In this post, we will see how to create a WebAPI project to execute CRUD operations in a MongoDB database.
We start creating a Docker-compose file in order to define a MongoDB container and a Mongo-Express container:
[DOCKER-COMPOSE.YML]
version: '3'
services:
dockermongo:
image: mongo
environment:
- MONGO_INITDB_ROOT_USERNAME=admindb
- MONGO_INITDB_ROOT_PASSWORD=pass123
volumes:
- dbmongo:/data/db
networks:
- netMongoDBServer
ports:
- 27017:27017
dockermongoexpress:
image: mongo-express
restart: always
ports:
- 8081:8081
environment:
- ME_CONFIG_MONGODB_ADMINUSERNAME=admindb
- ME_CONFIG_MONGODB_ADMINPASSWORD=pass123
- ME_CONFIG_MONGODB_SERVER=dockermongo
networks:
- netMongoDBServer
volumes:
dbmongo:
driver: local
networks:
netMongoDBServer:
driver: bridge
Then, with the command docker-compose up -d, we run the docker-compose file:

Now, we will add a database called dbuser into our MongoDB instance and then, we will add a collection called users:
execution of bash command inside the container
docker exec -it composerfiles_dockermongo_1 bash
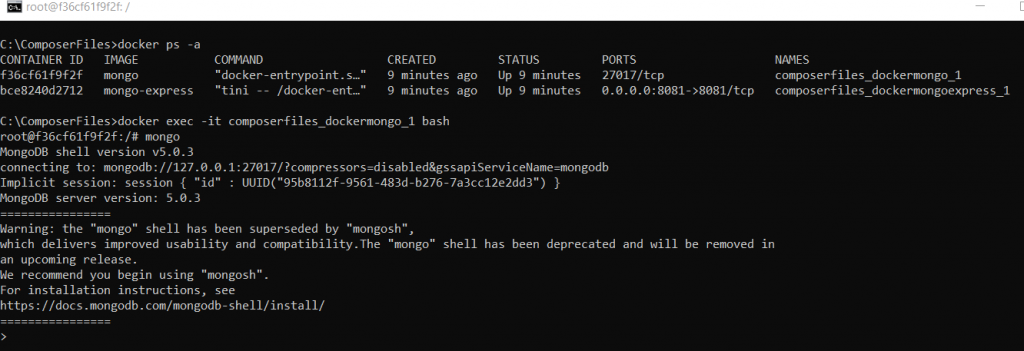
login in MongoDB
use admin
db.auth(“admindb”, passwordPrompt())
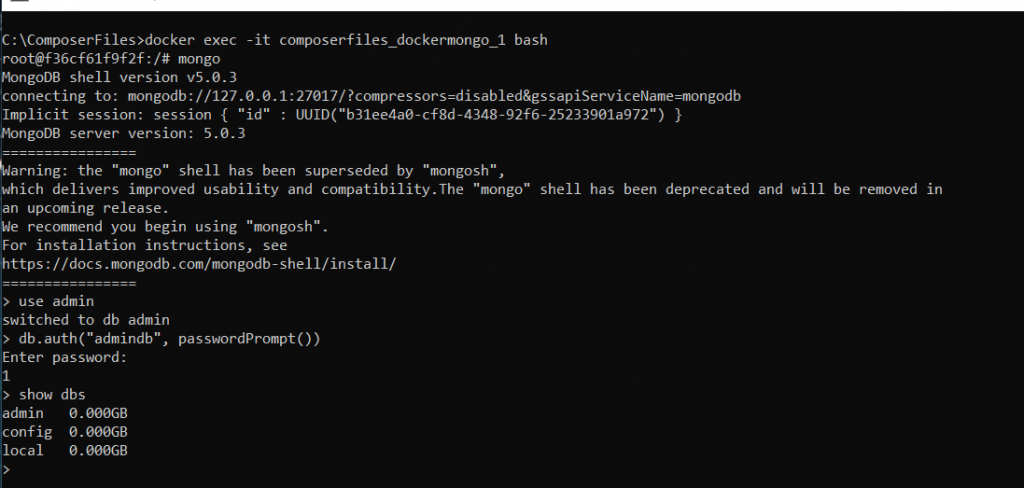
creation of a db called dbuser
use dbuser

creation of a collection called users
db.createCollection("users")

We have done and now, we will create a WebAPI project called MongoService where we will define the methods to execute CRUD operations:
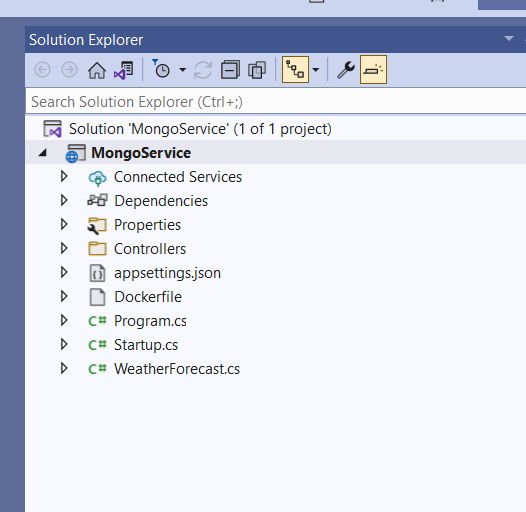
First of all, we add the Mongo driver library using the command:
Install-Package MongoDB.Driver -Version 2.14.1
Then, we define an entity called User:
[USER.CS]
using MongoDB.Bson;
using MongoDB.Bson.Serialization.Attributes;
using System;
namespace MongoService.Model.Entities
{
public class User
{
// With BsonId we define this property as primary key
[BsonId]
// With BsonRepresentation we will save this property as ObjectId
[BsonRepresentation(BsonType.ObjectId)]
public string Id { get; set; }
public string Username { get; set; }
public string Password { get; set; }
// With BsonIgnore this property will not save in the Mongo document
[BsonIgnore]
public bool Test { get; set; }
}
}
Now, we will add some MongoDB settings in the appsettings file and we will read them in the method ConfigureServices of Startup file:
[APPSETTINGS.JSON]
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"MongoConnect": {
"UserCollectionName": "users",
"ConnectionString": "mongodb://admindb:pass123@localhost:27017",
"DatabaseName": "dbuser"
}
}
[IMONGOCONNECT.CS]
namespace MongoService.Model.MongoConnect
{
public interface IMongoConnect
{
string UserCollectionName { get; set; }
string ConnectionString { get; set; }
string DatabaseName { get; set; }
}
}
[MONGOCONNECT.CS]
namespace MongoService.Model.MongoConnect
{
public class MongoConnect : IMongoConnect
{
public string UserCollectionName { get; set; }
public string ConnectionString { get; set; }
public string DatabaseName { get; set; }
}
}
[STARTUP.CS]
public void ConfigureServices(IServiceCollection services)
{
services.Configure<MongoConnect>(
Configuration.GetSection(nameof(MongoConnect)));
services.AddSingleton<IMongoConnect>(sp =>
sp.GetRequiredService<IOptions<MongoConnect>>().Value);
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "MongoService", Version = "v1" });
});
}
Then, we define a service layer called UserService to manage CRUD operation in MongoDB:
[USERSERVICE.CS]
using MongoDB.Driver;
using MongoService.Model.Entities;
using MongoService.Model.MongoConnect;
using System.Collections.Generic;
namespace MongoService.Service
{
public class UserService
{
// definition of User collection
private readonly IMongoCollection<User> _users;
public UserService(IMongoConnect settings)
{
// definition MongoDB client
var client = new MongoClient(settings.ConnectionString);
// get MongoDB database
var database = client.GetDatabase(settings.DatabaseName);
// feed the collection
_users = database.GetCollection<User>(settings.UserCollectionName);
}
public List<User> GetAllUsers() =>
_users.Find(user => true).ToList();
public User GetUserById(string id) =>
_users.Find<User>(user => user.Id == id).FirstOrDefault();
public User AddUser(User user)
{
_users.InsertOne(user);
return user;
}
public void UpdateUser(string id, User userInput) =>
_users.ReplaceOne(user => user.Id == id, userInput);
public void RemoveUser(string id) =>
_users.DeleteOne(user => user.Id == id);
}
}
[STARTUP.CS]
public void ConfigureServices(IServiceCollection services)
{
services.Configure<MongoConnect>(
Configuration.GetSection(nameof(MongoConnect)));
services.AddSingleton<IMongoConnect>(sp =>
sp.GetRequiredService<IOptions<MongoConnect>>().Value);
services.AddSingleton<UserService>();
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "MongoService", Version = "v1" });
});
}
Finally, we create a controller called UserController:
[USERCONTROLLER.CS]
using Microsoft.AspNetCore.Mvc;
using MongoService.Model.Entities;
using MongoService.Service;
using System.Collections.Generic;
namespace MongoService.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class UserController : ControllerBase
{
private readonly UserService _userService;
public UserController(UserService userService)
{
_userService = userService;
}
[HttpGet]
public ActionResult<List<User>> GetAllUser() => _userService.GetAllUsers();
[HttpGet("{id}", Name = "GetUser")]
public ActionResult<User> GetUserById(string id)
{
var user = _userService.GetUserById(id);
if (user == null)
{
return NotFound();
}
return user;
}
[HttpPost]
public ActionResult<User> AddUser(User user)
{
_userService.AddUser(user);
return CreatedAtRoute("GetUser", new { id = user.Id }, user);
}
[HttpPut("{id}")]
public IActionResult UpdateUser(string id, User userInput)
{
var user = _userService.GetUserById(id);
if (user == null)
{
return NotFound();
}
_userService.UpdateUser(id, userInput);
return NoContent();
}
[HttpDelete("{id}")]
public IActionResult DeleteUser(string id)
{
var user = _userService.GetUserById(id);
if (user == null)
{
return NotFound();
}
_userService.RemoveUser(user.Id);
return NoContent();
}
}
}
We have done and now, if we run the service, these will be the results:
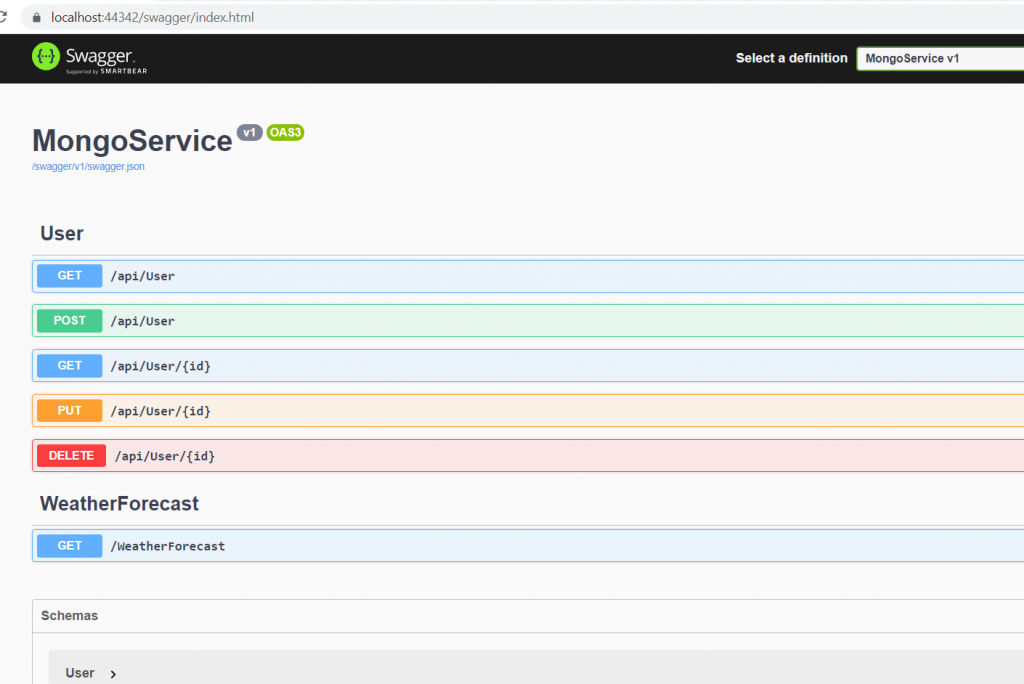
GET ALL USERS

GET USER BY ID
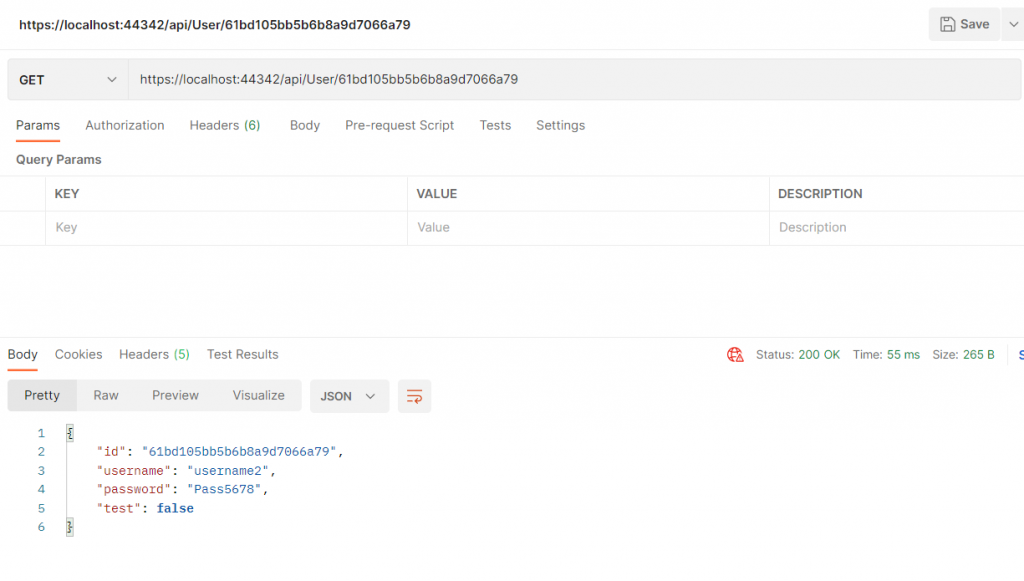
ADD USER
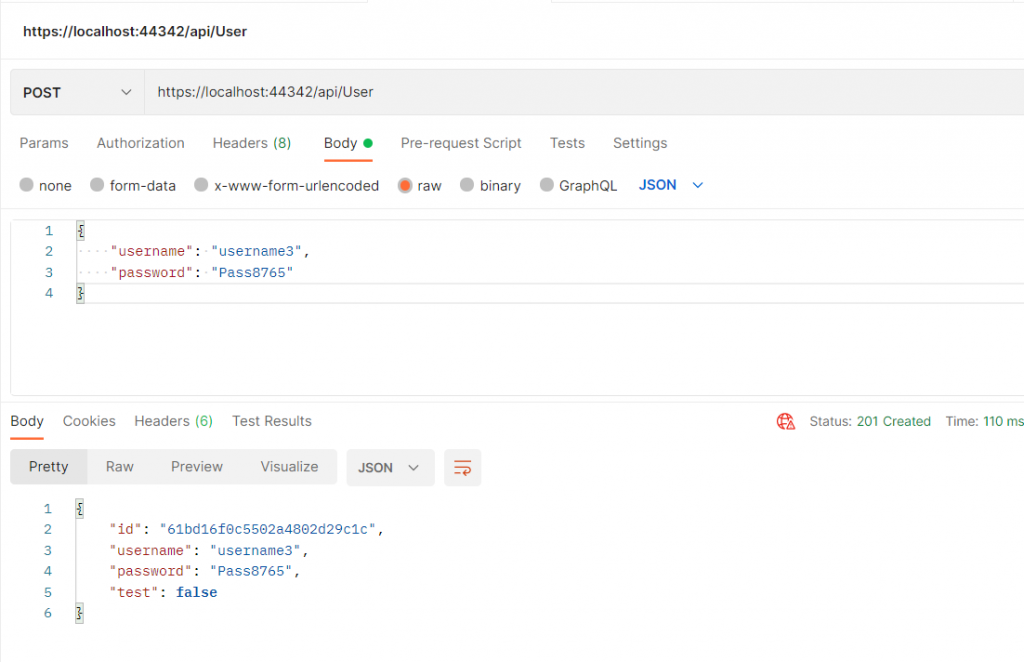

UPDATE USER
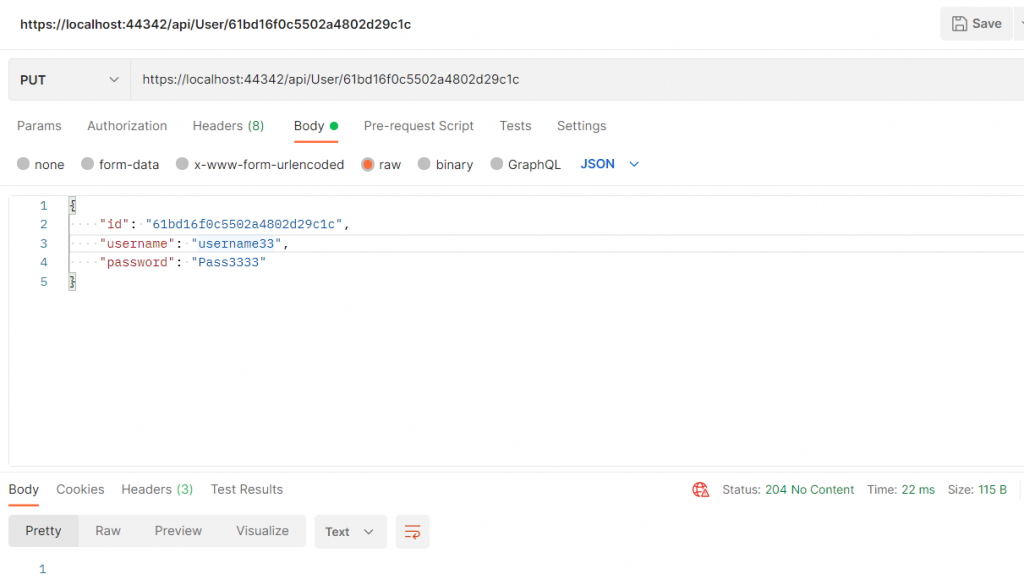
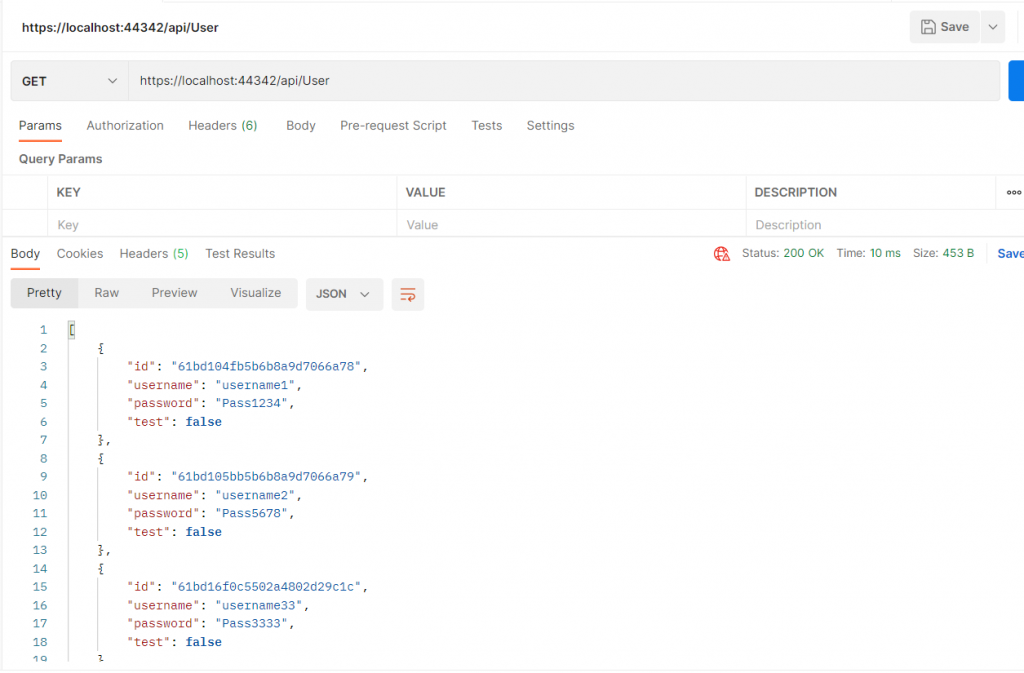
DELETE USER
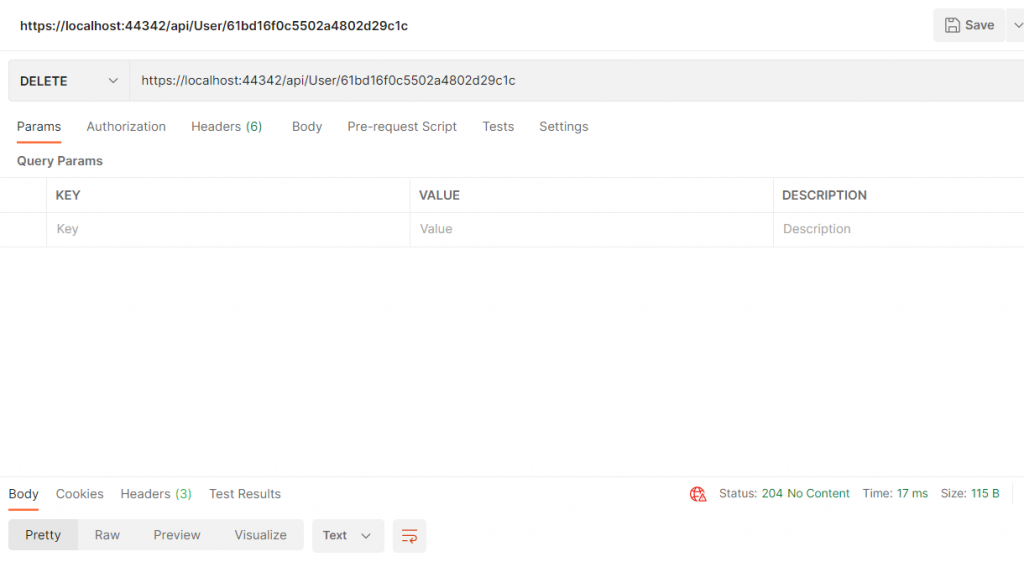
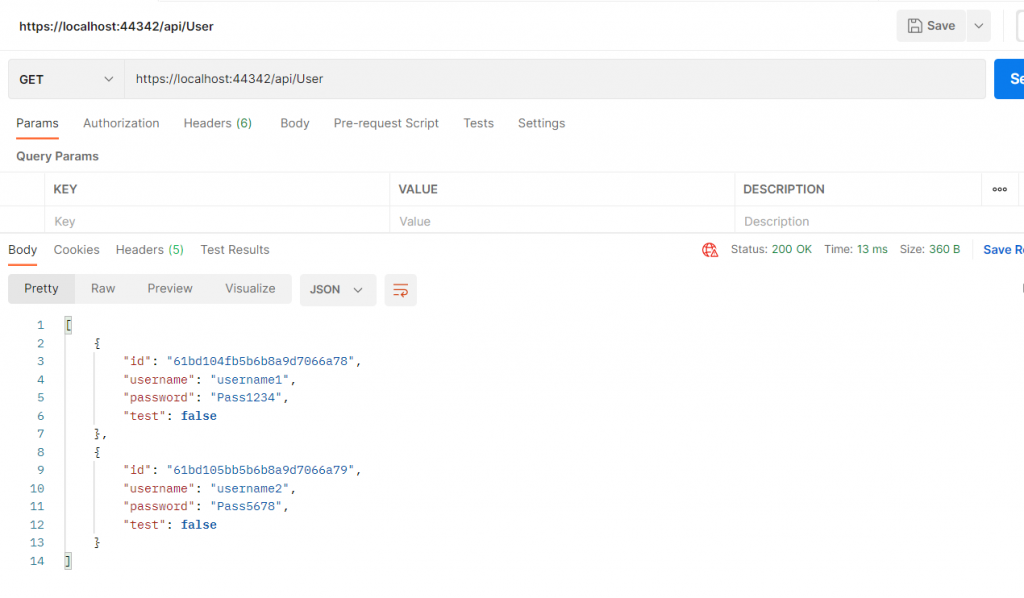
MONGOSERVICE – CODE
https://github.com/ZoneOfDevelopment/MongoService