In this post, we will see how to manage Tuples in Python.
But first of all, what is a Tuple?
A tuple is a collection of immutable objects separated by commas.
CREATE A TUPLE
# empty tuple
tup1 = tuple()
print(f"tuple tup1: {tup1}")
# tuple
tup2 = 1,2
tup3 = 3,"4"
tup4 = ('Damiano', 'Test', 2019)
tup5 = (10,) * 6
print(f"tuple tup2: {tup2}")
print(f"tuple tup3: {tup3}")
print(f"tuple tup4: {tup4}")
print(f"tuple tup5: {tup5}")
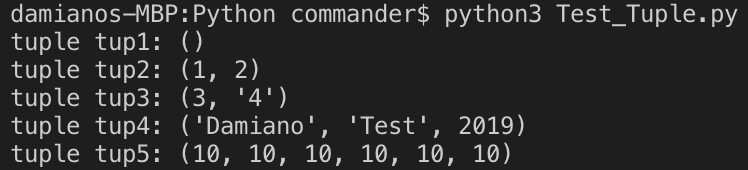
CONCATENATION
# Concatenation
tup6 = tup2 + tup3
print(f"tuple tup2: {tup2}")
print(f"tuple tup3: {tup3}")
print(f"tuple tup6: {tup6}")

NESTING
# Nesting
tup7 = (tup2,tup3)
print(f"tuple tup2: {tup2}")
print(f"tuple tup3: {tup3}")
print(f"tuple tup7: {tup7}")

GET VALUES
# Get values in a tuple
print(f"tuple tup6: {tup6}")
print(f"tuple tup6[0]: {tup6[0]}")
print(f"tuple tup6[3]: {tup6[3]}")
for i in tup6:
print(i)
print("Second loop")
for i in range(0,len(tup6)):
print(tup6[i])
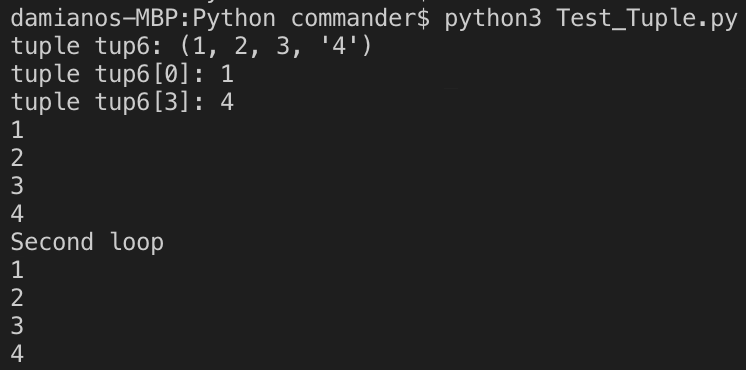
DELETE A TUPLE
# Delete tuple
print(f"tuple tup6: {tup6}")
del tup6
print(f"tuple tup6: {tup6}")
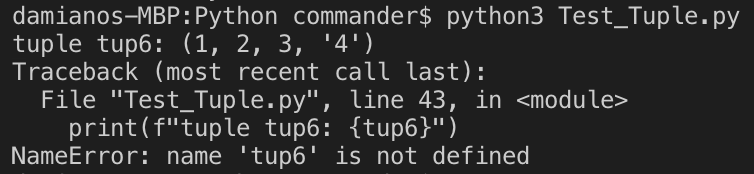
UPDATE
# Update tuple
print(f"tuple tup6: {tup6}")
tup6[0] = 5
print(f"tuple tup6: {tup6}")
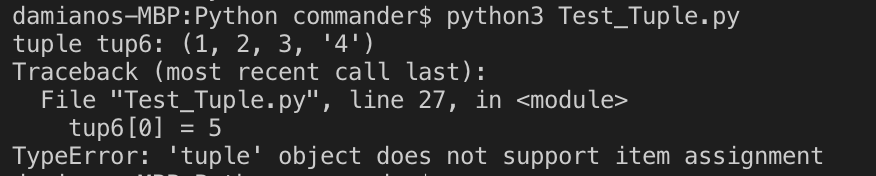
SEARCH
val1 = (1,"two", False, 1, 2, 4, 1)
# get the position of the first item found in the tupla
print(val1.index(1)) # -> 0
print(val1.index(2)) # -> 4
# get the number of items in the tupla
print(val1.count(1)) # -> 3
print(val1.count(4)) # -> 1
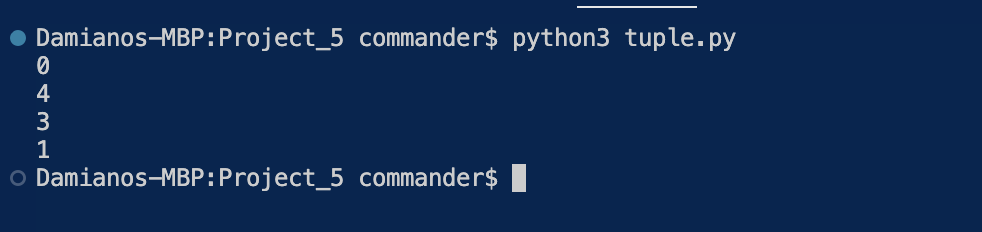
TUPLE IN ACTION
# define a method to find min and mix in a list
def maxmin(list):
valMax = max(list)
valMin = min(list)
return (valMax,valMin)
# define a list
lst = [1,2,3,4,5,6,7,9,0]
# call the method
findMaxMin = maxmin(lst)
print(f"Max value is:{findMaxMin[0]}")
print(f"Min value is:{findMaxMin[1]}")
