Dart is a programming language by Google for developing mobile, desktop, backend and web applications.
It is object-oriented with a C-style syntax and it is possible to run Dart code in three way:
1) Compiled as Javascript, in order to run in a web browser
2) Stand-alone using a Dart Virtual Machine
3) Ahead-of-time compiled into machine code.
An example of the last one, are the apps built using Flutter, the Google’s UI toolkit for building app for mobile, web and desktop.
For all information see the official web sites:
DART official web site
Flutter official web site
In this post, we will see how to install Dart and how it works.
First of all, we have to install the Dart SDK and we can see the page
https://dart.dev/get-dart#install to understand how to do it.
In my case with a Mac, I will install the SDK using Homebrew with the commands:
brew tap dart-lang/dart
brew install dart
Now, we have to decide which IDE we will use to write code and, once again, my choice is for Visual Studio Code.
We open Visual Studio Code and then we install the Dart extension:
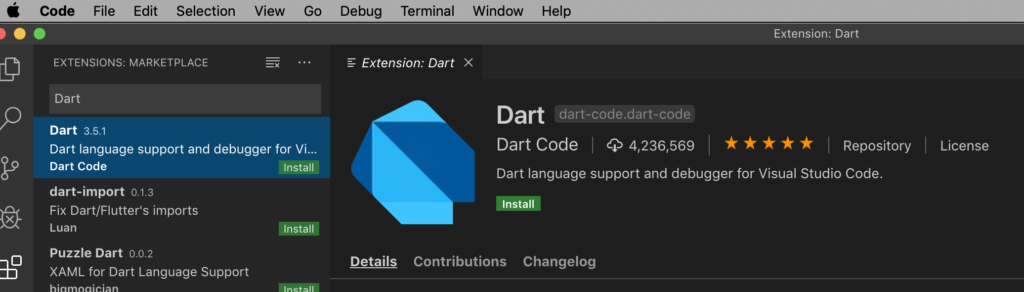
After the installation, we create a Dart file called first.dart:
// Main is where the app starts to run
main()
{
print("Hello world");
}
Now, we run the command dart first.dart, in order to run the application:

We have run our first Dart application!
Now, some piece of code to understand how Dart works:
VARIABLES
main()
{
//define variables
var value1 = 1;
String value2;
bool isReal = true;
double value3 = 4.56;
value2 = "First";
// We cannot change the value of value5
final value5 = 900;
print("$value1 $value2 $value5");
print(isReal.toString() + " " + value3.toString());
// With dynamic, it is possible to define a variable
// that can change the type
dynamic value4 = 1;
print(value4);
value4 = "Hello World";
print(value4);
}

CONDITIONALS AND LOOPS
main()
{
final max = 3;
print("For");
for (var i = 1; i<=max; i++){
print("Value$i");
}
print("\nFor with break");
for (var i = 1; i<=20; i++){
if(i == 10){
break;
}
if(i%2==0){
print("The number $i is even");
}
else{
print("The number $i is odd");
}
}
print("\nWhile");
var start = 10;
while(start>0){
print(start);
start--;
}
}
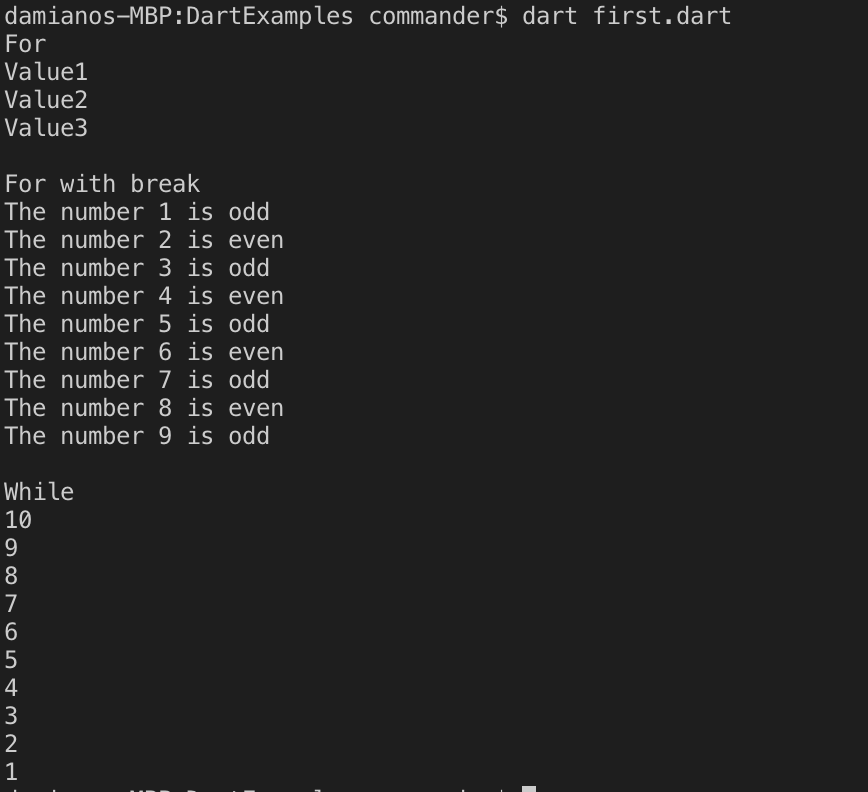
LIST
main()
{
// Create List
List lst1 = [1,2,3];
// Create empty List
List lst2 = [];
// print at index 1
print(lst1[1]);
print("First for");
for (var item in lst1) {
print(item);
}
// Add an object
lst1.add(4);
print("Second for");
for (var item in lst1) {
print(item);
}
// Remove object at position 0
lst1.removeAt(0);
print("Third for");
for (var item in lst1) {
print(item);
}
// delete List
lst1.clear();
print("Fourth for");
for (var item in lst1) {
print(item);
}
}
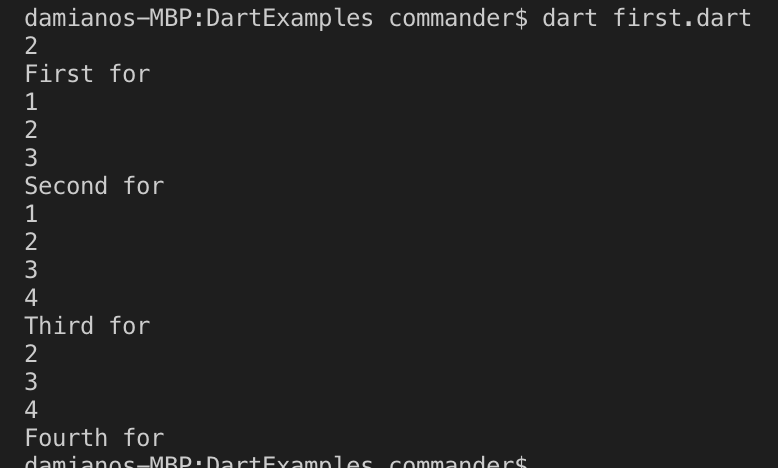
MAP
main()
{
// Create Map
var lstNumber = Map();
lstNumber[1] = "One";
lstNumber[2] = "Two";
lstNumber[3] = "Three";
print(lstNumber[3]);
lstNumber[3] = "four";
print(lstNumber[3]);
}

FUNCTIONS
// function with parameters in input and value in output
int sum(int val1, int val2){
return val1 + val2;
}
// function with parameters in input and without value in output
sum2(int val1, int val2){
print("Function sum2: " + (val1 + val2).toString());
}
// function with parameters (with default value) in input and value in output
int sum3(int val1, [int val2 = 40]){
return val1 + val2;
}
main()
{
var sumValue = sum(10,10);
print("Function sum: $sumValue");
sum2(20,20);
var sum3Value1 = sum3(30,30);
print("Function sum3: $sum3Value1");
var sum3Value2 = sum3(30);
print("Function sum3: $sum3Value2");
}

CLASS
class User
{
String Name;
int Age;
// Constructor
User(String inputName, int inputAge){
this.Name = inputName;
this.Age = inputAge;
}
// method
String PrintInfo()
{
return "Name: " + Name + " - Age: " + Age.toString();
}
}
class Operator extends User{
String Color;
Operator(String inputName, int inputAge, String inputColor) : super(inputName, inputAge)
{
this.Color = inputColor;
}
PrintColor()
{
print(this.Color);
}
}
main()
{
var objUser = new User("Damiano", 45);
print(objUser.PrintInfo());
var objOperator = new Operator("Dorian", 50, "Blue");
objOperator.PrintColor();
}
