In this post, we will see how to pass data between components, using query string.
We start creating a project called PassData and installing Angular Material as well:
ng new PassData
ng add @angular/material
Then, we create three components called “Page1”, “Page2” and “Page3”:
ng g c Pages/Page1
ng g c Pages/Page2
ng g c Pages/Page3
Finally, we create an entity called “TestData”:
ng g class Entities/TestData
At the end, we should have a project like that:
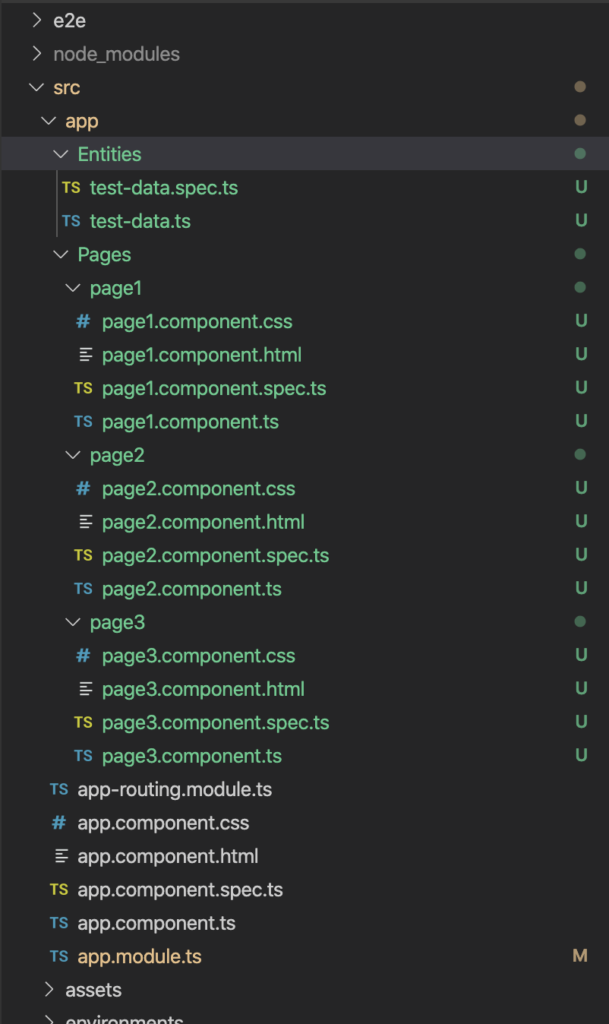
Now, we change the app-routing file, in order to use page1 and page2:
[APP-ROUTING.MODULE.TS]
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import {Page1Component} from './Pages/page1/page1.component';
import {Page2Component} from './Pages/page2/page2.component';
const routes: Routes = [
{ path: '', pathMatch: 'full', redirectTo: 'page1'},
{ path: 'page1', component: Page1Component },
{ path: 'page2', component: Page2Component },
{ path: '**', pathMatch: 'full', redirectTo: 'page1' }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Then, we define the entity TestData and we modify the files app.component.html and app.module.ts:
[TEST-DATA.TS]
export class TestData {
Reference: string;
Value: number;
}
[APP.COMPONENT.HTML]
<router-outlet></router-outlet>
[APP.MODULE.TS]
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { MatNativeDateModule,MatDatepickerModule,MatIconModule,
MatButtonModule,MatCheckboxModule, MatToolbarModule,
MatCardModule,MatFormFieldModule,MatInputModule,MatRadioModule,
MatListModule } from '@angular/material';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { Page1Component } from './Pages/page1/page1.component';
import { Page2Component } from './Pages/page2/page2.component';
import { Page3Component } from './Pages/page3/page3.component';
@NgModule({
declarations: [
AppComponent,
Page1Component,
Page2Component,
Page3Component
],
imports: [
BrowserModule,
AppRoutingModule,
BrowserAnimationsModule,
FormsModule,
BrowserAnimationsModule,
MatNativeDateModule,
MatDatepickerModule,
MatIconModule,
MatButtonModule,
MatCheckboxModule,
MatToolbarModule,
MatCardModule,
MatFormFieldModule,
MatInputModule,
MatRadioModule,
MatListModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Finally, we define Page1 and Page2, that we will use in this post.
[PAGE1.COMPONENT.TS]
import { Component, OnInit} from '@angular/core';
import { Router, NavigationExtras } from '@angular/router';
import { TestData } from '../../Entities/test-data';
@Component({
selector: 'app-page1',
templateUrl: './page1.component.html',
styleUrls: ['./page1.component.css']
})
export class Page1Component implements OnInit {
constructor(private router: Router) { }
objTestData: TestData = new TestData();
ngOnInit() {
}
Send()
{
// we define a NavigationExtras where
// we put, in the queryParams, Reference and Value
let navigationExtras: NavigationExtras = {
queryParams: {
Reference: this.objTestData.Reference,
Value: this.objTestData.Value
}
};
// we pass the NavigationExtras in the route.navigate
this.router.navigate(["page2"], navigationExtras);
}
}
[PAGE1.COMPONENT.HTML]
<mat-toolbar>
<span>Pass Data</span>
</mat-toolbar>
<mat-card class="my-card">
<mat-card-content>
<form class="my-form" (ngSubmit)="Send()" #TestDataForm="ngForm">
<mat-form-field class="full-width">
<mat-label>Reference</mat-label>
<input matInput placeholder="Loan Reference" [(ngModel)]="objTestData.Reference" name="Reference" #Reference="ngModel" required>
</mat-form-field>
<mat-form-field class="full-width">
<mat-label>Value</mat-label>
<input matInput placeholder="Value" [(ngModel)]="objTestData.Value" name="Value" #Value="ngModel" required>
</mat-form-field>
<mat-card-actions>
<button type="submit" mat-raised-button [disabled]="!TestDataForm.form.valid">Send via Querystring</button>
</mat-card-actions>
</form>
</mat-card-content>
</mat-card>
[PAGE1.COMPONENT.CSS]
.my-form{
min-width: 150px;
max-width: 500px;
width: 100%;
}
.full-width {
width: 100%;
}
[PAGE2.COMPONENT.TS]
import { Component, OnInit } from '@angular/core';
import { Router, ActivatedRoute } from '@angular/router';
import { TestData } from '../../Entities/test-data';
@Component({
selector: 'app-page2',
templateUrl: './page2.component.html',
styleUrls: ['./page2.component.css']
})
export class Page2Component implements OnInit {
objTestData: TestData = new TestData();
constructor(private router: Router, private activatedRoute: ActivatedRoute)
{
// using activatedRoute we can get the parameters
// that we put in the previous page
this.activatedRoute.queryParams.subscribe(params => {
this.objTestData.Reference = params["Reference"];
this.objTestData.Value = params["Value"];
});
}
ngOnInit() {
}
Back()
{
this.router.navigate(['/page1']);
}
}
[PAGE2.COMPONENT.HTML]
<mat-toolbar>
<span>Data in Page2</span>
</mat-toolbar>
<mat-card class="my-card">
<mat-card-content>
<input matInput placeholder="TestData Reference" [value]="objTestData.Reference" name="Reference" >
<input matInput placeholder="TestData Value" [value]="objTestData.Value" name="Value">
</mat-card-content>
<mat-card-content>
<button mat-raised-button (click)="Back()" color="primary">Back</button>
</mat-card-content>
</mat-card>
We have done and, if we run the application, this will be the result:
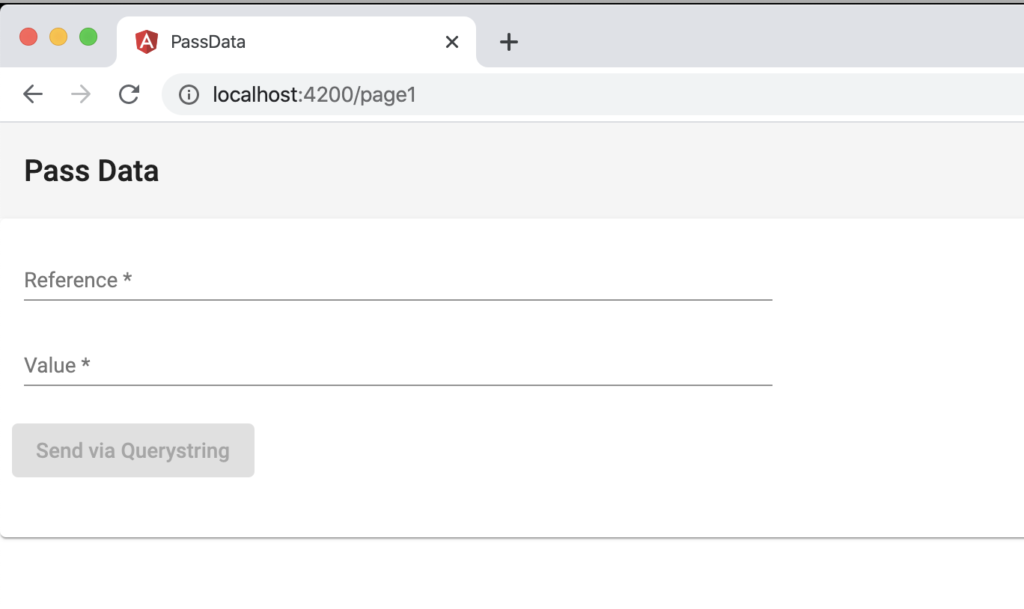
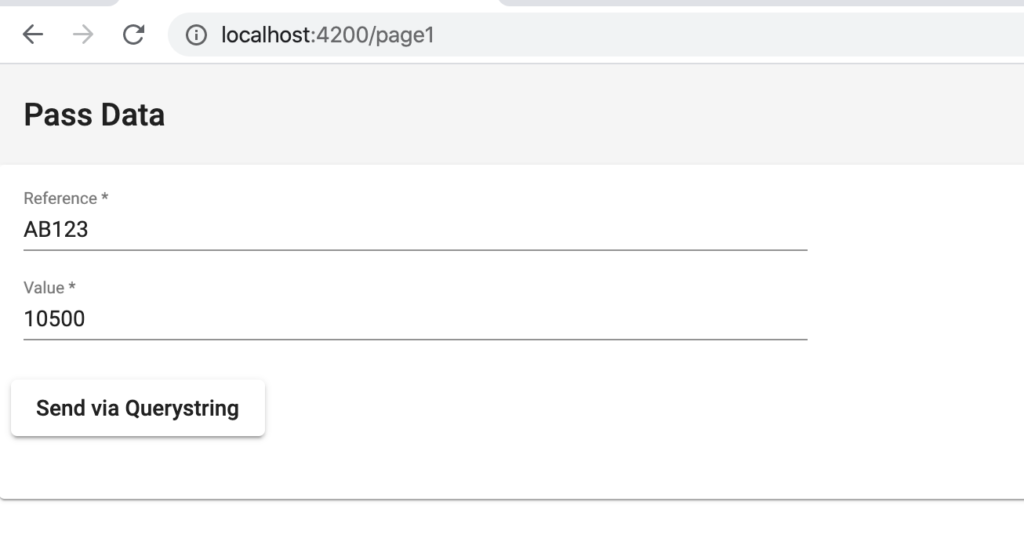
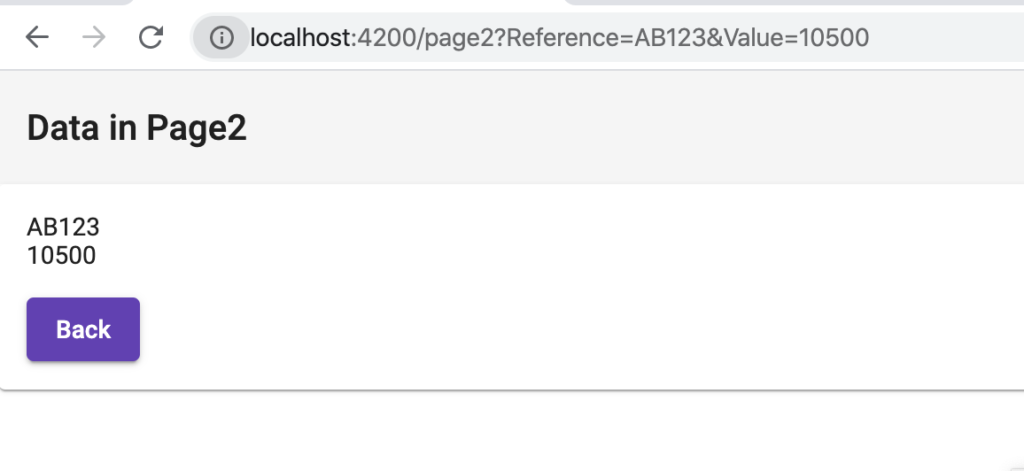