In this post, we will see some scripts that we can use to management files and directories.
CURRENT WORKING DIRECTORY:
import os
# Get the current working directory
current_dir = os.getcwd()
print(current_dir)
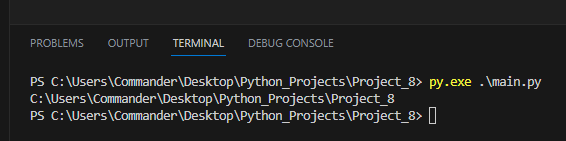
LIST DIRECTORY CONTENTS:
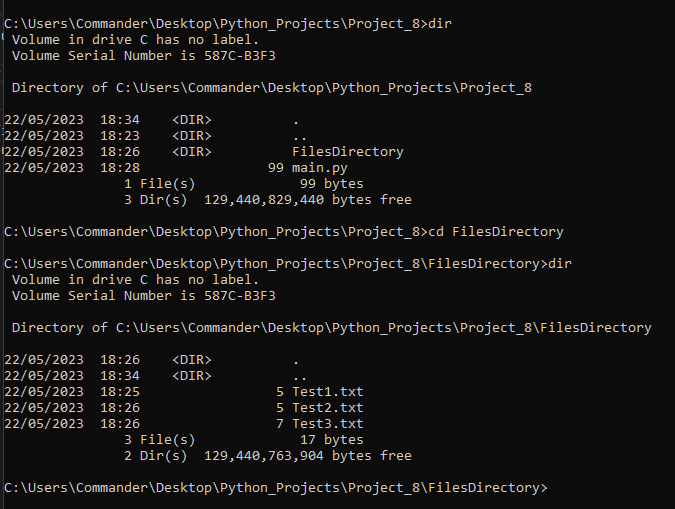
import os
# Get the current working directory
current_dir = os.getcwd()
# We create the path for the directory called "FilesDirectory"
pathDir = os.path.join(current_dir, "FilesDirectory")
# List directory contents
files = os.listdir(pathDir)
print(files)

CHECKING FILE EXISTENCE:
import os
# Get the current working directory
current_dir = os.getcwd()
# We create the path for the directory called "FilesDirectory"
pathDir = os.path.join(current_dir, "FilesDirectory")
# Check file existence
checkFile1 = os.path.join(pathDir, "Test2.txt")
checkFile2 = os.path.join(pathDir, "Test4.txt")
verifyFile1 = os.path.exists(checkFile1)
verifyFile2 = os.path.exists(checkFile2)
print(f"Does the file {checkFile1} exist? {verifyFile1}")
print(f"Does the file {checkFile2} exist? {verifyFile2}")

CREATING AND REMOVING A DIRECTORY:
import os
# Get the current working directory
current_dir = os.getcwd()
# Create a new directory called FilesTest
pathDir = os.path.join(current_dir, "FilesTest")
os.mkdir(pathDir)
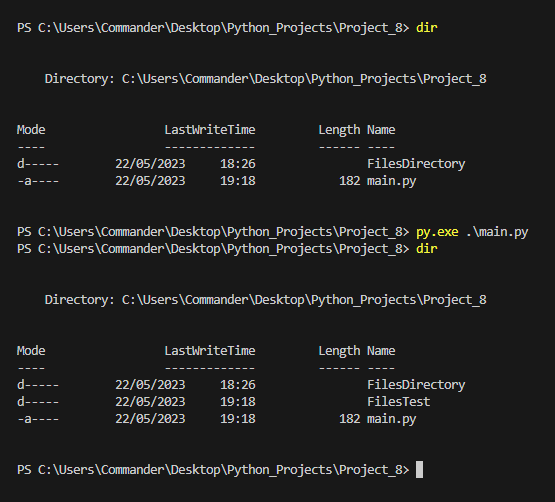
import os
# Get the current working directory
current_dir = os.getcwd()
# Remove, if exists, a directory called FilesTest
pathDir = os.path.join(current_dir, "FilesTest")
if os.path.exists(pathDir):
os.rmdir(pathDir)
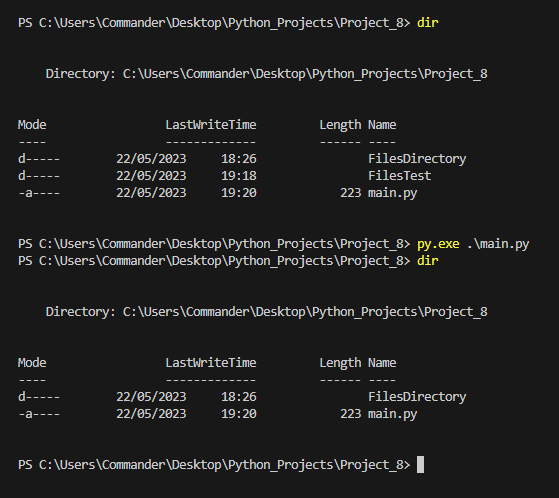
COPYING, MOVING, RENAMING AND DELETING A FILE:
import os
import shutil
# Get the current working directory
current_dir = os.getcwd()
# Get the path for the directory "FilesDirectory"
pathDir = os.path.join(current_dir, "FilesDirectory")
# Get the path for the file Test1.txt
fileSource = os.path.join(pathDir, "Test1.txt")
# We copy the file Test1.txt in the current working directory
shutil.copy(fileSource, current_dir)
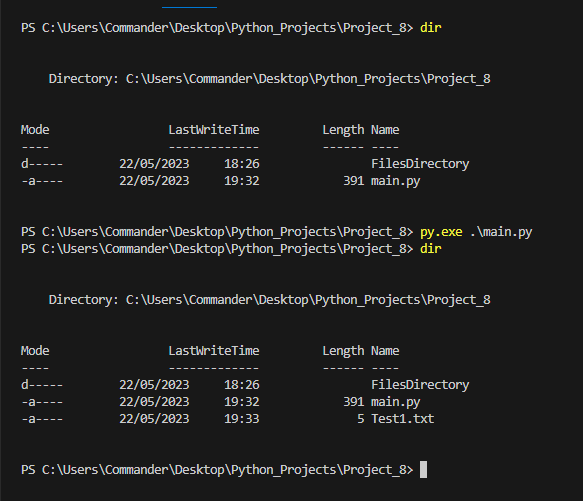
import os
import shutil
# Get the current working directory
current_dir = os.getcwd()
# Get the path for the directory "FilesDirectory"
pathDir = os.path.join(current_dir, "FilesDirectory")
# Get the path for the file Test1.txt
fileSource = os.path.join(pathDir, "Test1.txt")
# We move the file Test1.txt in the current working directory
shutil.move(fileSource, current_dir)
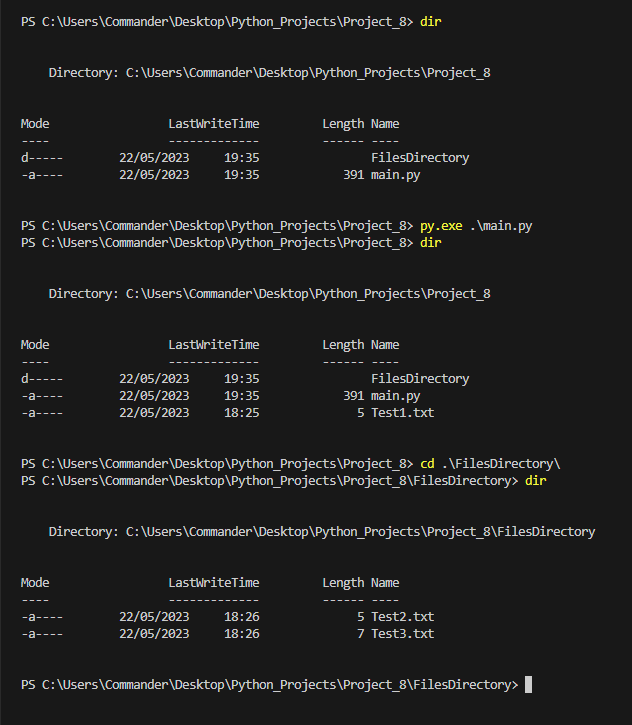
import os
# Get the current working directory
current_dir = os.getcwd()
# Get the path for the file Test1.txt
fileSource = os.path.join(current_dir, "Test1.txt")
# Get the path for the "new file"
newfile = os.path.join(current_dir, "TestDamiano.txt")
# We rename the file Test1.txt in TestDamiano.txt
os.rename(fileSource, newfile)
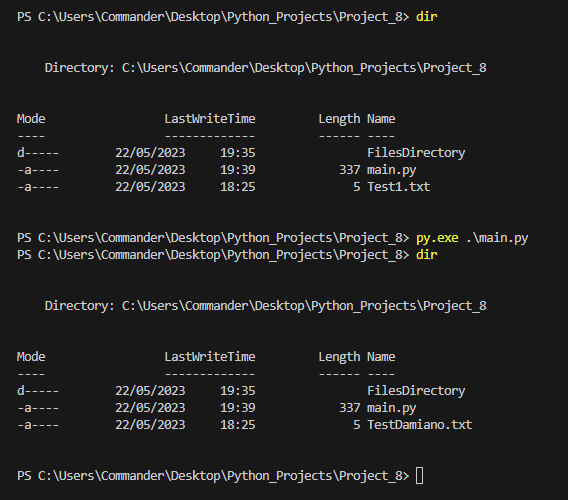
import os
# Get the current working directory
current_dir = os.getcwd()
# Get the path for the file TestDamiano.txt
newfile = os.path.join(current_dir, "TestDamiano.txt")
# Delete file
os.remove(newfile)
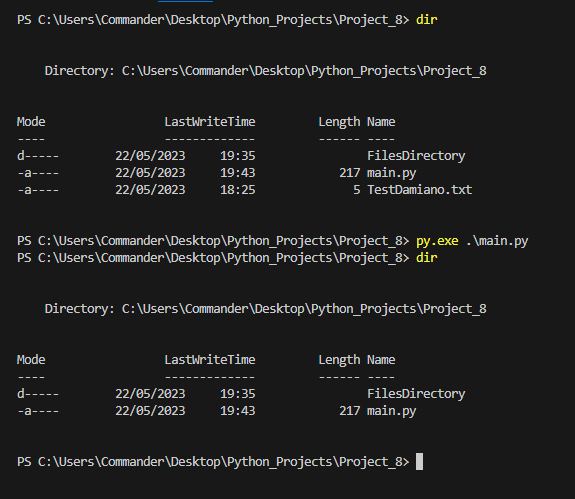