In this post, we will see how to create a simple versioning of a Web API method, using the Routing attribute.
However, before starting, I want to remember that the best way for versioning a method it was describe here: Web API – Versioning
We create a Web API project called TestAPI and we add a class called User:
USER
using System;
namespace TestAPI
{
public class User
{
public Guid Id { get; set; }
public string UserName { get; set; }
}
}
Then, we define a controller called UserController:
USERCONTROLLER
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
namespace TestAPI.Controllers
{
[ApiController]
public class UserController : ControllerBase
{
private List<User> lstUser = new List<User>();
public UserController()
{
for(var i=1;i<10;i++)
{
lstUser.Add(new User { Id = Guid.NewGuid(), UserName=$"UserName_{i}" });
}
}
// Definition of version 1 of getall
[HttpGet("v1/getall")]
public IActionResult GetAll()
{
return Ok(lstUser);
}
}
}
We have done and now, if we call the method GetAll using Postman, this will be the result:

Now, we will define a second version of GetAll method, starting to create a new entity called User2:
USER2
using System;
namespace TestAPI
{
public class User2:User
{
public DateTime CreatedAt { get; set; }
}
}
Then, we modify the UserController, adding a new version of GetAll called GetAll2:
USERCONTROLLER
using Microsoft.AspNetCore.Mvc;
using System;
using System.Collections.Generic;
namespace TestAPI.Controllers
{
[ApiController]
public class UserController : ControllerBase
{
private List<User> lstUser = new List<User>();
private List<User2> lstUser2 = new List<User2>();
public UserController()
{
for(var i=1;i<10;i++)
{
lstUser.Add(new User { Id = Guid.NewGuid(), UserName=$"UserName_{i}" });
lstUser2.Add(new User2 { Id = Guid.NewGuid(), UserName = $"UserName_{i+10}", CreatedAt= DateTime.Now.AddDays(-i) });
}
}
// Definition of version 1 of getall
[HttpGet("v1/getall")]
public IActionResult GetAll()
{
return Ok(lstUser);
}
// Definition of version 2 of getall
[HttpGet("v2/getall")]
public IActionResult GetAll2()
{
return Ok(lstUser2);
}
}
}
We have done and now, if we call the method GetAll (v2) using Postman, this will be the result:
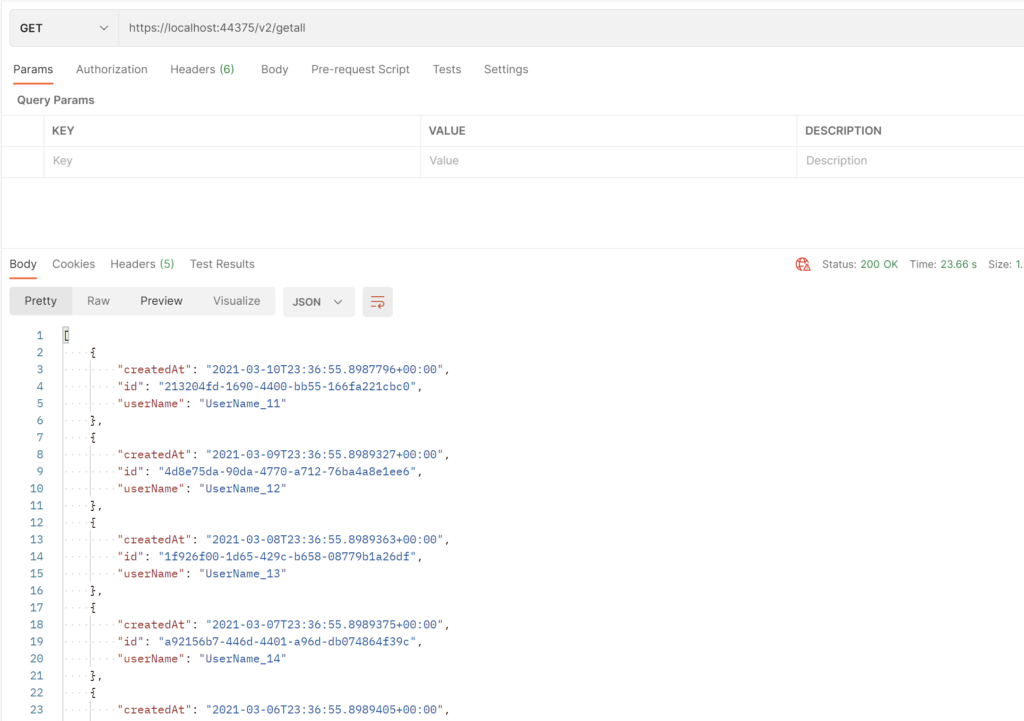