In this post, we will see what LINQ’s SequenceEqual is and how we can use it in our code.
But first of all, what is LINQ’s SequenceEqual?
LINQ’s SequenceEqual is a method used to determine if the elements of two sequences are equal.
It is important to highlight two things:
1) it is possible to use any type of sequences (objects as well)
2) keep in mind that when we work with large sequences, the performance of SequenceEqual can become a problem. This is especially true if the sequences are not already ordered.
In such cases, it may be more efficient to sort the sequences first or to use another approach to check for equality.
Let’s see some examples:
LIST OF INTEGERS
In this example, we create two lists called list1 and list2, each containing the same elements.
We then call the SequenceEqual method on list1, passing list2 as the argument. The method returns a boolean value indicating whether or not the two lists are equal. Finally, we print out the result of the comparison to the console.
[PROGRAM.CS]
Console.WriteLine("Testing SequenceEqual");
List<int> list1 = new List<int> { 1, 2, 3, 4 };
List<int> list2 = new List<int> { 1, 2, 3, 4 };
bool areEqual = list1.SequenceEqual(list2);
Console.WriteLine("The two lists are equal: " + areEqual);
If we run the program, the following will be the result:

LIST OF STRINGS
In this example, we create three lists: list1 and list2, each containing the same elements instead list3 is like the other two but with one less item element.
We call the SequenceEqual method on list1, passing list2 as the argument.
Then, we call the SequenceEqual method on list1, passing list3 as the argument.
Finally, we print out the result of the comparisons to the console.
[PROGRAM.CS]
Console.WriteLine("Testing SequenceEqual");
List<string> list1 = new List<string> { "A", "B", "C", "D" };
List<string> list2 = new List<string> { "A", "B", "C", "D" };
List<string> list3 = new List<string> { "A", "B", "C"};
bool areEqual = list1.SequenceEqual(list2);
Console.WriteLine("The list1 and list2 are equal: " + areEqual);
bool areEqual2 = list1.SequenceEqual(list3);
Console.WriteLine("The list1 and list3 are equal: " + areEqual2);
If we run the program, the following will be the result:
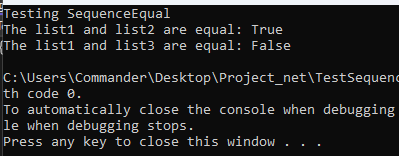
LIST OF OBJECTS
We said that we can use SequenceEqual to compare lists of objects. However, we need to be aware that SequenceEqual will compare the references to the objects in the lists, rather than their contents. This means that even if two objects have the same property values, they will not be considered equal by SequenceEqual if they are separate instances.
To compare the contents of two lists of classes using SequenceEqual, we need to implement a custom IEqualityComparer that compares the properties of the objects in the lists.
In this example, we define a Person class with Name and Age properties. We then define a PersonEqualityComparer class that implements IEqualityComparer<Person> to compare instances of the Person class. The Equals method compares the Name and Age properties of the objects, and the GetHashCode method generates a hash code based on those properties.
We create two lists of Person objects, list1 and list2, that contain the same objects. We then call the SequenceEqual method on list1, passing list2 as the argument, as well as an instance of PersonEqualityComparer to perform the comparison. The method returns a boolean value indicating whether or not the two lists are equal.
Finally, we will compare the two lists without use the PersonEqualityComparer.
[PERSON.CS]
namespace TestSequenceEqual;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
[PERSONEQUALITYCOMPARER.CS]
namespace TestSequenceEqual;
public class PersonEqualityComparer : IEqualityComparer<Person>
{
public bool Equals(Person x, Person y)
{
return x.Name == y.Name && x.Age == y.Age;
}
public int GetHashCode(Person obj)
{
return obj.Name.GetHashCode() ^ obj.Age.GetHashCode();
}
}
[PROGRAM.CS]
using TestSequenceEqual;
Console.WriteLine("Testing SequenceEqual");
var list1 = new List<Person>
{
new Person { Name = "Alice", Age = 30 },
new Person { Name = "Bob", Age = 40 },
new Person { Name = "Charlie", Age = 50 }
};
var list2 = new List<Person>
{
new Person { Name = "Alice", Age = 30 },
new Person { Name = "Bob", Age = 40 },
new Person { Name = "Charlie", Age = 50 }
};
bool areEqual = list1.SequenceEqual(list2, new PersonEqualityComparer());
bool areEqual2 = list1.SequenceEqual(list2);
Console.WriteLine("(with PersonEqualityComparer) The two lists are equal: " + areEqual);
Console.WriteLine("(without PersonEqualityComparer) The two lists are equal: " + areEqual2);
We have done and now, if we run the program, the following will be the result:
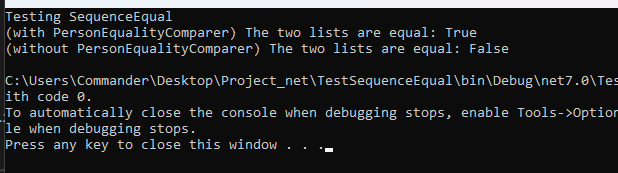