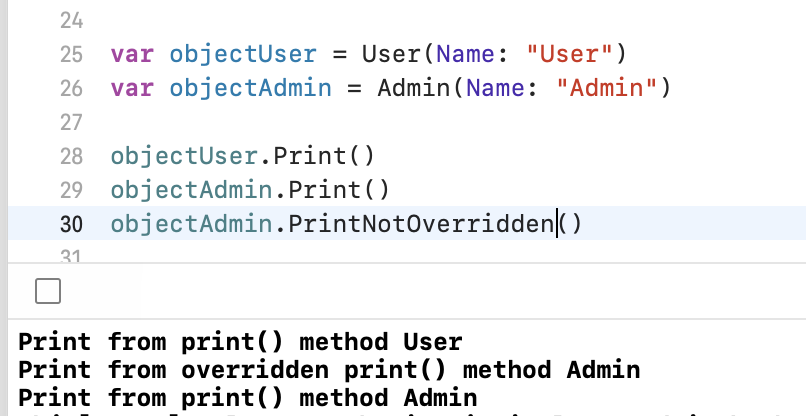