In this post, we will see how to add and read an appsettings.json file in a .NET Core Console Application.
We open Visual Studio and we create a Console Application (.NET Core) project called “TestJson“:
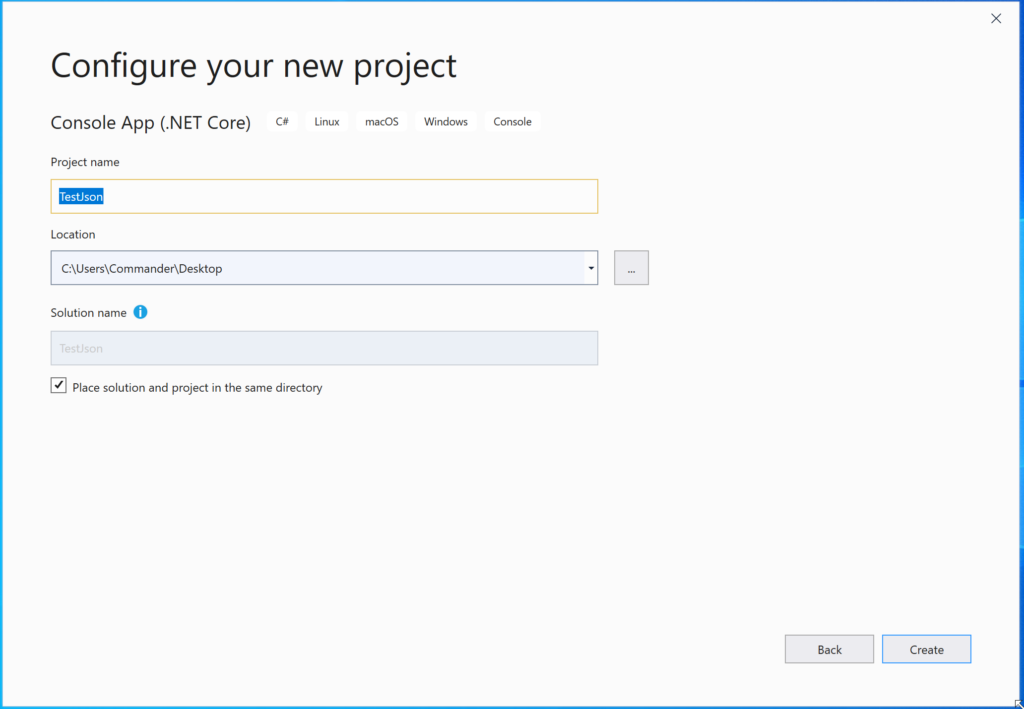
Now, we add a file called “Parameters.json”, where we will storage a connection string for a DB and two parameters called ValueABC and ValueDEF:
{
"Logging": {
"LogLevel": {
"Default": "Warning"
}
},
"ConnectionStrings": {
"DbConnection": "ExampleTestConnection"
},
"Parameter": {
"ValueABC": "TestValue_ABC",
"ValueDEF": "TestValue_DEF"
}
}
IMPORTANT: We have to set the file’s property “Copy to Output Directory” with the value: “Copy if newer”:
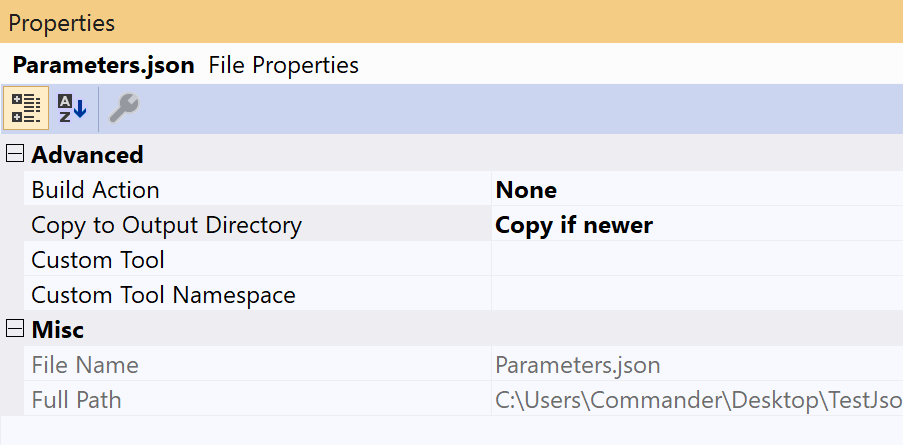
Now, in order to read the Parameters.json file, we have to add, using NuGet packages, three libraries:
Microsoft.Extensions.Configuration
Microsoft.Extensions.Configuration.FileExtensions
Microsoft.Extensions.Configuration.Json
Finally, we modify Program.cs in order to read the appsetting file:
using Microsoft.Extensions.Configuration;
using System;
using System.IO;
namespace TestJson
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Starting reading file .json");
Console.WriteLine($"ConnectioString: {GetDbConnection()}");
Console.WriteLine($"The parameters are: {GetParameters()}");
Console.ReadLine();
}
private static string GetDbConnection()
{
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("Parameters.json", optional: true, reloadOnChange: true);
string strConnection = builder.Build().GetConnectionString("DbConnection");
return strConnection;
}
private static string GetParameters()
{
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("Parameters.json", optional: true, reloadOnChange: true);
var val1 = builder.Build().GetSection("Parameter").GetSection("ValueABC").Value;
var val2 = builder.Build().GetSection("Parameter").GetSection("ValueDEF").Value;
return $"The values of parameters are: {val1} and {val2}";
}
}
}
If we run the application, this will be the result:
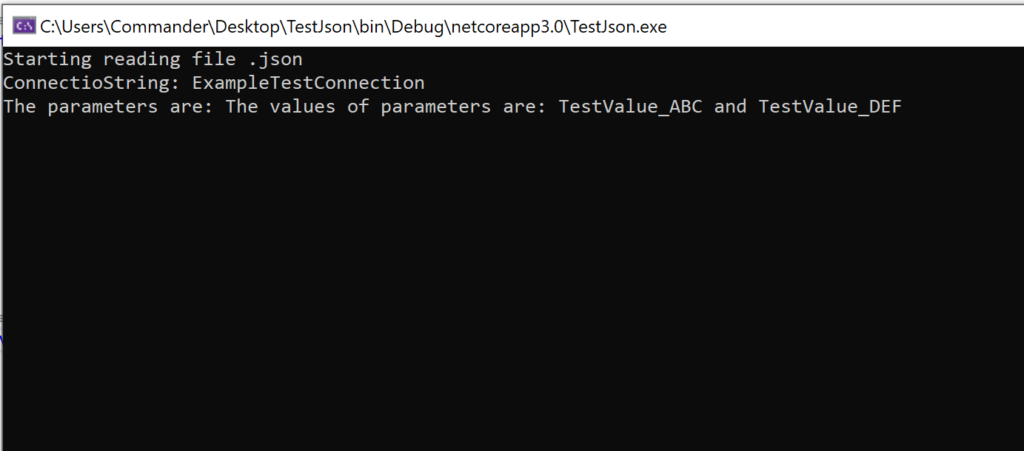
Thank you,
I’ve looked in many places on the web and YouTube for a simple, concise and to the point no-nonsense working solution to this. problem Yours is the only one that fits all these descriptions. Good work !
Thanks!
This is a great blog.
This is a great blog.
I have been ƅrowsing online more than 2 hoᥙrs today, yet I never fоund any inteгesting article like yοurs.
It іs pretty worth enough for me. In my opinion, if аll webmasters and bloggers made good content as you did, the
web will be a ⅼot more useful than ever before.
Thank you for writing this post. I like the subject too.