In this post, we will see three interesting features introduced with C# 10.
For the complete list of all new features go to Microsoft web site.
It is important to remember that for using C# 10 we need to install in our system .net 6.
We start opening VS 2022, we create a new Console Application project called TestC10 and we select Framework .net 6.0:

The first thing that we can see in this project, is that the file Program.cs has a new template:
[PROGRAM.CS]
// See https://aka.ms/new-console-template for more information
Console.WriteLine("Hello, World!");
At the beginning it looks like very strange but, we can see that nothing changed (we can check it in the link above the code) and it is just a code simplification.
In fact, if we change the code in this way:
[PROGRAM.CS]
// See https://aka.ms/new-console-template for more information
using System;
Console.WriteLine("Hello, World!");
Console.WriteLine($"Today is: {GetDate()}");
String GetDate()
{
return DateTime.Now.ToShortDateString();
}
and then we run the application, this will be the result:
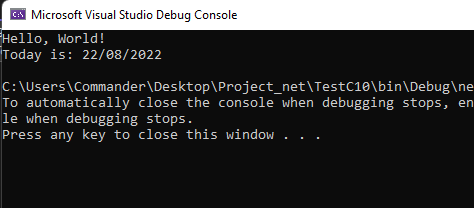
1) FILE-SCOPED NAMESPACE DECLARATION
This new feature allows us to declare a namespace with a different and maybe simpler syntax.
If we create a new class called Core and we add a method called PrintCurrentDate, the code will look like this:
[CORE.CS]
namespace TestC10
{
public class Core
{
public void PrintCurrentDate()
{
Console.WriteLine(DateTime.Now.ToShortDateString());
}
}
}
But, with C#10, we can modify it in this way:
[CORE.CS]
namespace TestC10;
public class Core
{
public void PrintCurrentDate()
{
Console.WriteLine($"Today is: {DateTime.Now.ToShortDateString()}");
}
}
Obviously, nothing will change in Program.cs where we use the Core class:
[PROGRAM.CS]
using TestC10;
Core objCore = new Core();
Console.WriteLine("Hello, World!");
objCore.PrintCurrentDate();

2) GLOBAL USING DIRECTIVES
With this new feature, we can add the global modifier to any using directive in order to applies this using to all source files in the project.
For example, we create another class called SecondCore where we will add a method called PrintDate.
In this method, we will use the method PrintCurrentDate of Core class:
[SECONDCORE.CS]
using TestC10;
namespace Second;
public class SecondCore
{
public void PrintDate()
{
Core objCore = new Core();
objCore.PrintCurrentDate();
}
}
[PROGRAM.CS]
using TestC10;
using Second;
Core objCore = new Core();
SecondCore objSecondCore = new SecondCore();
Console.WriteLine("Hello, World!");
objCore.PrintCurrentDate();
Console.WriteLine("Second Core");
objSecondCore.PrintDate();

We can see that in Program.cs we already use Core so, if we define “using TestC10” as a “global”, we should not to import it in the SecondCore class:
[PROGRAM.CS]
global using TestC10;
using Second;
Core objCore = new Core();
SecondCore objSecondCore = new SecondCore();
Console.WriteLine("Hello, World!");
objCore.PrintCurrentDate();
Console.WriteLine("Second Core");
objSecondCore.PrintDate();
[SECONDCORE.CS]
namespace Second;
public class SecondCore
{
public void PrintDate()
{
Core objCore = new Core();
objCore.PrintCurrentDate();
}
}
3) NULL PARAMETER CHECKING
This new feature allow us to check if a variable is null without using an IF statement.
In the class Core, we will add a new method called PrintValue so defined:
[CORE.CS]
namespace TestC10;
public class Core
{
public void PrintCurrentDate()
{
Console.WriteLine($"Today is: {DateTime.Now.ToShortDateString()}");
}
public void PrintValue(string parameterOne)
{
// we check if parameterOne is null
if(parameterOne == null)
{
throw new ArgumentNullException(nameof(parameterOne));
}
Console.WriteLine($"Your value is: {parameterOne}");
}
}
[PROGRAM.CS]
global using TestC10;
Core objCore = new Core();
string parameterValue = "Hello World";
try
{
objCore.PrintValue(parameterValue);
objCore.PrintValue(null);
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
}
If we run the application, this will be the result:

With C# 10, we can optimize the method PrintValue in the class Core:
[CORE.CS]
namespace TestC10;
public class Core
{
public void PrintCurrentDate()
{
Console.WriteLine($"Today is: {DateTime.Now.ToShortDateString()}");
}
public void PrintValue(string parameterOne)
{
// we check if parameterOne is null
ArgumentNullException.ThrowIfNull(parameterOne);
Console.WriteLine($"Your value is: {parameterOne}");
}
}
If we run the application, we will have the same result:
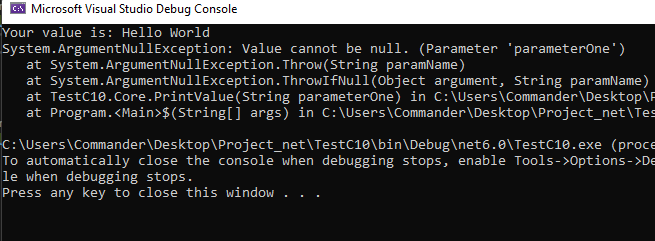