In this post, we will see how to create a Splash Screen with SwiftUI.
First of all, we create a “Single View App” project:
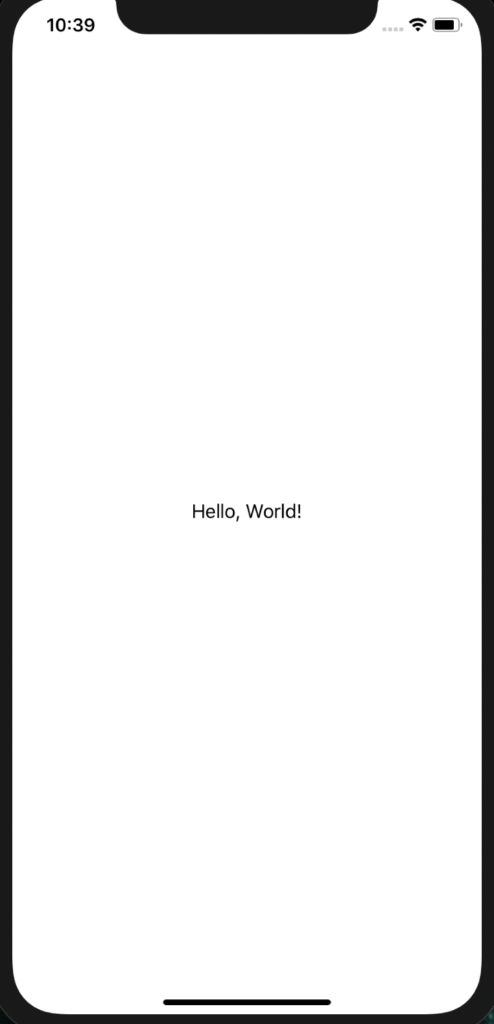
Then, we add a SwiftUI file called Page1 where we will define our principal view:
[PAGE1.SWIFT]
import SwiftUI
struct Page1: View {
var body: some View {
ZStack {
Color.yellow.edgesIgnoringSafeArea(.all)
VStack{
Text("PAGE 1")
.foregroundColor(Color.blue)
}
}
}
}
struct Page1_Previews: PreviewProvider {
static var previews: some View {
Page1()
}
}
The view is very easy and simple but, it isn’t the main goal of the post.
Finally, we will modify the file ContentView.swift (the default view) in order to insert a simple Splash Screen that, it will be visible for three seconds before showing Page1:
[CONTENTVIEW.SWIFT]
import SwiftUI
struct ContentView: View {
// definition of a variable called showMainView
@State var showMainView:Bool = false
var body: some View {
VStack{
if self.showMainView
{
// show the principal page
Page1()
}
else
{
// show the Splash Screen
Text("LOADING...")
.bold()
.foregroundColor(.blue)
.font(.system(size: 30))
}
}
.onAppear {
// after three seconds, system will change the value of the
// showMainView variable
DispatchQueue.main.asyncAfter(deadline: .now() + 3) {
withAnimation {
self.showMainView = true
}
}
}
}
}