In this post, we will see how to deploy a Console Application as a single file.
This, can be advantageous when we want to simplify the distribution of our applications and make it easer for users to execute.
Obviously, the size of the single file in a self-contained application is large since it includes the runtime and the framework libraries.
For all information, we can consult the Microsoft website.
First of all, we create a Console Application called “TimeSpanCalculation”, used to calculate the time difference (timespan) between two times:
static void CheckInput()
{
// Input two times in a 24-hour format
Console.Write("Enter the first time (hh:mm:ss): ");
string input1 = Console.ReadLine();
Console.Write("Enter the second time (hh:mm:ss): ");
string input2 = Console.ReadLine();
// Validate the input strings
if (!IsValidTime(input1) || !IsValidTime(input2))
{
Console.WriteLine("Invalid time format. Please use the format hh:mm:ss.");
return;
}
// Parse the input strings into DateTime objects
DateTime time1 = DateTime.Parse(input1);
DateTime time2 = DateTime.Parse(input2);
// Check that the second time is greater than the first
if (time2 <= time1)
{
Console.WriteLine("The second time must be greater than the first time.");
return;
}
// Calculate the difference between the two times
TimeSpan difference = time2 - time1;
// Output the time difference
Console.WriteLine("The time difference is: " + difference);
}
// Helper method to validate the time format
static bool IsValidTime(string input)
{
TimeSpan time;
return TimeSpan.TryParse(input, out time);
}
CheckInput();
If we run the application, the following will be the result:
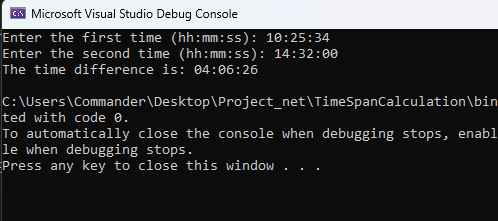
Now, if we want to release this software, we have to select ‘release’ and then, we have to Build the Solution.
The result will be a new directory called “Release” where, we will find all files for the release.

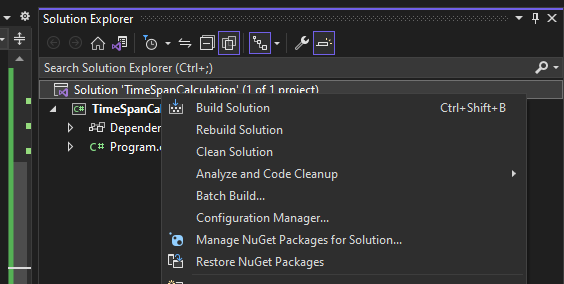
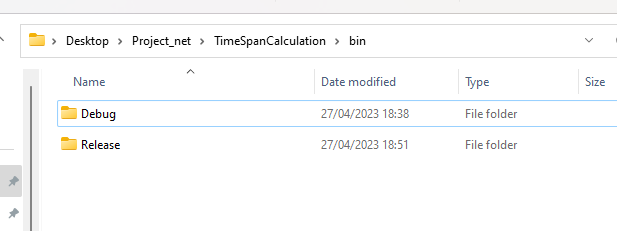
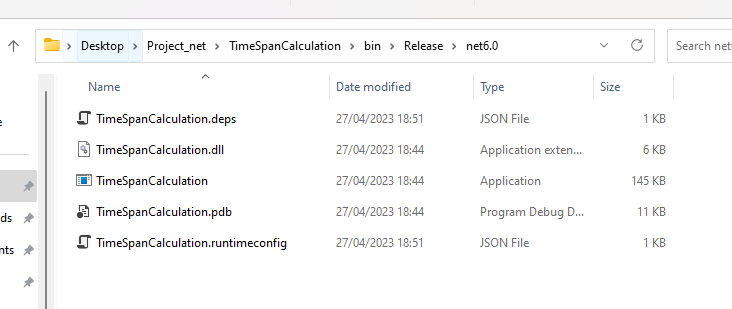
We have done and now, if we double click on TimeSpaCalculation application file, the following will be the result:
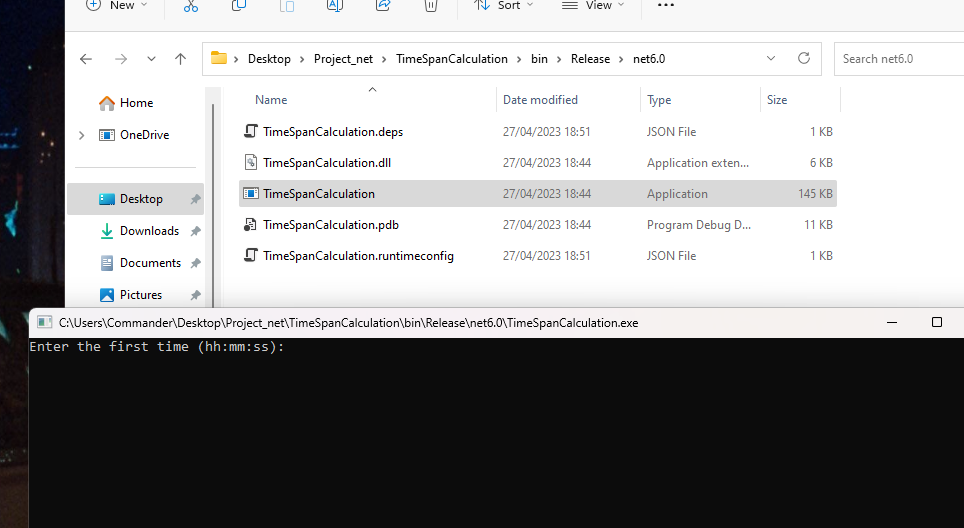
We can see that everything works fine but now, we will release the application as a single file using the “dotnet publish” command:
dotnet publish -c Release -r <runtime> --self-contained true -p:PublishSingleFile=true -o ./publish
where, in the <runtime> parameter, we can choice our desired target runtime, such as “win-x64”, “linux-x64”, or “osx-x64”.
This command will publish our console application as a single-file executable in the “./publish” folder.

If we go to the project folder, we will see a new folder called “publish” with the single file of the application:
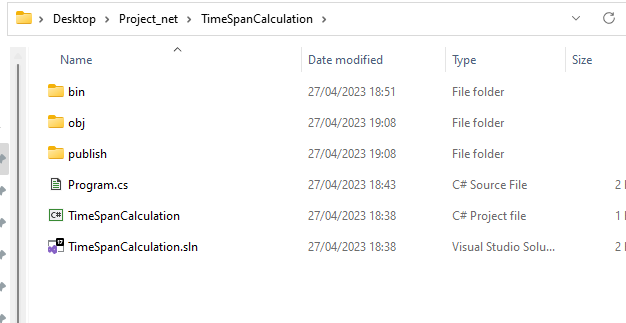
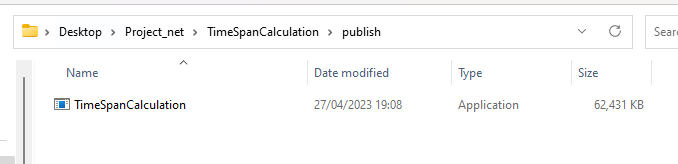
Now, if we double click on TimeSpaCalculation file, the following will be the result:
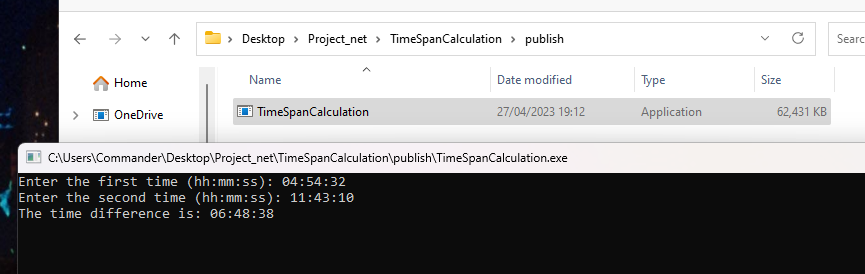
Finally, let’s see how to optimize the output of the single file by modifying only the “dotnet publish” command like this:
dotnet publish -c Release -r <runtime> --self-contained true -p:PublishSingleFile=true -p:PublishReadyToRun=true -p:PublishTrimmed=true -o ./publish
With this command, we have enabled the ReadyToRun (R2R) compilation and trim the unused code. R2R can reduce the startup time of our application by precompiling it, while trimming can reduce the file size.

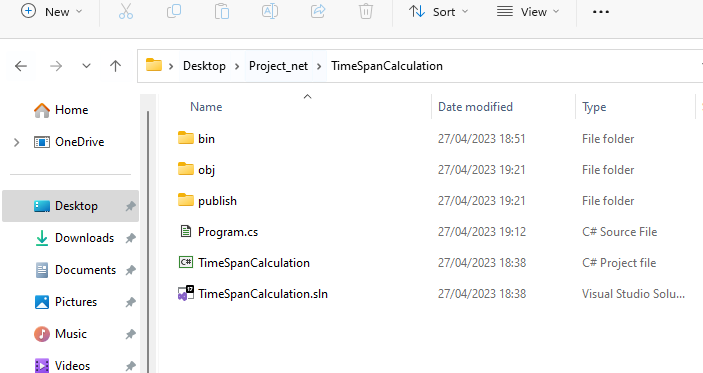
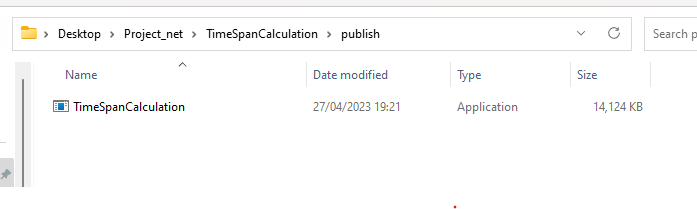
We can see that now the size of the file is only 14,124 KB while before it was 62,431 KB.
Obviously, if we double click on the TimeSpanCalculation file, the result will be the same:
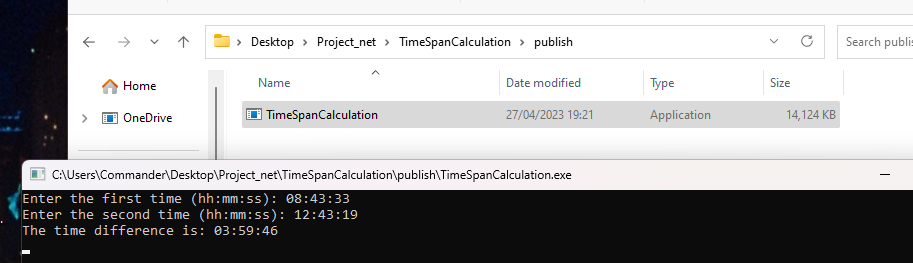