In this post, we will see how to render a list without an index in React.
First of all, we create a function component called listusersnoindex where, we will define an array of string:
[LISTUSERSNOINDEX.JS]
import React from 'react'
function ListUsersNoIndex() {
const lstUsers = ["User1", "User2", "User3", "User4"]
const result = lstUsers.map(Name => (<h3>{Name}</h3>))
return (
<div>
{result}
</div>
)
}
export default ListUsersNoIndex
Then, we modify the file App.js in order to add the component:
[APP.JS]
import React from 'react';
import './App.css';
import ListUsersNoIndex from './components/listusersnoindex';
function App() {
return (
<div className="App">
<header className="App-header">
<ListUsersNoIndex></ListUsersNoIndex>
</header>
</div>
);
}
export default App;
If we run the application, this will be the result:
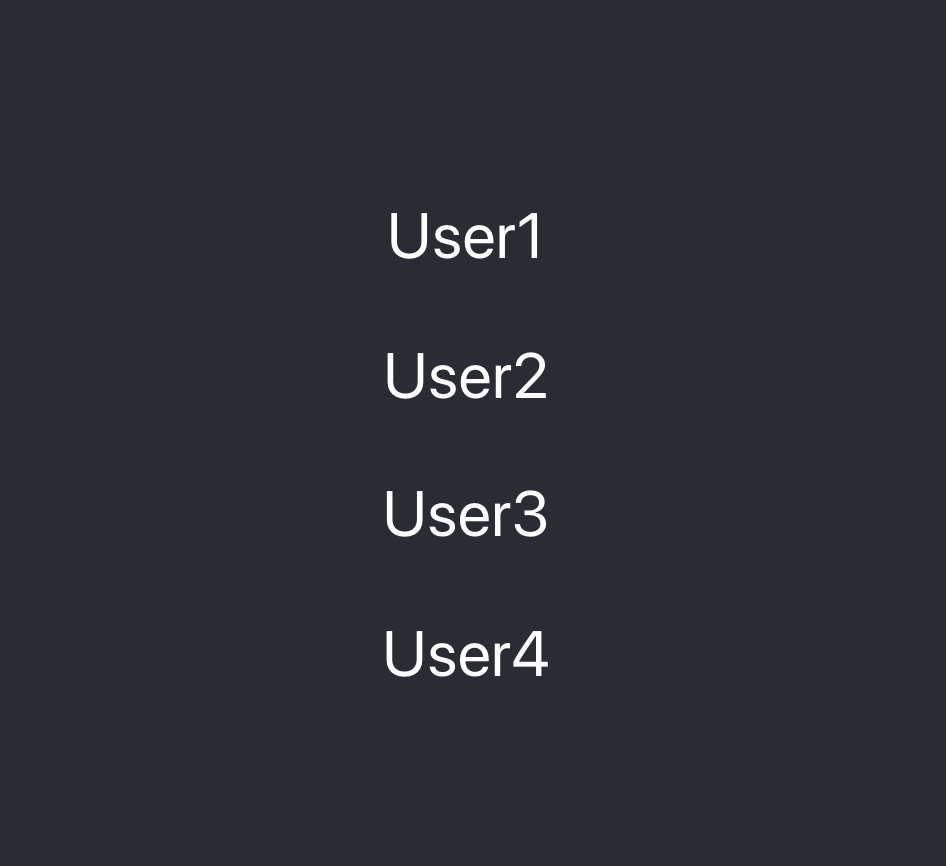
It seems works fine but, if we open the Developer Tools in Chrome, we will see this error:
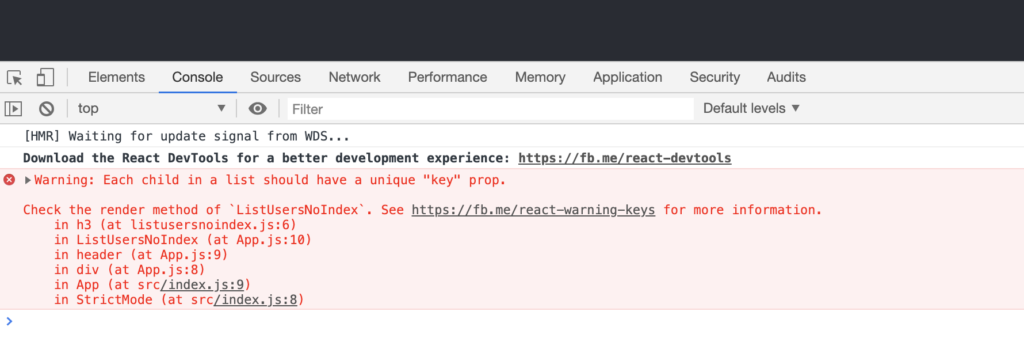
The message is quite clear: we need of a key in the list.
In order to fix the problem, we have to modify the file listusersnoindex:
import React from 'react'
function ListUsersNoIndex() {
const lstUsers = ["User1", "User2", "User3", "User4"]
const result = lstUsers.map((Name, index) => (<h3 key={index}>{Name}</h3>))
return (
<div>
{result}
</div>
)
}
export default ListUsersNoIndex
Now, if we run the application, this will be the result:
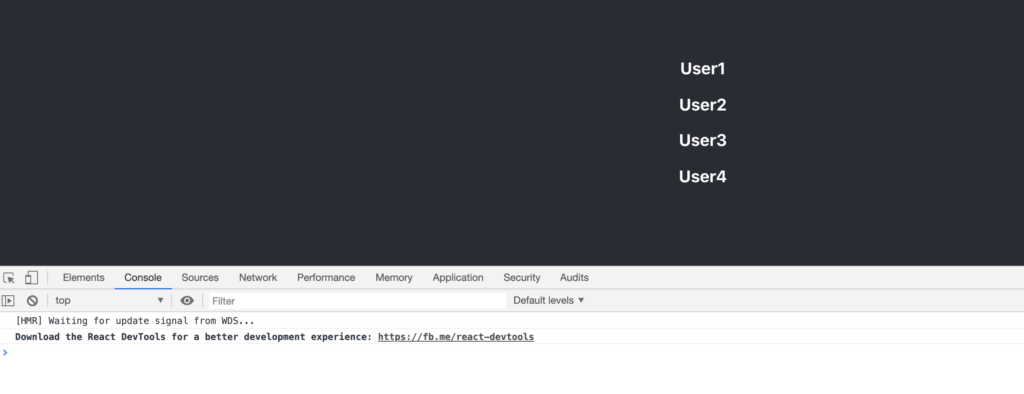