In this post, we will see how to create, modify and delete a file with Node.js.
For this post, we will use the file manageFile.js created in the post
How to manage file with Node.js – Part I (Read a file)
and a new file called FinalManage.js:
[FINALMANAGEFILE.JS]
var path = require('path');
const readline = require('readline-sync');
// definition of the directory where we will put the file to read
const dirFile = "data";
// read the name of file to find in the directory
let inputFileName = readline.question("Enter the name of the file to create: ");
// creration of the complete path
let fileName = path.join(dirFile,inputFileName);
CREATE AN EMPTY FILE
We add the method CreateFile in manageFile.js:
Function CreateFile(pathFile)
{
objFS.open(pathFile, 'w', function (err, file) {
if (err) throw err;
console.log('File was created successfully.');
});
}
module.exports = {
ReadFileCustom,
CreateFile
}
Finally, we add this method in the file FinalManageFile.js:
let fileOperation = require('./manageFile');
// create file
fileOperation.CreateFile(fileName);
If we run the application, this will be the result:

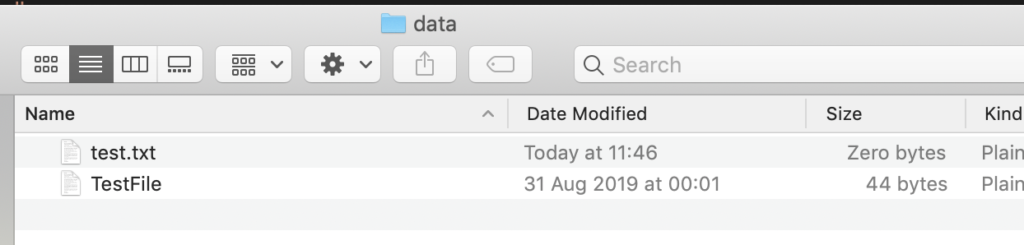
WRITE A FILE
We add the method CreateFile in manageFile.js:
// require 'os' to add new line after a new line was inserted
var os = require("os");
function WriteFile(pathFile, dataToWrite)
{
var newdatawithnewline = dataToWrite + os.EOL
objFS.writeFile(pathFile,newdatawithnewline, 'utf8',
function(err) {
if (err) throw err;
// if no error
console.log("Data was written to file successfully.")
});
}
module.exports = {
ReadFileCustom,
CreateFile,
WriteFile
}
Now, we add this method in the file FinalManageFile.js:
// write file
fileOperation.WriteFile(fileName, dataToWrite);
If we run the application, this will be the result:

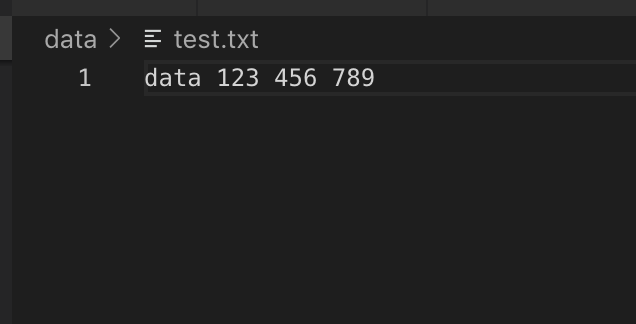
The command writeFile overwrite every time the data into the file.
If we want to add new data without delete nothing, we have to use the command appendfile.
APPEND
We add the method AppendData in manageFile.js:
function AppendData(pathFile, dataToWrite)
{
var newdatawithnewline = dataToWrite + os.EOL
objFS.appendFile(pathFile,newdatawithnewline, 'utf8',
function(err) {
if (err) throw err;
// if no error
console.log("Data was appended to file successfully.")
});
}
module.exports = {
ReadFileCustom,
CreateFile,
WriteFile,
AppendData
}
Now, we modify the code in FinalManageFile.js:
var path = require('path');
const readline = require('readline-sync');
// definition of the directory where put the file to read
const dirFile = "data";
let dataToWrite = readline.question("Enter data to write in the file: ");
// creration of the complete path
let fileName = path.join(dirFile,"test.txt");
let fileOperation = require('./manageFile');
// append data
fileOperation.AppendData(fileName, dataToWrite);
If we run the application, this will be the result:

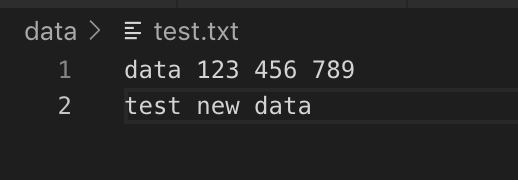
DELETE A FILE
We add the method DeleteFile in manageFile.js:
function DeleteFile(pathFile)
{
objFS.unlink(pathFile, function (err) {
if (err) throw err;
// if no error, file has been deleted successfully
console.log('File was deleted successfully.!');
});
}
module.exports = {
ReadFileCustom,
CreateFile,
WriteFile,
AppendData,
DeleteFile
}
Now, we modify the code in FinalManageFile.js:
var path = require('path');
const readline = require('readline-sync');
// definition of the directory where put the file to read
const dirFile = "data";
// creration of the complete path
let fileName = path.join(dirFile,"test.txt");
let fileOperation = require('./manageFile');
// delete file
fileOperation.DeleteFile(fileName);
If we run the application, this will be the result:

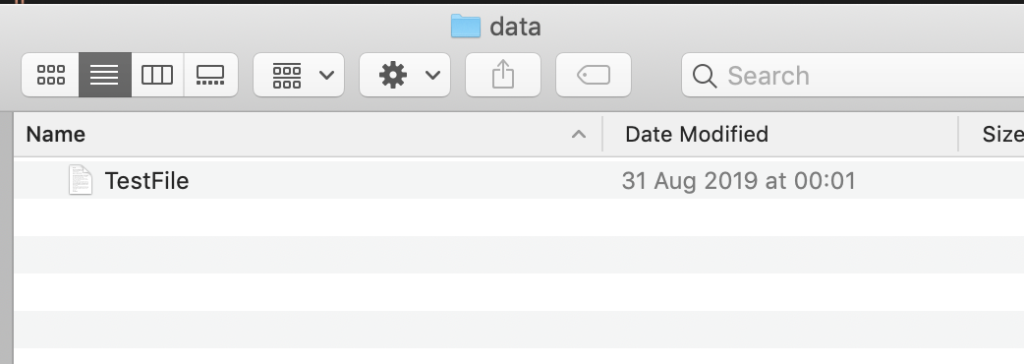