In this post, we will see two interesting features introduced with C# 11.
For the complete list of all new features, go to the Microsoft web site.
In order to use C# 11, we have to install .NET 7.0 that we can download here:
https://dotnet.microsoft.com/en-us/download/dotnet/7.0
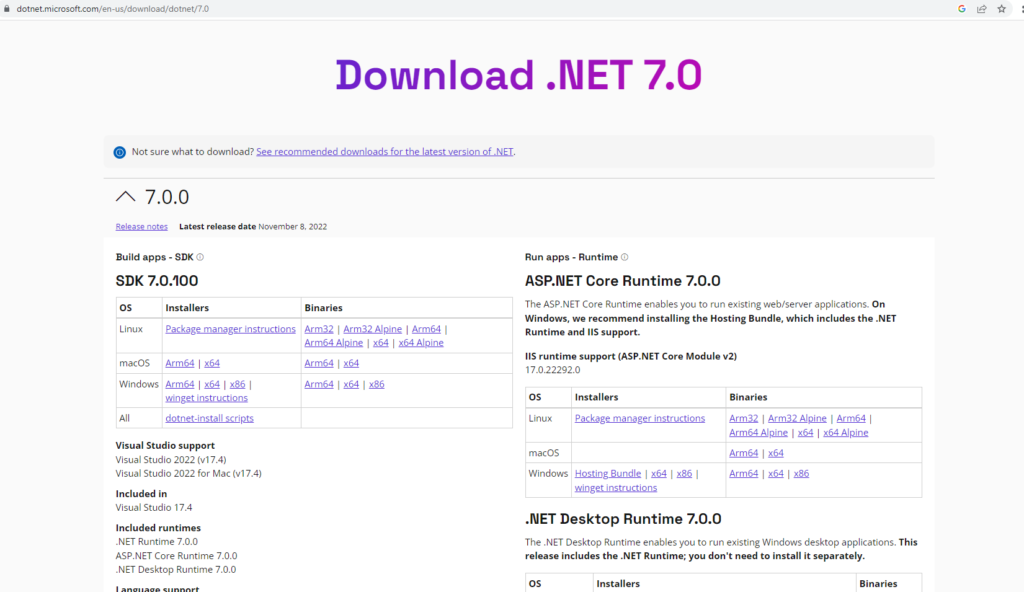
We select our OS (in my case Windows x64), we download the installation file and finally we install it:
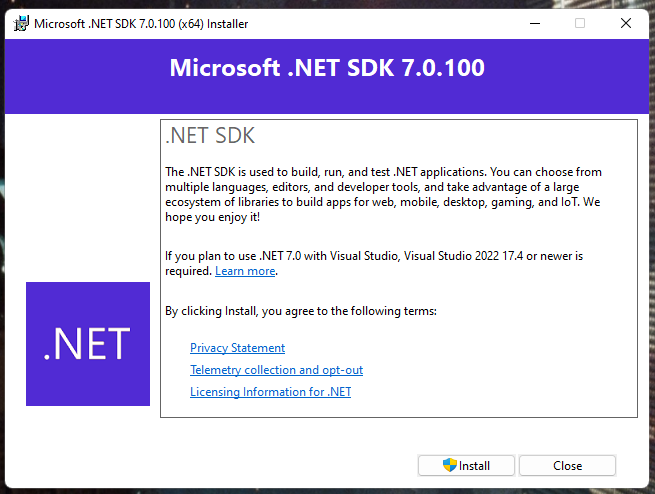
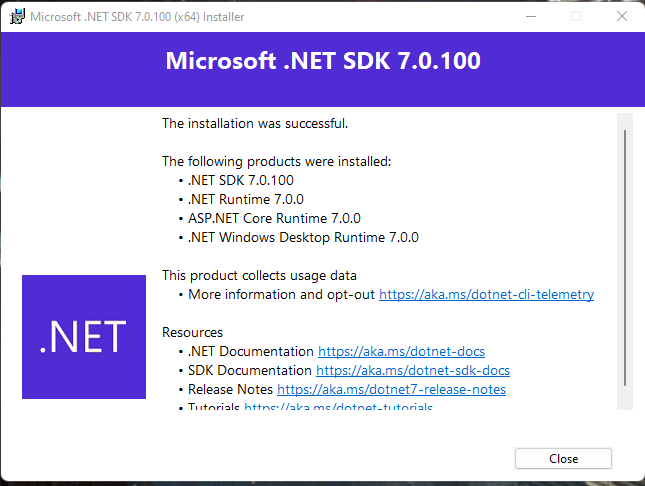
We have done and now, if we open Visual Studio and we create a Console Application, we will be able to select .NET 7.0:
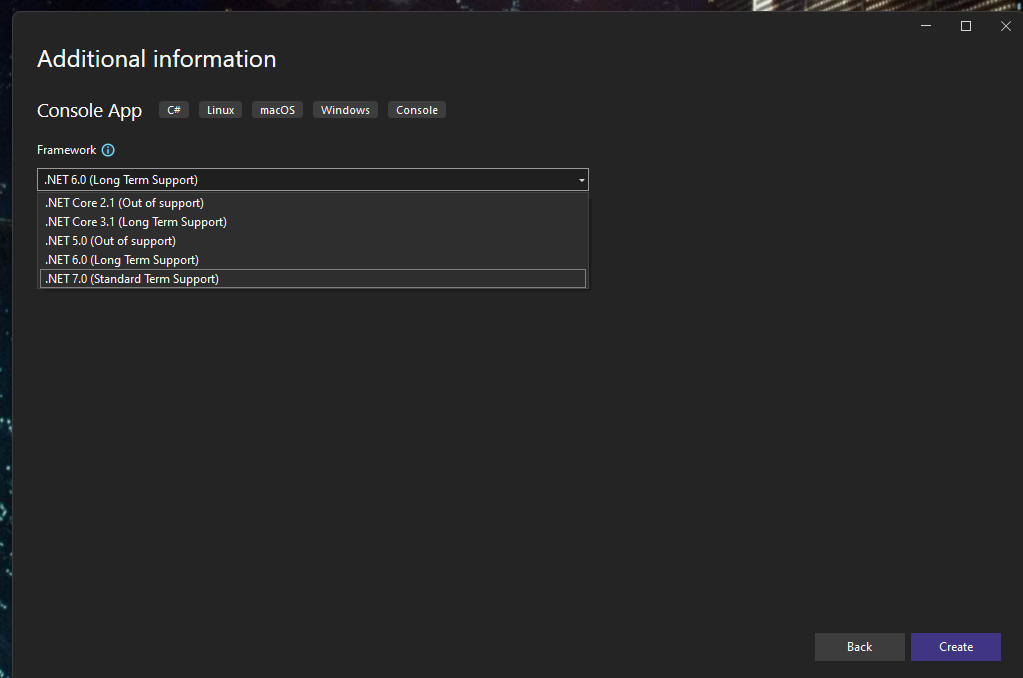
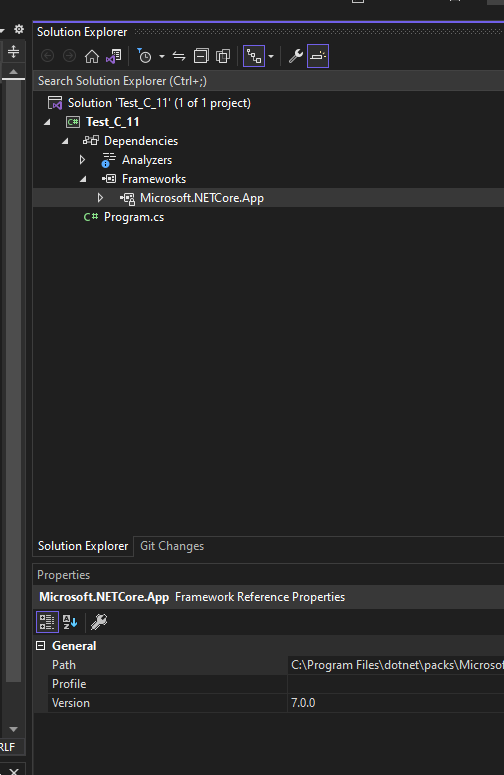
1) RAW STRING LITERALS
A raw string literals starts and ends with at least three double-quote “”” and it can contain text, whitespace, new lines, embedded quotes and other special characters:
string message = """
Hello World
I am Damiano!
How are you?
This is a example of a new "features" of C#11.
""";
Console.WriteLine(message);
If we run the code, this will be the result:
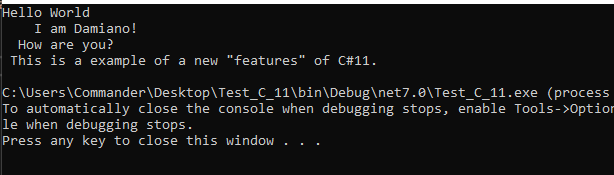
Obviously, we can combine it with string interpolation:
string name = "Damiano";
string greeting = "How are you?";
string message = $"""
Hello World
I am {name}!
{greeting}?
This is a example of a new "features" of C#11.
""";
Console.WriteLine(message);
If we run the code, this will be the result:
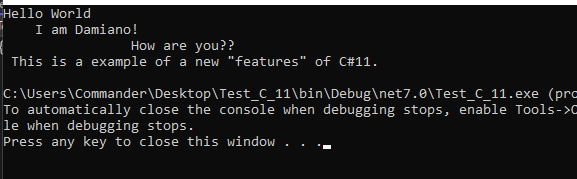
2) LIST PATTERN
List pattern extend pattern matching to match sequences of elements in a list or an array:
var numbers = new[] { 7, 9, 12, 16 };
// List and constant patterns
Console.WriteLine(numbers is [7, 9, 12, 16]); // True
Console.WriteLine(numbers is [7, 9, 12]); // False
// List and discard patterns
Console.WriteLine(numbers is [_, 9, _, 16]); // True
Console.WriteLine(numbers is [.., 12, _]); // True
// List and logical patterns
Console.WriteLine(numbers is [_, > 8, _, >=16]); // True
If we run the code, this will be the result:
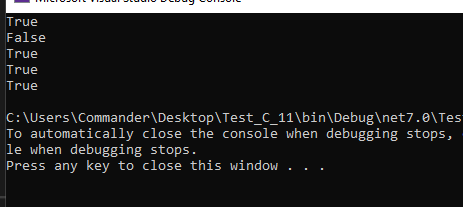
Obviously, we can use the List Pattern in an IF condition:
var numbers = new[] { 7, 9, 12, 16 };
if(numbers is [_,_,12,_])
{
Console.WriteLine("Ok - First if");
}
else
{
Console.WriteLine("No - First if");
}
if (numbers is [_, _, 11, _])
{
Console.WriteLine("Ok - Second if");
}
else
{
Console.WriteLine("No - Second if");
}
If we run the code, this will be the result:
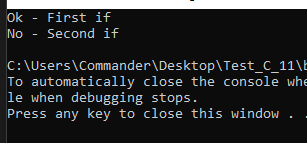