In this post, we will see how to fetch data in React using a library called Axios, modifying the application created in the post: React – Fetch data in Class component.
Axios is a promise based HTTP client for the browser and Node.js and, it makes easy to send asynchronous HTTP requests to REST endpoints and perform CRUD operations.
For knowing all Axios’ functionalities, go to the Official GitHub project.
First of all, we install the library Axios using the command npm I axios and then, we modify the component GetListUsers:
[GETLISTUSERS.JS]
import React, { Component } from 'react'
import ViewListUsers from './HTMLUserList';
import axios from 'axios';
// definition of the Web API's url
const APIUrl = "https://webapidocker.azurewebsites.net/users";
class GetListUsers extends Component {
// definition of variables
constructor() {
super();
this.state = {
lstUsers: [],
loading: false,
error: null,
};
}
// Definition of a method used to load data from service
// The method is called by the button "Load Data"
LoadData()
{
this.setState({loading:true});
axios.get(APIUrl)
.then(result => this.setState({
lstUsers: result.data,
loading: false
}))
.catch(error => this.setState({
error: error,
loading: false
}));
}
render() {
// Using the Destructuring assignment, we define three variables used in the component
const { lstUsers, loading, error } = this.state;
// in case of error, component will display an error message
if (error) {
return <h1 style = {{color:"white"}}>Attention! There are problems with the Web API.</h1>;
}
return (
<div>
<h1 style = {{color:"white"}}>List users</h1>
<br></br>
<button onClick={() => this.LoadData()}>Load Data</button>
{loading ? <h2 style = {{color:"white"}}>Loading...</h2>:
<ViewListUsers users={lstUsers}/>
}
</div>
)
}
}
export default GetListUsers
If we run the application, this will be the result:

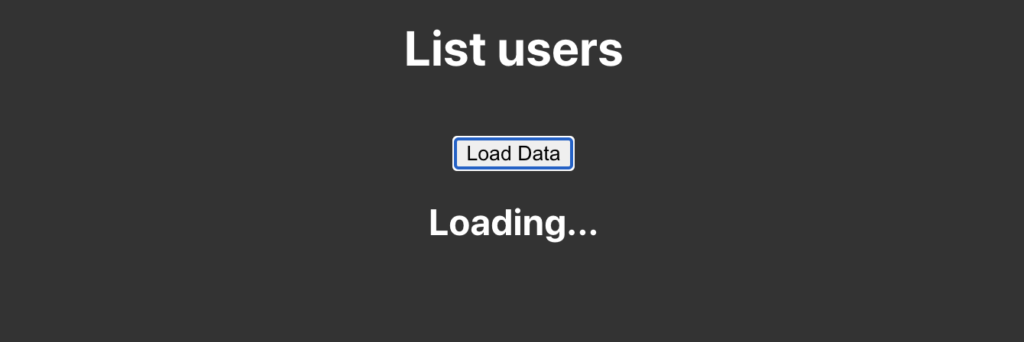
