In this post, we will see how to use the Javascript library called Faker.js for generating fake data for various areas, including address, commerce, company, date, finance, image, random, or name.
Here, it is possible to find the project.
[HOW TO INSTALL]
First of all, we have to install node.js in our system, because Faker is available as a NPM package.
Then, using the command npm install faker we will install the library:
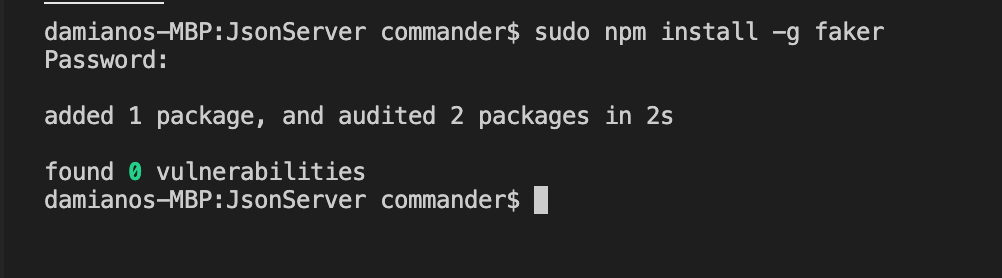
Now, using a javascript file called script.js, we will see how to use the library:
FAKE DATA FOR USER:
// we import the faker library
const faker = require('faker');
// FAKE DATA FOR USER
console.log("User 1")
console.log("First Name:" + faker.name.firstName());
console.log("Last Name:" + faker.name.lastName());
console.log("Job Title:" + faker.name.jobTitle());
console.log("\nUser 2")
console.log("First Name:" + faker.name.firstName());
console.log("Last Name:" + faker.name.lastName());
console.log("Job Title:" + faker.name.jobTitle());
console.log("\nUser 3")
console.log("First Name:" + faker.name.firstName());
console.log("Last Name:" + faker.name.lastName());
console.log("Job Title:" + faker.name.jobTitle());
If we run the script, using the command node script.js, this will be the result:

FAKE DATA FOR ADDRESS:
// we import the faker library
const faker = require('faker');
// FAKE DATA FOR ADDRESS
console.log("City 1")
console.log("City Name: " + faker.address.cityName());
console.log("Country: " + faker.address.country());
console.log("State: " + faker.address.state());
console.log("Address: " + faker.address.streetAddress());
console.log("Latitude: " + faker.address.latitude());
console.log("Longitude: " + faker.address.longitude());
console.log("\nCity 2")
console.log("City Name: " + faker.address.cityName());
console.log("Country: " + faker.address.country());
console.log("State: " + faker.address.state());
console.log("Address: " + faker.address.streetAddress());
console.log("Latitude: " + faker.address.latitude());
console.log("Longitude: " + faker.address.longitude());
console.log("\nCity 3")
console.log("City Name: " + faker.address.cityName());
console.log("Country: " + faker.address.country());
console.log("State: " + faker.address.state());
console.log("Address: " + faker.address.streetAddress());
console.log("Latitude: " + faker.address.latitude());
console.log("Longitude: " + faker.address.longitude());
If we run the script, this will be the result:
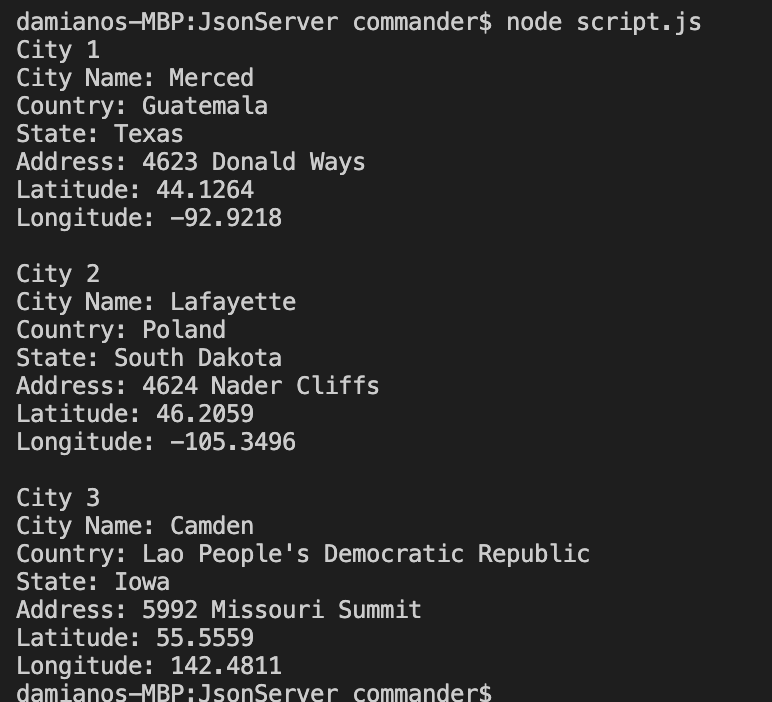
FAKE DATA FOR COMMERCE:
// we import the faker library
const faker = require('faker');
// FAKE DATA FOR COMMERCE
console.log("Product 1")
console.log("Product Name:" + faker.commerce.productName());
console.log("Product Description:" + faker.commerce.productDescription());
console.log("Product Price:" + faker.commerce.price());
console.log("\nProduct 2")
console.log("Product Name:" + faker.commerce.productName());
console.log("Product Description:" + faker.commerce.productDescription());
console.log("Product Price:" + faker.commerce.price());
console.log("\nProduct 3")
console.log("Product Name:" + faker.commerce.productName());
console.log("Product Description:" + faker.commerce.productDescription());
console.log("Product Price:" + faker.commerce.price());
If we run the script, this will be the result:
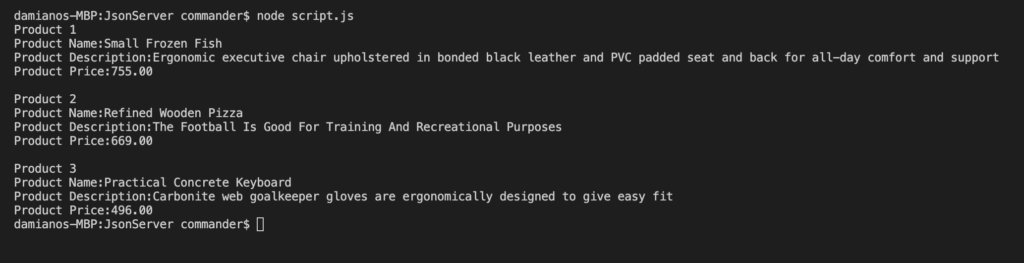
FAKE RANDOM DATA:
// we import the faker library
const faker = require('faker');
// RANDOM DATA
console.log("Random 1");
console.log("Word: " + faker.random.word());
console.log("Image: " + faker.random.image());
console.log("Boolean: " + faker.datatype.boolean());
console.log("Date: " + faker.datatype.datetime().toLocaleDateString());
console.log("Number: " + faker.datatype.number());
console.log("Json: " + faker.datatype.json());
console.log("GUID: " + faker.datatype.uuid());
console.log("\nRandom 2");
console.log("Word: " + faker.random.word());
console.log("Image: " + faker.random.image());
console.log("Boolean: " + faker.datatype.boolean());
console.log("Date: " + faker.datatype.datetime().toLocaleDateString());
console.log("Number: " + faker.datatype.number());
console.log("Json: " + faker.datatype.json());
console.log("GUID: " + faker.datatype.uuid());
console.log("\nRandom 3");
console.log("Word: " + faker.random.word());
console.log("Image: " + faker.random.image());
console.log("Boolean: " + faker.datatype.boolean());
console.log("Date: " + faker.datatype.datetime().toLocaleDateString());
console.log("Number: " + faker.datatype.number());
console.log("Json: " + faker.datatype.json());
console.log("GUID: " + faker.datatype.uuid());
If we run the script, this will be the result:
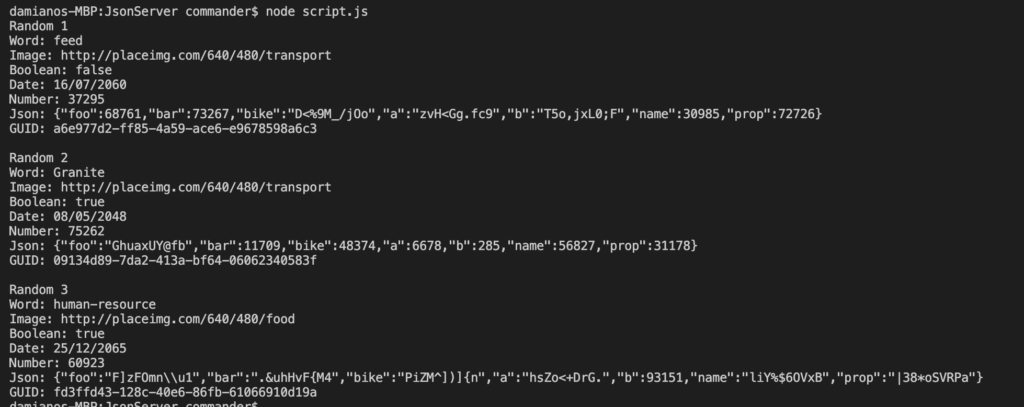
FAKE DATA FOR INTERNET :
// we import the faker library
const faker = require('faker');
// FAKE DATA FOR INTERNET
console.log("Internet 1");
console.log("Avatar: " + faker.internet.avatar());
console.log("Domain Name: " + faker.internet.domainName());
console.log("Email: " + faker.internet.email());
console.log("Ip: " + faker.internet.ip());
console.log("Url: " + faker.internet.url());
console.log("\nInternet 2");
console.log("Avatar: " + faker.internet.avatar());
console.log("Domain Name: " + faker.internet.domainName());
console.log("Email: " + faker.internet.email());
console.log("Ip: " + faker.internet.ip());
console.log("Url: " + faker.internet.url());
console.log("\nInternet 3");
console.log("Avatar: " + faker.internet.avatar());
console.log("Domain Name: " + faker.internet.domainName());
console.log("Email: " + faker.internet.email());
console.log("Ip: " + faker.internet.ip());
console.log("Url: " + faker.internet.url());
If we run the script, this will be the result:
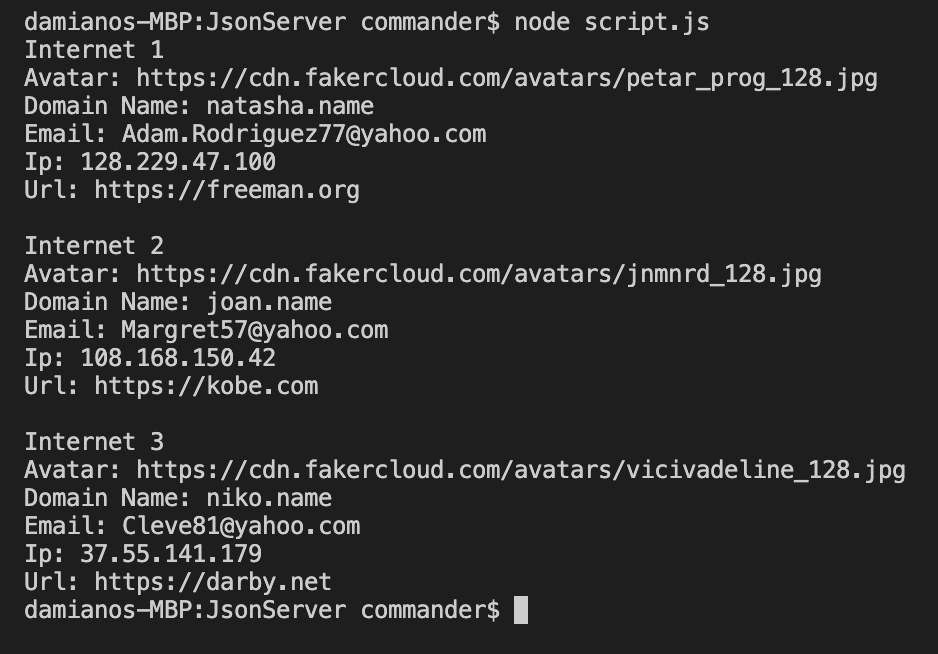
FAKE DATE:
// we import the faker library
const faker = require('faker');
// FAKE DATE
console.log(`Today is: ${(new Date).toLocaleDateString()} \n`);
// future date
console.log("FUTURE DATE");
console.log(faker.date.future().toLocaleDateString());
console.log(faker.date.future().toLocaleDateString());
console.log(faker.date.future().toLocaleDateString());
console.log(faker.date.future().toLocaleDateString());
console.log(faker.date.future().toLocaleDateString());
// past date
console.log("\nPAST DATE");
console.log(faker.date.past().toLocaleDateString());
console.log(faker.date.past().toLocaleDateString());
console.log(faker.date.past().toLocaleDateString());
console.log(faker.date.past().toLocaleDateString());
console.log(faker.date.past().toLocaleDateString());
// recent date
console.log("\nRECENT DATE");
console.log(faker.date.recent().toLocaleDateString());
console.log(faker.date.recent().toLocaleDateString());
console.log(faker.date.recent().toLocaleDateString());
console.log(faker.date.recent().toLocaleDateString());
console.log(faker.date.recent().toLocaleDateString());
If we run the script, this will be the result:
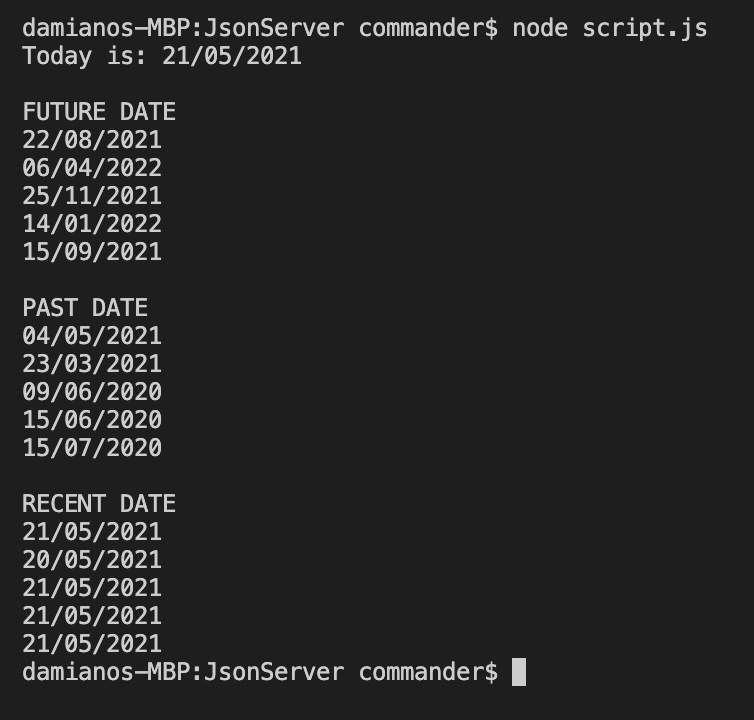
We have seen some example of Fake data and we could use this library, in order to create a json file to use with Json Server:
// we import the faker library
const faker = require('faker');
const fs = require('fs')
function generateData() {
let data = []
for (let id=1; id <= 5; id++) {
let id = faker.datatype.uuid();
let username = faker.internet.userName();
let password = faker.internet.password();
let email = faker.internet.email();
data.push({
"id": id,
"username": username,
"password": password,
"email": email
});
}
return { "users": data }
}
let dataObj = generateData();
fs.writeFileSync('data.json', JSON.stringify(dataObj, null, '\t'));
If we run the script, it will create a file json called data.json:
{
"users": [
{
"id": "48d11bfc-8d09-4d60-a6df-ca7a4bfab5fd",
"username": "Harry.Murazik",
"password": "pF5KLec8vHTJ_b0",
"email": "Cathrine48@yahoo.com"
},
{
"id": "31e4558b-74d3-4b3f-a3b8-5ee85f847799",
"username": "Winnifred46",
"password": "jtr6A_CqAMkmVxt",
"email": "Ahmed_Runte@yahoo.com"
},
{
"id": "d65d94c8-226d-469c-a81f-9e75a8e2edc2",
"username": "Dorian_Kozey9",
"password": "vrzEFamz9Ik3vzQ",
"email": "Jamaal41@gmail.com"
},
{
"id": "9a8aeabc-ac4e-4e88-afbf-c1371a5a34a3",
"username": "Abner.Weimann",
"password": "L9TWneGOLYdQCpV",
"email": "Willis_Hickle@hotmail.com"
},
{
"id": "a7183044-410a-4967-97b9-e8d4cde3ac48",
"username": "Johan_Morissette64",
"password": "C0RLwZdCx7ki_Qf",
"email": "Fanny54@hotmail.com"
}
]
}
Finally, if we run Json server (json-server –watch data.json) and we go to http://localhost:3000/users, we will see the data:

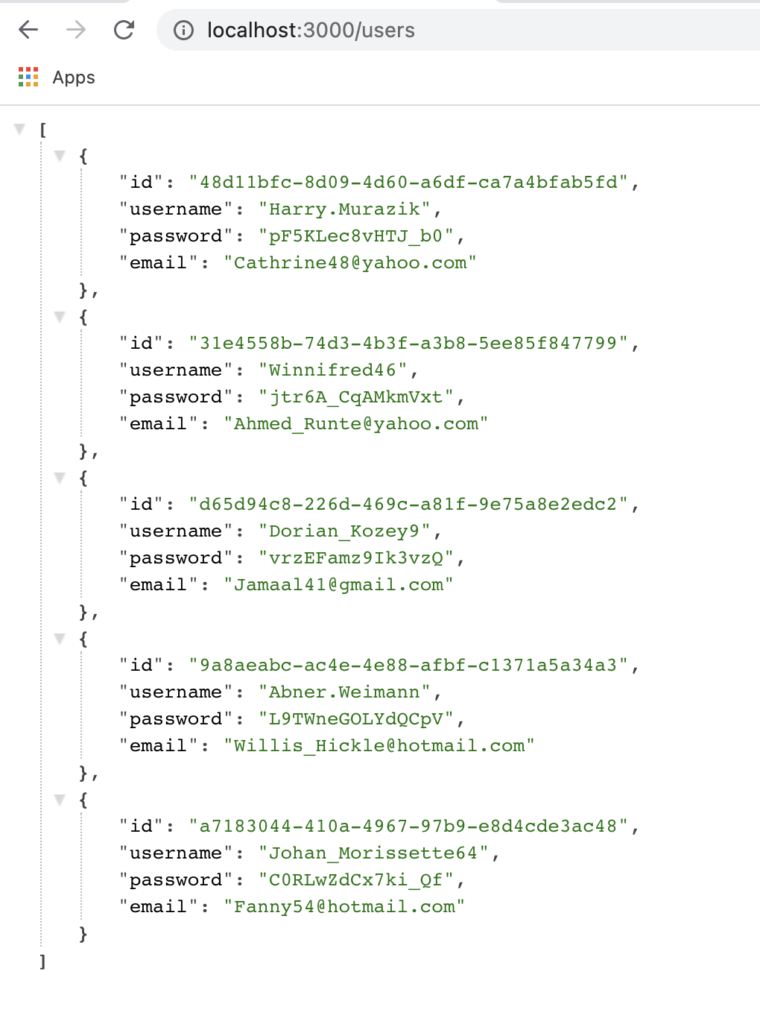