From internet:
“Streams are a way to handle reading/writing files, network communications, or any kind of end-to-end information exchange in an efficient way.
What makes streams unique, is that instead of a program reading a file into memory all at once like in the traditional way, streams read chunks of data piece by piece, processing its content without keeping it all in memory.
This makes streams really powerful when working with large amounts of data, for example, a file size can be larger than your free memory space, making it impossible to read the whole file into the memory in order to process it. That’s where streams come to the rescue!
Using streams to process smaller chunks of data, makes it possible to read larger files“.
In this post, we will see how to read and write a file, using Streams in Node.js.
READ A FILE
This is the code used to read a file called README.md, that it is in the same directory of the node.js application:
// import FS to manage File System
var fs = require('fs');
// definition of the directory where the js file is running
var pathDir = __dirname;
// File path
var finalPath = pathDir + '/README.md';
// Creation of a readable stream
var fileReadStream = fs.createReadStream(finalPath, 'utf8');
// String where put all data from the file
var result = '';
//// Handle the filestream events for ReadStream
// receive chunk (piece od data)
fileReadStream.on('data', function(chunk) {
result += chunk;
});
// end chunck
fileReadStream.on('end', function() {
console.log("End Read data from file.");
console.log("*************************************************");
console.log("This is the data: " + result);
console.log("*************************************************");
});
// handle error
fileReadStream.on('error', function(error) {
console.log("Attention! There is an error: " + error.stack);
});
This is the project folder with the file README.md:
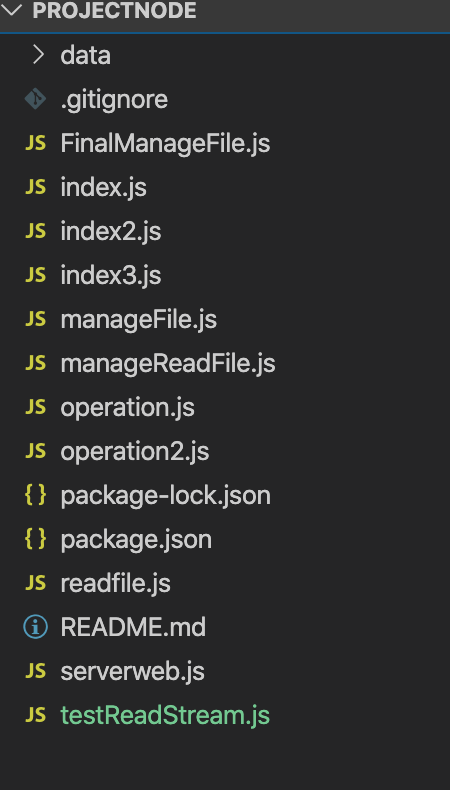
If we run the application, this will be the result:
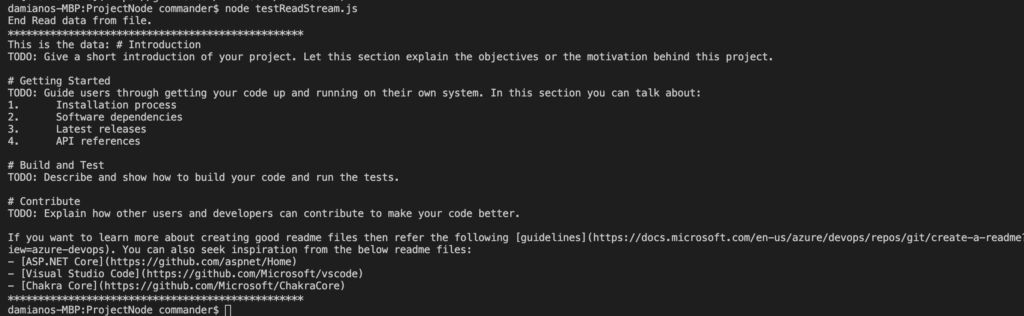
Write a file
This is the code used to read a file called README.md and write its content in a new file called NewFile.txt:
// import FS to manage File System
var fs = require('fs');
// Definition of the directory where the js file is running
var pathDir = __dirname;
// Read File path
var finalReadPath = pathDir + '/README.md';
// Write File path
var finalWritePath = pathDir + '/NewFile.txt'
// Creation of a readable stream
var fileReadStream = fs.createReadStream(finalReadPath, 'utf8');
// Creation of a writable stream
var fileWriteStream = fs.createWriteStream(finalWritePath, 'utf8');
//// Handle the filestream events for ReadStream
// receive chunk (piece od data)
fileReadStream.on('data', function(chunk) {
// everytime a chunck is ready, we will write it in the new file
fileWriteStream.write(chunk);
});
// end chunck from readfile
fileReadStream.on('end', function() {
console.log("End Read data from file.")
});
// handle error for readfile
fileReadStream.on('error', function(error) {
console.log("Attention! There is an error: " + error.stack);
});
//// Handle the filestream events for WriteStream
fileWriteStream.on('error', function(err) {
console.log("Attention! There is an error during the creation of the new file: " + error.stack);
});
If we run the application, this will be the result:


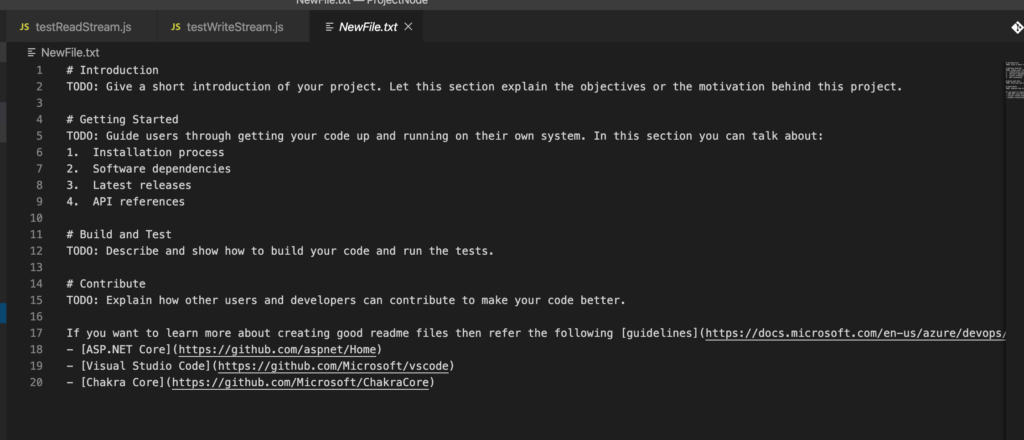