In this post, we will see how to read values, change values, delete keys and add new keys in a JSON file.
First of all, we define a JSON file like this:
[USER.JSON]
{
"Name":"Damiano",
"Surname": "Abballe",
"Telephones": ["1234567", "76544578"],
"InfoUser":{
"UserName": "UsernameDamiano",
"Password": "Password123",
"UserRoles":[
{
"Id": 1,
"Info": "Admin"
},
{
"Id": 2,
"Info": "Reader"
}
]
}
}
Then, we open Visual Studio Code and we create a file called readJson.py that we will use to manage the JSON file:
READ FILE:
# We import this module to read JSON file
import json
# Open the JSON file
with open('user.json') as fileJson:
# Read and Parse the JSON file
data = json.load(fileJson)
# Reading the values
print(f"Name -> {data['Name']}")
print(f"Surname -> {data['Surname']}")
# Reading the phone numbers
print("List of Telephones:")
phone_numbers = data['Telephones']
for number in phone_numbers:
print(number)
# Reading the values in the InfoUser section
print(f"Username -> {data['InfoUser']['UserName']}")
print(f"Password -> {data['InfoUser']['Password']}")
# Reading the roles in the UserRoles list
print("List of roles:")
roles = data['InfoUser']['UserRoles']
for role in roles:
print(f"{role['Id']} -> {role['Info']}")
# Close the file
# In theory, we don't need to close the file because the
# 'with' statement automatically closes the file when the
# block of code under the statement is completed
fileJson.close()
If we run the file, the following will be the result:
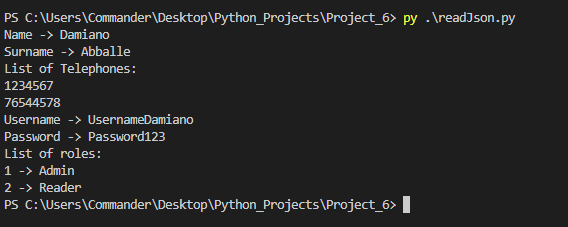
UPDATE VALUES:
# We import this module to read JSON file
import json
def readFile():
# Open the JSON file
with open('user.json') as fileJson:
# Read and Parse the JSON file
data = json.load(fileJson)
# Reading the values
print(f"Name -> {data['Name']}")
print(f"Surname -> {data['Surname']}")
# Reading the phone numbers
print("List of Telephones:")
phone_numbers = data['Telephones']
for number in phone_numbers:
print(number)
# Reading the values in the InfoUser section
print(f"Username -> {data['InfoUser']['UserName']}")
print(f"Password -> {data['InfoUser']['Password']}")
# Reading the roles in the UserRoles list
print("List of roles:")
roles = data['InfoUser']['UserRoles']
for role in roles:
print(f"{role['Id']} -> {role['Info']}")
# Close the file
# In theory, we don't need to close the file because the
# 'with' statement automatically closes the file when the
# block of code under the statement is completed
fileJson.close()
# Print the old file
print("Old JSON file")
readFile()
# Open the JSON file and load its contents into a variable
with open('user.json') as fileJson:
data = json.load(fileJson)
# Change the value of Telephones
data['Telephones'] = ['999999999', '88888888', '1111111']
# Change the value of Password
data['InfoUser']['Password'] = "NewPassword1"
# Save the updated values to the JSON file
with open('user.json', 'w') as updateFile:
json.dump(data, updateFile, indent=4)
fileJson.close()
updateFile.close()
# Print the new file
print()
print()
print("New JSON file")
readFile()
If we run the file, the following will be the result:
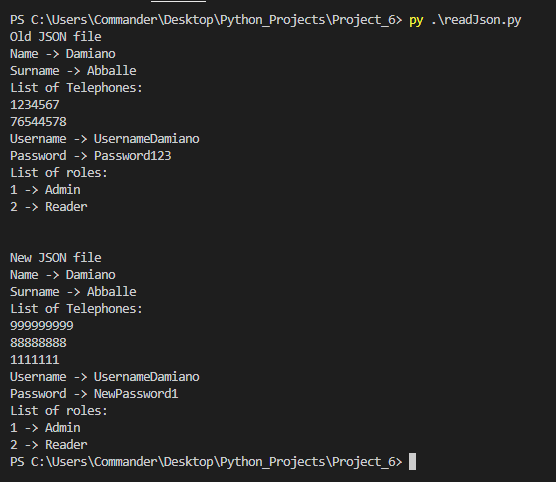
DELETE KEYS AND VALUES
Now, we will modify the file readJosn.py in order to delete the key “Telephones” and delete the first element in “UserRoles”:
# We import this module to read JSON file
import json
def readFile():
# Open the JSON file
with open('user.json') as fileJson:
# Read and Parse the JSON file
data = json.load(fileJson)
# Reading the values
print(f"Name -> {data['Name']}")
print(f"Surname -> {data['Surname']}")
# Check if Telephones key is present
if 'Telephones' in data:
# Reading the phone numbers
print("List of Telephones:")
phone_numbers = data['Telephones']
for number in phone_numbers:
print(number)
# Reading the values in the InfoUser section
print(f"Username -> {data['InfoUser']['UserName']}")
print(f"Password -> {data['InfoUser']['Password']}")
# Reading the roles in the UserRoles list
print("List of roles:")
roles = data['InfoUser']['UserRoles']
for role in roles:
print(f"{role['Id']} -> {role['Info']}")
# Close the file
# In theory, we don't need to close the file because the
# 'with' statement automatically closes the file when the
# block of code under the statement is completed
fileJson.close()
# Print the old file
print("Old JSON file")
readFile()
# Open the JSON file and load its contents into a variable
with open('user.json') as fileJson:
data = json.load(fileJson)
# Delete the Telephones key and its value
del data['Telephones']
# Delete the first element in the UserRoles list
del data['InfoUser']['UserRoles'][0]
# Save the updated values to the JSON file
with open('user.json', 'w') as updateFile:
json.dump(data, updateFile, indent=4)
fileJson.close()
updateFile.close()
# Print the new file
print()
print()
print("New JSON file")
readFile()
If we run the file, the following will be the result:
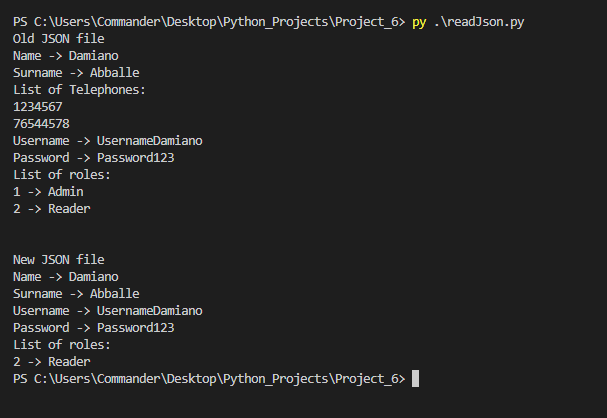
ADD A NEW KEY
Finally, we will modify the file readJosn.py in order to add a new key called “Address”:
# We import this module to read JSON file
import json
def readFile():
# Open the JSON file
with open('user.json') as fileJson:
# Read and Parse the JSON file
data = json.load(fileJson)
# Reading the values
print(f"Name -> {data['Name']}")
print(f"Surname -> {data['Surname']}")
# Check if Address key is present
if 'Address' in data:
print(f"Address -> {data['Address']}")
# Reading the phone numbers
print("List of Telephones:")
phone_numbers = data['Telephones']
for number in phone_numbers:
print(number)
# Reading the values in the InfoUser section
print(f"Username -> {data['InfoUser']['UserName']}")
print(f"Password -> {data['InfoUser']['Password']}")
# Reading the roles in the UserRoles list
print("List of roles:")
roles = data['InfoUser']['UserRoles']
for role in roles:
print(f"{role['Id']} -> {role['Info']}")
# Close the file
# In theory, we don't need to close the file because the
# 'with' statement automatically closes the file when the
# block of code under the statement is completed
fileJson.close()
# Print the old file
print("Old JSON file")
readFile()
# Open the JSON file and load its contents into a variable
with open('user.json') as fileJson:
data = json.load(fileJson)
# Add a new Key called Address
data['Address'] = '123 Main Streat'
# Save the updated values to the JSON file
with open('user.json', 'w') as updateFile:
json.dump(data, updateFile, indent=4)
fileJson.close()
updateFile.close()
# Print the new file
print()
print()
print("New JSON file")
readFile()
If we run the file, the following will be the result:
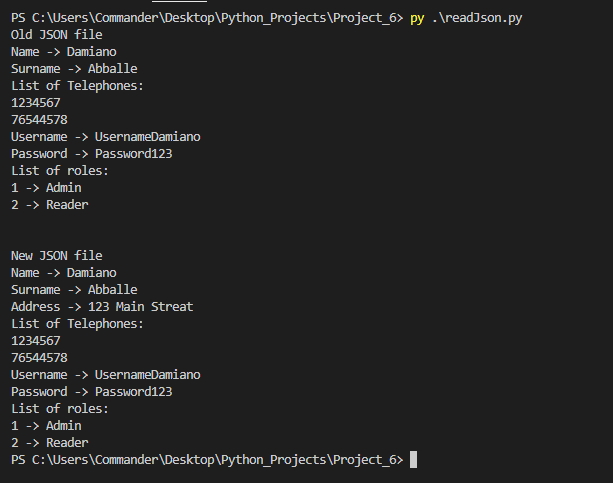