In this post, we will see how to overload methods in Python.
We remember that, Method overloading, is a feature of object-oriented programming that allows a class to have multiple methods with the same name but, with different parameters. This can make code more concise and easier to read by reducing the number of method names that need to be remembered.
In some programming languages, such as C#, method overloading is a built-in feature of the language. However, Python does not support method overloading in the traditional sense. This is because Python is a dynamically typed language, which means that the type of a variable is determined at runtime rather than at compile time.
Despite this limitation, we can still achieve method overloading in Python using default arguments or variable-length arguments.
Let’s see some examples:
DEFAULT ARGUMENTS
One way to achieve Method overloading in Python is to use default arguments.
When defining a method with default arguments, we can define a single method that can take different numbers of arguments.
Here’s an example:
[MAIN.PY]
def methodprint():
print("No parameters in Input")
methodprint()
If we run the code, the following will be the result:

Now, we overload this method using a parameter that, if it is not empty, it will be printed on the screen:
[MAIN.PY]
def methodprint(value1=None):
if value1 == None:
print("No parameters in Input")
else:
print(f"The value passed in input is:{str(value1)}")
methodprint()
methodprint("Damiano")
methodprint(3)
If we run the code, the following will be the result:

Obviously, we can have more parameters in input:
[MAIN.PY]
def methodprint(value1=None, value2=None, value3=None):
result = 'The values passed in input are: '
if value1 == None and value2 == None and value3 == None:
result = "No parameters in Input"
if value1 != None:
result = result + str(value1) + ' '
if value2 != None:
result = result + str(value2) + ' '
if value3 != None:
result = result + str(value3) + ' '
print(result)
methodprint()
methodprint("Damiano")
methodprint("Damiano", "Abballe")
methodprint("Damiano", "Abballe", "Software Architect")
If we run the code, the following will be the result:

VARIABLE-LENGTH ARGUMENTS
Another way to achieve Method overloading in Python, is to use variable-length arguments.
When defining a method with variable-length arguments, we can define a single method that can take any number of arguments.
Here’s an example:
[MAIN.PY]
def methodprint(*args):
result = 'The values passed in input are: '
if len(args) == 0:
result = "No parameters in Input"
if len(args) == 1:
result = result + str(args[0]) + ' '
if len(args) == 2:
result = result + str(args[0]) + ' ' + str(args[1]) + ' '
if len(args) == 3:
result = result + str(args[0]) + ' ' + \
str(args[1]) + ' ' + str(args[2]) + ' '
print(result)
methodprint()
methodprint("Damiano")
methodprint("Damiano", "Abballe")
methodprint("Damiano", "Abballe", "Software Architect")
If we run the code, the following will be the result:

Before concluding this post, I want to highlight that these two ways to overload a method can be applied for a constructor method of a class as well.
Let’s see an example:
[MAIN.PY] -> DEFAULT ARGUMENTS
class Person():
def __init__(self, name=None, surname=None, job=None):
self.Name = name
self.Surname = surname
self.Job = job
def PrintMethod(self):
result = 'The values passed in input are: '
if self.Name == None and self.Surname == None and self.Job == None:
result = "No parameters in Input"
if self.Name != None:
result = result + str(self.Name) + ' '
if self.Surname != None:
result = result + str(self.Surname) + ' '
if self.Job != None:
result = result + str(self.Job) + ' '
print(result)
objPerson = Person()
objPerson2 = Person("Damiano")
objPerson3 = Person("Damiano", "Abballe")
objPerson4 = Person("Damiano", "Abballe", "Software Architect")
objPerson.PrintMethod()
objPerson2.PrintMethod()
objPerson3.PrintMethod()
objPerson4.PrintMethod()
If we run the code, the following will be the result:
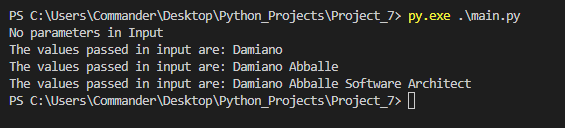
[MAIN.PY] -> VARIABLE-LENGTH ARGUMENTS
class Person():
def __init__(self, *args):
self.Name = None
self.Surname = None
self.Job = None
if len(args) == 1:
self.Name = args[0]
if len(args) == 2:
self.Name = args[0]
self.Surname = args[1]
if len(args) == 3:
self.Name = args[0]
self.Surname = args[1]
self.Job = args[2]
def PrintMethod(self):
result = 'The values passed in input are: '
if self.Name == None and self.Surname == None and self.Job == None:
result = "No parameters in Input"
if self.Name != None:
result = result + str(self.Name) + ' '
if self.Surname != None:
result = result + str(self.Surname) + ' '
if self.Job != None:
result = result + str(self.Job) + ' '
print(result)
objPerson = Person()
objPerson2 = Person("Damiano")
objPerson3 = Person("Damiano", "Abballe")
objPerson4 = Person("Damiano", "Abballe", "Software Architect")
objPerson.PrintMethod()
objPerson2.PrintMethod()
objPerson3.PrintMethod()
objPerson4.PrintMethod()
If we run the code, the following will be the result:
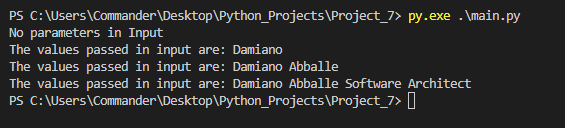