In this post, we will see how to create a Minimal APIs with .net Core.
But first of all, what is a Minimal APIs?
From Microsoft web site:
“Minimal APIs are architected to create HTTP APIs with minimal dependencies. They are ideal for microservices and apps that want to include only the minimum files, features, and dependencies in ASP.NET Core.”
In a nutshell, we can use Minimal APIs when we need to create simple services with less complexity, layers and classes.
For this post, we will develop a Minimal APIs for managing the CRUD operations of an entity called Dog.
We start opening VS and we create an ASP.NET Core Web API project called MinimalAPI with this properties:
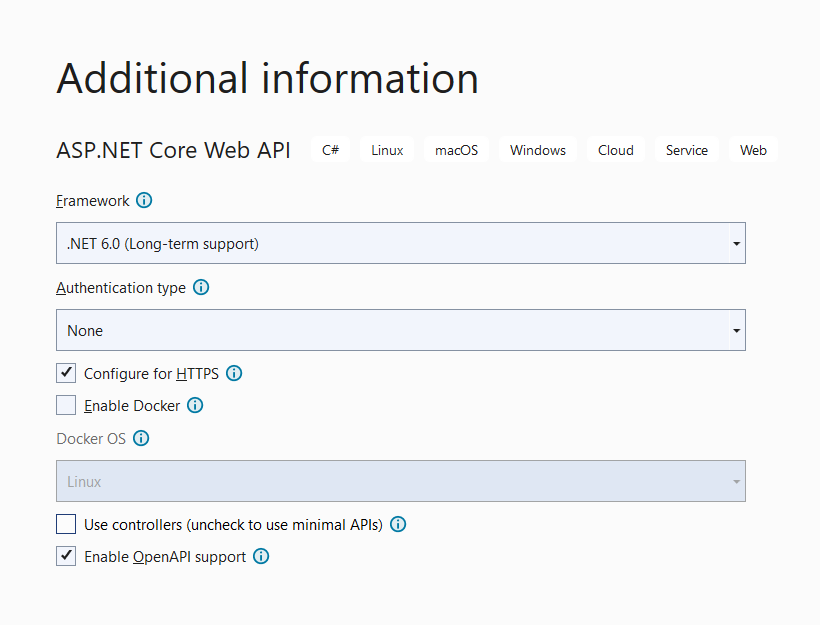
Then, we will install the “Microsoft Entity FrameworkCore InMemory” library, running from the Package Manager Console the command:
Install-Package Microsoft.EntityFrameworkCore.InMemory
Now, we will create the Business Layer to manage CRUD operations:
[DOG.CS]
namespace MinimalAPI.Model
{
public class Dog
{
public Guid Id { get; set; }
public string Name { get; set; }
public string Breed { get; set; }
public string Color { get; set; }
}
}
[DATACONTEXT.CS]
using Microsoft.EntityFrameworkCore;
namespace MinimalAPI.Model
{
public class DataContext: DbContext
{
public DataContext(DbContextOptions<DataContext> options)
: base(options) { }
public DbSet<Dog> Dogs => Set<Dog>();
}
}
[IDOGCOMMANDS.CS]
using MinimalAPI.Model;
namespace MinimalAPI.Commands
{
public interface IDogCommands
{
Task<bool> AddDog(Dog dog);
Task<List<Dog>> GetAllDogs();
Task<Dog> GetDogById(Guid id);
Task<bool> UpdateDog(Dog dog, Guid id);
Task<bool> DeleteDog(Guid id);
Task Save();
}
}
[DOGCOMMANDS.CS]
using Microsoft.EntityFrameworkCore;
using MinimalAPI.Model;
namespace MinimalAPI.Commands
{
public class DogCommands:IDogCommands
{
private readonly DataContext _dataContext;
public DogCommands(DataContext dataContext)
{
_dataContext = dataContext;
}
public async Task<bool> AddDog(Dog dog)
{
try
{
await _dataContext.Dogs.AddAsync(dog);
return true;
}
catch (Exception)
{
return false;
}
}
public async Task<List<Dog>> GetAllDogs()
{
return await _dataContext.Dogs.AsNoTracking().ToListAsync();
}
public async Task<Dog> GetDogById(Guid id)
{
return await _dataContext.Dogs.FindAsync(id);
}
public async Task<bool> UpdateDog(Dog dog, Guid id)
{
var dogInput = await _dataContext.Dogs.FindAsync(id);
if(dogInput == null)
{
return false;
}
dogInput.Name = dog.Name;
dogInput.Color = dog.Color;
dogInput.Breed = dog.Breed;
await _dataContext.SaveChangesAsync();
return true;
}
public async Task<bool> DeleteDog(Guid id)
{
var dogInput = await _dataContext.Dogs.FindAsync(id);
if (dogInput == null)
{
return false;
}
_dataContext.Dogs.Remove(dogInput);
return true;
}
public async Task Save()
{
await _dataContext.SaveChangesAsync();
}
}
}
Finally, we modify Program.cs where we will add the API code:
[PROGRAM.CS]
using Microsoft.EntityFrameworkCore;
using MinimalAPI.Commands;
using MinimalAPI.Model;
var builder = WebApplication.CreateBuilder(args);
// definition of DataContext
builder.Services.AddDbContext<DataContext>(opt => opt.UseInMemoryDatabase("DbDog"));
// definition of Dependency Injection
builder.Services.AddScoped<IDogCommands, DogCommands>();
// Add services to the container.
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
// Definition Get Method
app.MapGet("/dog", async (IDogCommands commands) =>
await commands.GetAllDogs());
// Definition Get{Id} Method
app.MapGet("/dog/{id}", async (Guid id, IDogCommands commands) =>
{
var dog = await commands.GetDogById(id);
if (dog == null) return Results.NotFound();
return Results.Ok(dog);
});
// Definition Post Method
app.MapPost("/dog", async (Dog dog, IDogCommands commands) =>
{
await commands.AddDog(dog);
await commands.Save();
return Results.Ok();
});
// Definition Put Method
app.MapPut("/dog/{id}", async (Guid id, Dog dog, IDogCommands commands) =>
{
var updateOk = await commands.UpdateDog(dog, id);
if (!updateOk) return Results.NotFound();
return Results.NoContent();
});
// Definition Delete Method
app.MapDelete("/dog/{id}", async (Guid id, IDogCommands commands) =>
{
var deleteOk = await commands.DeleteDog(id);
if (deleteOk)
{
await commands.Save();
return Results.Ok();
}
return Results.NotFound();
});
app.Run();
We have done and now, if we run the application, this will be the result:
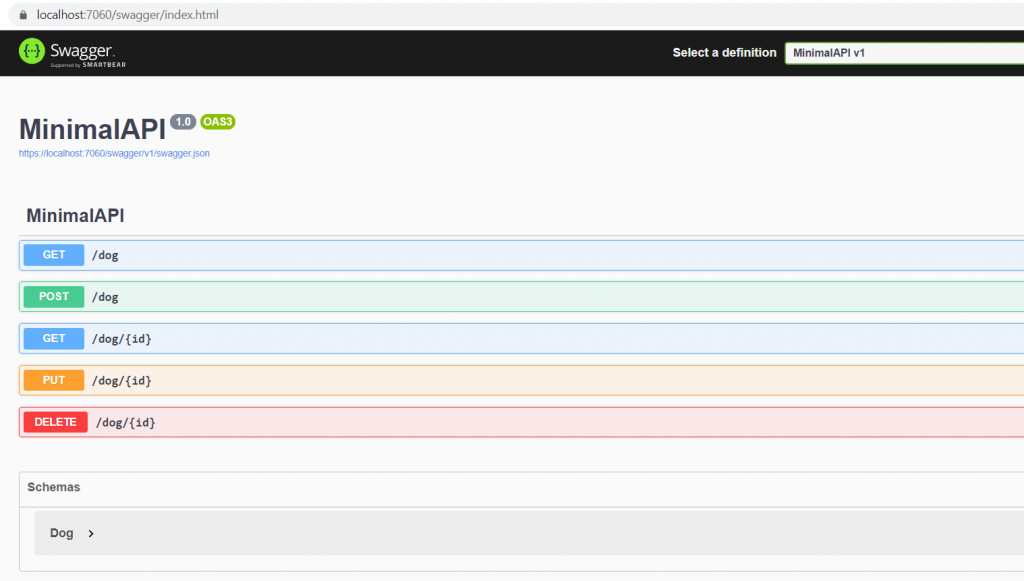
ADD DOG
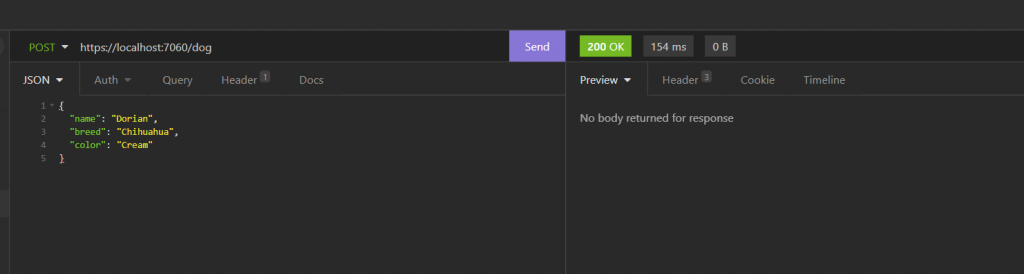
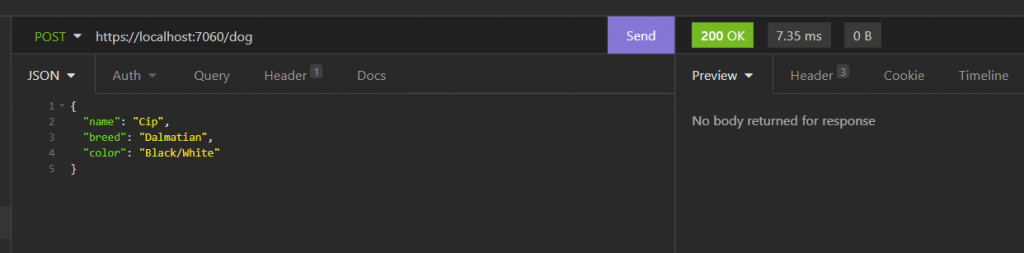
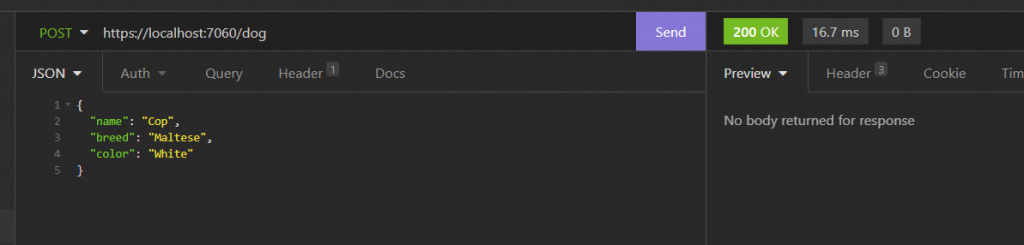
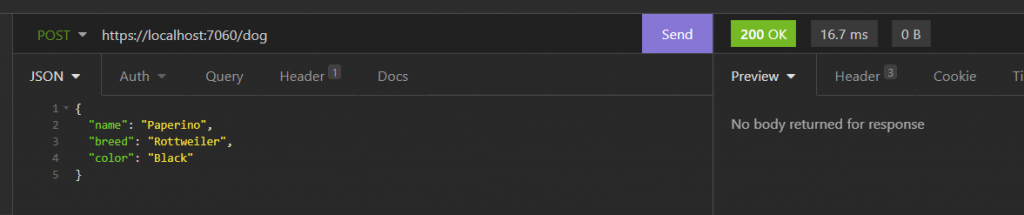
GET ALL DOGS
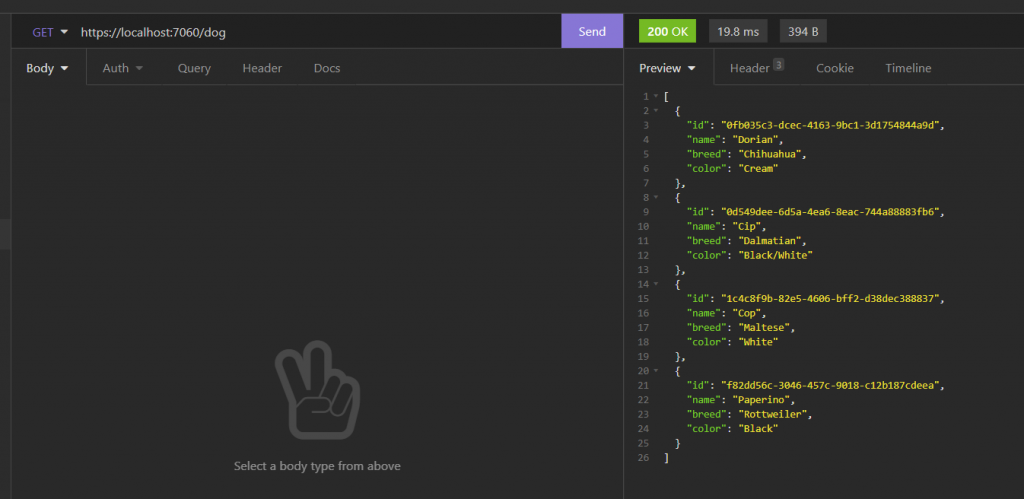
UPDATE DOG

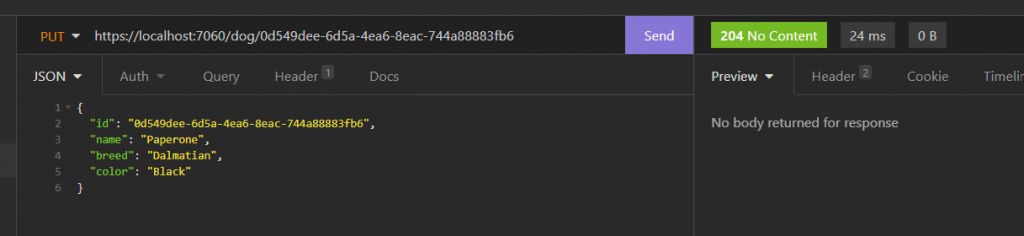
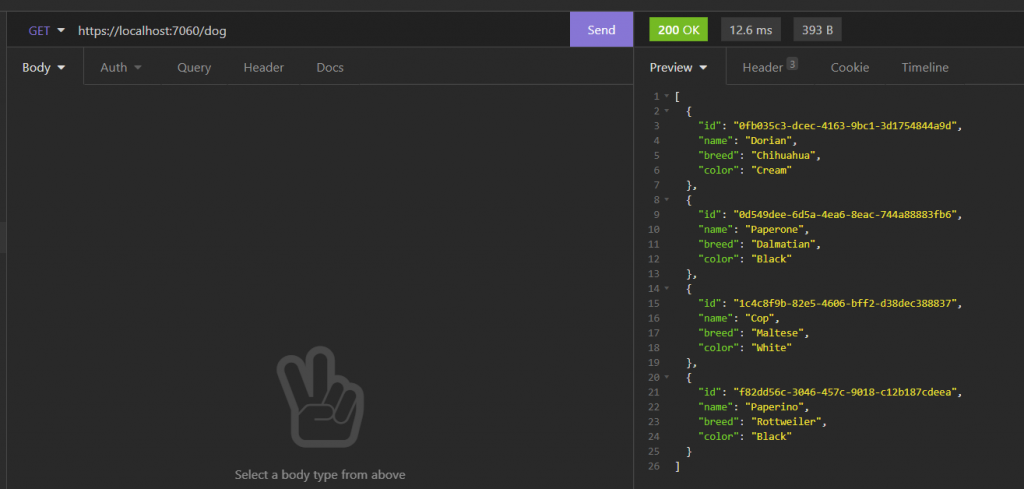
DELETE DOG

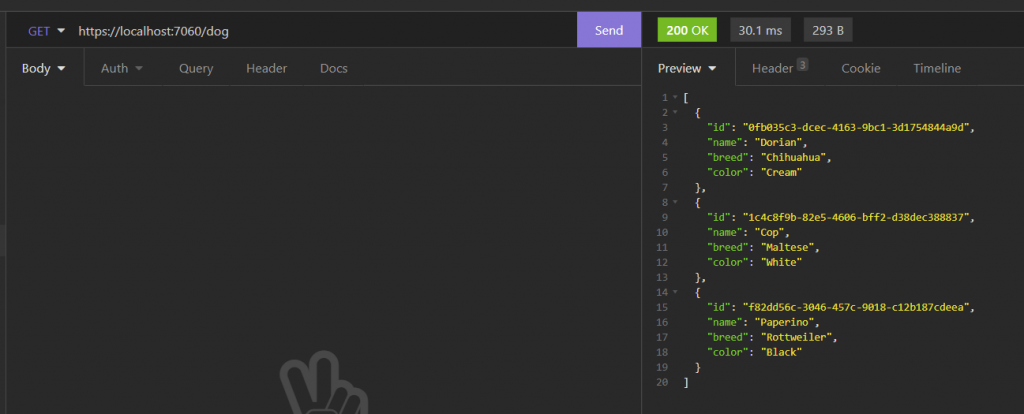
MINIMALAPI – CODE
https://github.com/ZoneOfDevelopment/MINIMALAPI