In this post, we will see how to render a list of objects in React.
First of all, we create a function component called listuser, where we will define a list of users:
[LISTUSER.JS]
import React from 'react'
function ListUser() {
const lstUsers = [
{
id:10,
userName: 'Admin01',
password: '$pass1',
createdAt: '04/20/2020'
},
{
id:11,
userName: 'Reader10',
password: '$read1',
createdAt: '04/20/2020'
},
{
id:12,
userName: 'Reader20',
password: '$pass20',
createdAt: '04/20/2020'
},
{
id:13,
userName: 'TestA1',
password: '$ttes1',
createdAt: '"04/20/2020'
},
{
id:14,
userName: 'TestA3',
password: '$ttes3',
createdAt: '04/20/2020'
}
]
return (
<div>
</div>
)
}
export default ListUser
Then, using the Javascript method map, we will display the list:
const result = lstUsers.map(User => (
<div key={User.id}>ID: {User.id} - UserName:{User.userName} - Password:{User.password} - Created At:{User.createdAt}</div>
))
return (
<div>
{result}
</div>
)
}
export default ListUsers
Finally, we add the component listuser in App.js:
import React from 'react';
import './App.css';
import LstUsers from './components/listuser';
function App() {
return (
<div className="App">
<header className="App-header">
<LstUsers></LstUsers>
</header>
</div>
);
}
export default App;
If we run the application, this will be the result:
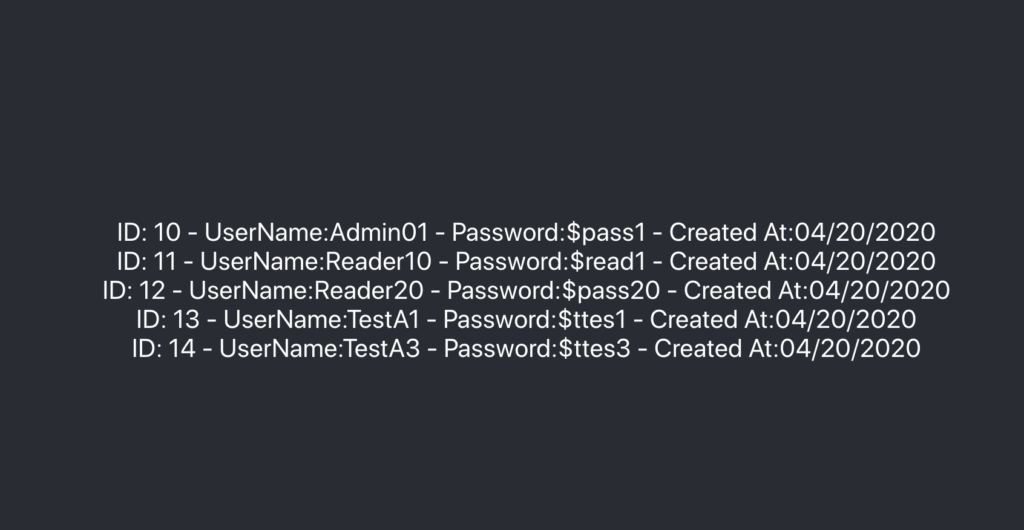
It works fine and now, we are going to do an optimization.
We create another component called itemuser, in order to display the object user:
[ITEMUSER.JS]
import React from 'react'
const ItemUser = (props) => {
return (
<li>
ID: {props.User.id} - UserName:{props.User.userName} - Password:{props.User.password} - Created At:{props.User.createdAt}
</li>
)
}
export default ItemUser
Finally, we add the component itemuser in the component listuser:
import React from 'react'
import ItemUser from './itemuser'
function ListUsers() {
const lstUsers = [
{
id:10,
userName: 'Admin01',
password: '$pass1',
createdAt: '04/20/2020'
},
{
id:11,
userName: 'Reader10',
password: '$read1',
createdAt: '04/20/2020'
},
{
id:12,
userName: 'Reader20',
password: '$pass20',
createdAt: '04/20/2020'
},
{
id:13,
userName: 'TestA1',
password: '$ttes1',
createdAt: '04/20/2020'
},
{
id:14,
userName: 'TestA3',
password: '$ttes3',
createdAt: '04/20/2020'
}
]
const result = lstUsers.map(User => (<ItemUser key={User.id} User={User}></ItemUser>))
return (
<ul>
{result}
</ul>
)
}
export default ListUsers
We have done and now, we can run the application to verify everything works fine again:
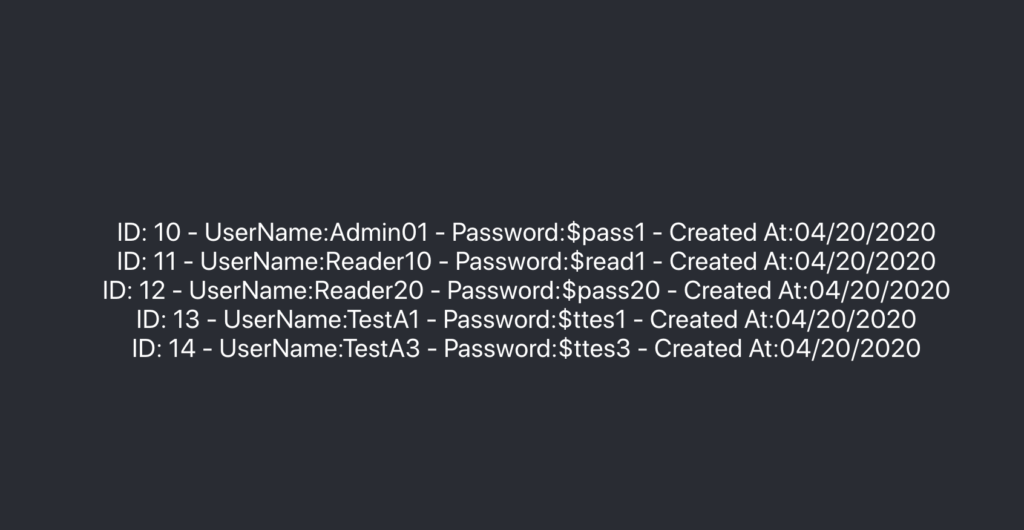