In this post, we will see how to use IHttpClientFactory in a console application, in order to call a WebAPI service that gives us a list of Users like this:
[
{
"id": 1,
"username": "username1",
"password": "password1",
"email": "user1@test.com"
},
{
"id": 2,
"username": "username2",
"password": "password2",
"email": "user2@test.com"
},
...
...
...
]
The url of this WebAPI is http://localhost:3000/users and I have created this service using Json Server
First of all, we create a Console application project called MockWEBAPI and we start to define a class called User:
[USERS.CS]
namespace MockWEBAPI
{
public class User
{
public int Id { get; set; }
public string Username { get; set; }
public string Password { get; set; }
public string Email { get; set; }
}
}
Now, we create an Interface called IServiceManagement where we will define the method used to call the service:
[ISERVICEMANAGEMENT.CS]
using System.Collections.Generic;
using System.Threading.Tasks;
namespace MockWEBAPI
{
public interface IServiceManagement
{
Task<List<User>> GetAllUsers();
}
}
Then, we define a class called ServiceManagement, where we will implement the IServiceManagement interface:
[SERVICEMANAGEMENT.CS]
using System.Collections.Generic;
using System.Net.Http;
using System.Text.Json;
using System.Threading.Tasks;
namespace MockWEBAPI
{
public class ServiceManagement:IServiceManagement
{
private readonly IHttpClientFactory _clientFactory;
public ServiceManagement(IHttpClientFactory clientFactory)
{
_clientFactory = clientFactory;
}
public async Task<List<User>> GetAllUsers()
{
List<User> lstUsers = null;
// Call the method Get
var responseService = await _clientFactory.CreateClient().GetAsync("http://localhost:3000/users");
// Check the response
if (responseService.IsSuccessStatusCode)
{
// Read the list of Users
var outputService = await responseService.Content.ReadAsByteArrayAsync();
var options = new JsonSerializerOptions() { PropertyNameCaseInsensitive = true };
try
{
// Deserialization of the output
lstUsers = JsonSerializer.Deserialize<List<User>>(outputService, options);
}
catch
{
throw;
}
}
return lstUsers;
}
}
}
Finally, we define the class Program:
[PROGRAM.CS]
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
using System;
using System.Net.Http;
using System.Threading.Tasks;
namespace MockWEBAPI
{
class Program
{
static async Task Main(string[] args)
{
var builder = new HostBuilder()
.ConfigureServices((hostContext, services) =>
{
// with AddHttpClient we register the IHttpClientFactory
services.AddHttpClient();
// here, we register the dependency injection
services.AddTransient<IServiceManagement, ServiceManagement>();
}).UseConsoleLifetime();
var host = builder.Build();
try
{
// here we 'get' IServiceManagement
var myService = host.Services.GetRequiredService<IServiceManagement>();
// we run the method GetAllUsers
var lstUsers = await myService.GetAllUsers();
foreach(var item in lstUsers)
{
Console.WriteLine($"{item.Id} - {item.Username} - {item.Password} - {item.Email}");
}
}
catch (Exception ex)
{
var logger = host.Services.GetRequiredService<ILogger<Program>>();
logger.LogError(ex, "An error occurred.");
}
}
}
}
We have done and now, if we run the application this will be the result:
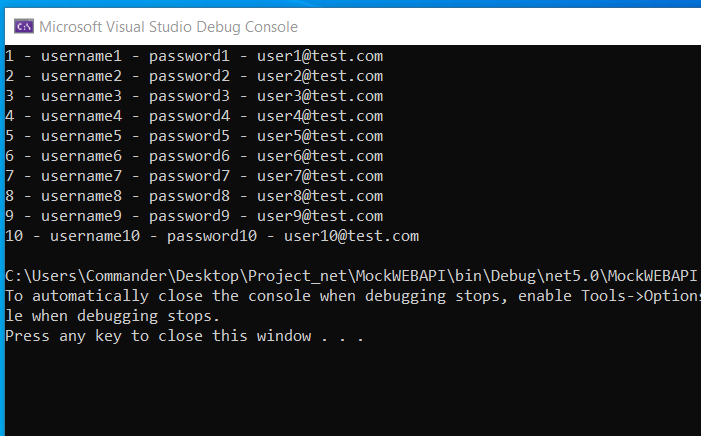