In this post, we will see how to use Interfaces in Python.
We remember that an Interface is a powerful concept in object-oriented programming that, allows us to define a common set of methods that implementing classes must provide.
It helps establish a contract between classes, ensuring that certain methods are available and must be implemented in each class that implements the interface.
By design Python doesn’t have explicit interfaces like some other programming languages but, we can achieve similar functionality using abstract base classes (ABCs).
In a nutshell, we can create interfaces using abstract base classes provided by the abc
module.
Abstract base classes are classes that cannot be instantiated and contain one or more abstract methods, which are declared but have no implementation.
Let’s see an example:
We open Visual Studio Code and we create a class called Print, so defined:
[PRINT.PY]
class Print():
def printStandard(self):
print('Method in print class')
Then, we create a file called main.py where, we will call the Print class:
[MAIN.PY]
from print import Print
objPrint = Print()
objPrint.printStandard()
If we run the main.py file, the following will be the result:
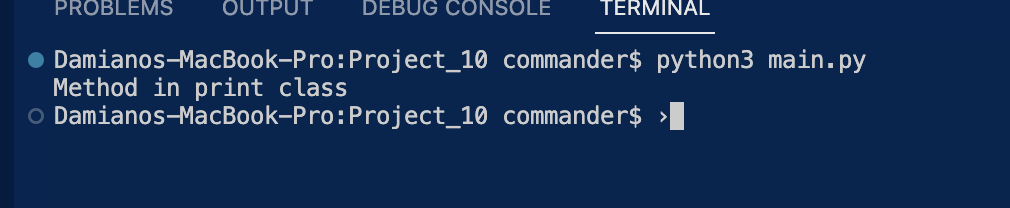
Now, we will create an Interface, called iPrint, that we will implement in the Print class:
[IPRINT.PY]
from abc import ABC, abstractmethod
class iPrint(ABC):
@abstractmethod
def printGeneric(self):
pass
@abstractmethod
def printName(self, name):
pass
Then, we will use it in Print class:
from iPrint import iPrint
class Print(iPrint):
def printStandard(self):
print('Method in print class')
If we run the main.py file, without implement the two abstract methods, we will receive an error:
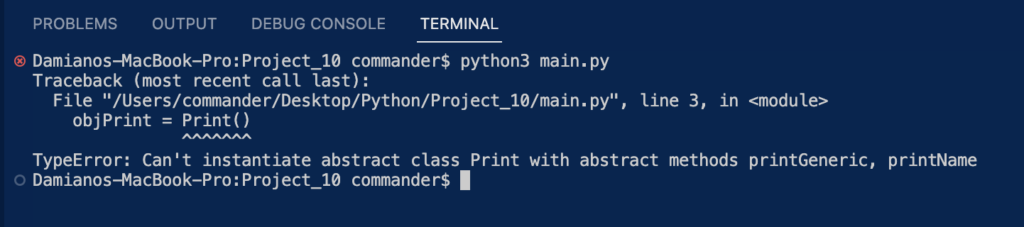
Obviously, to fix this error, we simply need to implement the methods in the Print class:
[PRINT.PY]
from iPrint import iPrint
class Print(iPrint):
def printStandard(self):
print('Method in print class')
def printGeneric(self):
print('Method in iPrint')
def printName(self, name):
print(f'Method in iPrint with this parameter in input: {name}')
Finally, we have to modify main.py:
[MAIN.PY]
from print import Print
objPrint = Print()
objPrint.printStandard()
objPrint.printGeneric()
objPrint.printName('Damiano')
We have done and now, If we run the main.py file, the following will be the result:
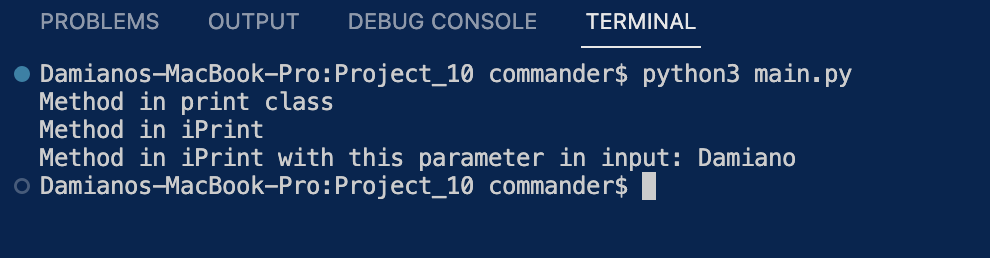