In this post, we will see how to manage Variables and Constants in Swift.
VARIABLES
// [Variable type inference]
var str = "Hello, playground"
str = "Hello World"
// [Variable type safe]
var str2: String = "Hello world 2"
var num: Int32 = 123
var verifyInput: Bool = true
var tot: Double = 120.65
// [Variable optional]
var val1: Int?
print(val1 as Any)
val1=4
print(val1 as Any)
// [Nil coalescing]
let val2 = val1 ?? 0
val1 = nil
let val3 = val1 ?? 0
// [Ternary operator]
var val4: String = "test"
var val5: Bool = val4 == "test1" ? true : false
var val6: Bool = val5 == false ? true : false
If we run this code in Playground, this will be the result:

CONSTANTS
// [Constant]
let val8: Int = 90
val8 = 100
If we run this code in Playground, this will be the result:
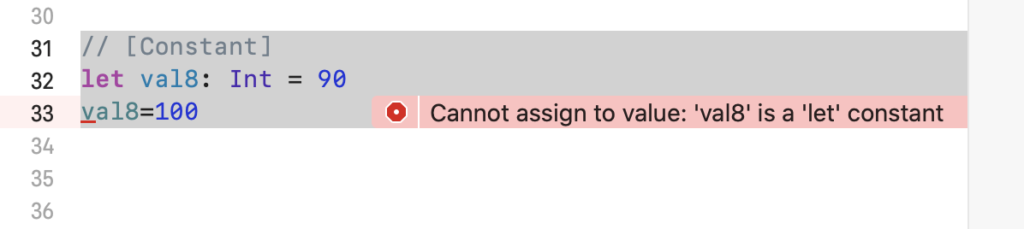