Extension methods enable you to “add” methods at one existing type without creating a new derived type, compiling, or otherwise modifying the original type.
An extension method is a static method that must been define in a static class with at least one parameter. The first parameter specifies the type on which the extension method will operate on and it must be preceded by the keyword “this”.
Some examples:
The first extension method uses the “Int32” type to calculate the square of a number.
public static class Utility
{
public static int SquareNumber(this Int32 valueInput)
{
return valueInput * valueInput;
}
}
class Program
{
static void Main(string[] args)
{
Console.Write("Insert an int number: ");
int valInput = Convert.ToInt32(Console.ReadLine());
Console.WriteLine($"The square is: {valInput.SquareNumber()}");
}
}
If we run the program, this will be the result:

The second extension method uses the “Int32” type to multiply a number with another.
public static class Utility
{
public static int Multiply(this Int32 valueInput, Int32 numberToMultiply)
{
return valueInput * numberToMultiply;
}
}
class Program
{
static void Main(string[] args)
{
Console.Write("Insert an int number: ");
int valInput = Convert.ToInt32(Console.ReadLine());
Console.Write("Insert the second int number: ");
int valInput2 = Convert.ToInt32(Console.ReadLine());
Console.WriteLine($"The result is: {valInput.Multiply(valInput2)}");
}
}
If we run the program, this will be the result:
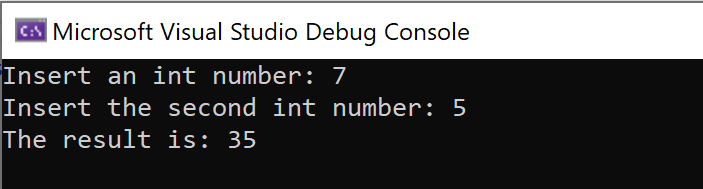
The last extension method uses the “int[]” type to give in output an array of only even numbers.
public static class Utility
{
public static int[] TakeEven(this int[] arrayInput)
{
List<int> lstEven = new List<int>();
foreach (int i in arrayInput)
{
if(i%2==0)
{
lstEven.Add(i);
}
}
return lstEven.ToArray();
}
}
class Program
{
static void Main(string[] args)
{
int[] lstTest = new int[] { 1, 2, 3, 4, 5, 6, 7 };
int[] lstEven = lstTest.TakeEven();
StringBuilder showLstEven = new StringBuilder();
Console.WriteLine("Array in input: [1 2 3 4 5 6 7]");
foreach(int i in lstEven)
{
showLstEven.Append($"{i} ");
}
Console.WriteLine($"Output array of only even numbers: [{showLstEven.ToString().Trim()}]");
}
}
If we run the program, this will be the result:
