In this post, we will see how to create and manage an Enum in Pyhton.
Just to recap, an Enum is used in programming to give names to individual constant values, which can improve the readability, maintainability, and robustness of our code.
Enumerated constants are essentially named constants that belong to a specific set of values, often referred to as an “enumeration.”
In Python, we can create an Enum using the ‘Enum’ class from the ‘enum’ module.
Let’s see some examples:
[TEST.PY]
# import the module
from enum import Enum
# dewfinition of an Enum called DayOfWeek
class DayOfWeek(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
THURSDAY = 4
FRIDAY = 5
SATURDAY = 6
SUNDAY = 7
# printing the name of the item selected
print(DayOfWeek.FRIDAY.name)
# printing the value of the item selected
print(DayOfWeek.FRIDAY.value)
If we run the file, the following will be the result:

If we want to get all names and values of an Enum, we have to modify the code in this way:
[TEST.PY]
# import the module
from enum import Enum
# dewfinition of an Enum called DayOfWeek
class DayOfWeek(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
THURSDAY = 4
FRIDAY = 5
SATURDAY = 6
SUNDAY = 7
# Getting all the names and values of the enum members
for day in DayOfWeek:
print(f"{day.value} - {day.name}")
If we run the file, the following will be the result:
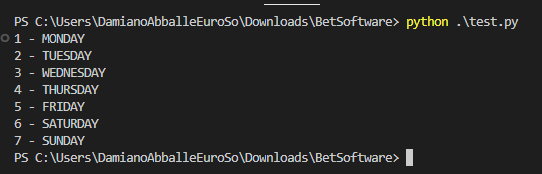
Finally, I want to show how to get the Enum name from its value:
[TEST.PY]
# import the module
from enum import Enum
# dewfinition of an Enum called DayOfWeek
class DayOfWeek(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
THURSDAY = 4
FRIDAY = 5
SATURDAY = 6
SUNDAY = 7
inputDay = input('Insert the number of the day: ')
dayName = DayOfWeek(int(inputDay))
print(f"The day inserted in input is: {dayName.name}")
If we run the file, the following will be the result:
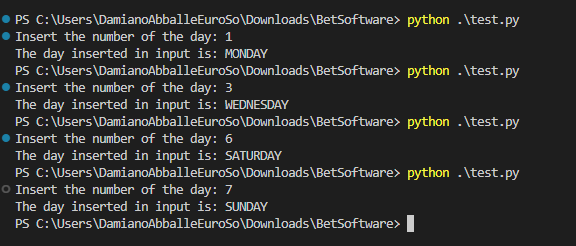